Java ArrayList.retainAll() Method
public boolean retainAll(Collection<?> c)
The retainAll() method is used to remove it's elements from a list that are not contained in the specified collection.
Package: java.util
Java Platform: Java SE 8
Syntax:
retainAll(Collection<?> c)
Parameters:
Name | Description |
---|---|
c | collection containing elements to be retained in this list |
Return Value:
true if this list changed as a result of the call
Throws:
- ClassCastException - if the class of an element of this list is incompatible with the specified collection (optional)
- NullPointerException - if this list contains a null element and the specified collection does not permit null elements (optional), or if the specified collection is null
Pictorial presentation of ArrayList.retainAll() Method
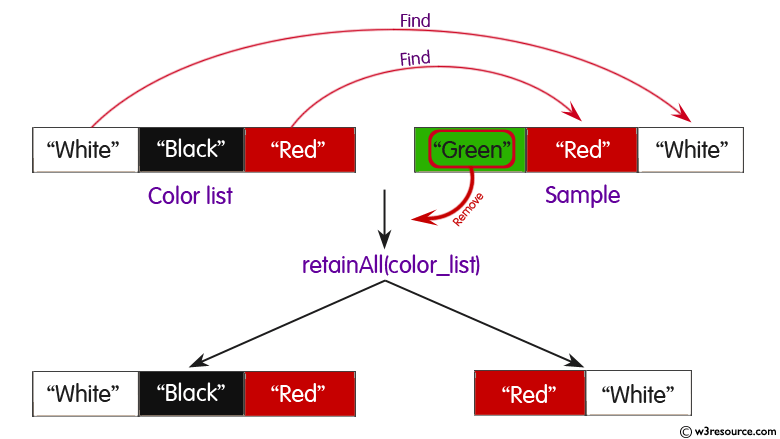
Example: Java ArrayList.retainAll() Method
The following example the retainAll() method is used to remove it's elements from a list that are not contained in the specified collection.
import java.util.*;
public class test {
public static void main(String[] args) {
// create an empty array list
ArrayList<String> color_list = new ArrayList<String>();
// use add() method to add values in the list
color_list.add("White");
color_list.add("Black");
color_list.add("Red");
// create an empty array sample with an initial capacity
ArrayList<String> sample = new ArrayList<String>();
// use add() method to add values in the list
sample.add("Green");
sample.add("Red");
sample.add("White");
System.out.println("First List :"+ color_list);
System.out.println("Second List :"+ sample);
sample.retainAll(color_list);
System.out.println("After applying the method, First List :"+ color_list);
System.out.println("After applying the method, Second List :"+ sample);
}
}
Output:
F:\java>javac test.java F:\java>java test First List :[White, Black, Red] Second List :[Green, Red, White] After applying the method, First List :[White, Black, Red] After applying the method, Second List :[Red, White]
Previous:removeAll Method
Next:listIterator(index) Method