Java ArrayList.lastIndexOf() Method
public int lastIndexOf(Object o)
The lastIndexOf() method is used to get the index of the last occurrence of an element in an ArrayList object.
Package : java.util
Java Platform : Java SE 8
Syntax:
lastIndexOf(Object o)
Parameters:
Name | Description |
---|---|
o | The element whose index is to be returned. |
Return Value:
The index of the last occurrence of an element in an ArrayList object, or -1 if the element does not exist.
Return Value Type: int
Pictorial presentation of ArrayList.lastIndexOf() Method
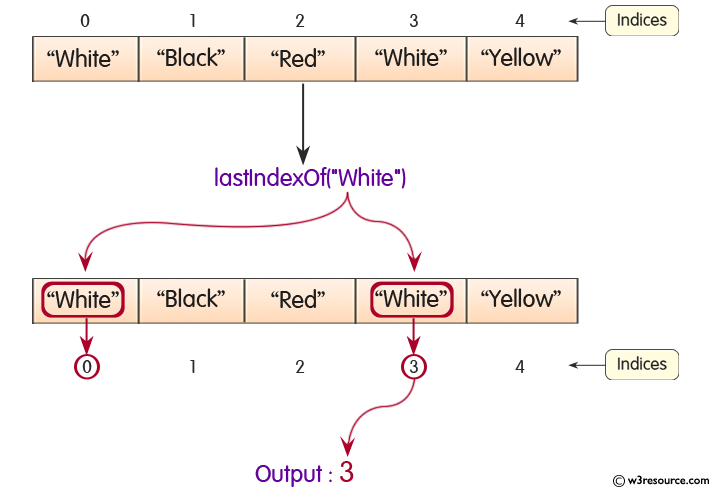
Example: ArrayList.lastIndexOf Method
The following example creates an ArrayList containing various colors. It then tries to determine the last position of a certain color.
import java.util.*;
public class test {
public static void main(String[] args) {
// create an empty array list with an initial capacity
ArrayList<String> color_list = new ArrayList<String>(5);
// use add() method to add values in the list
color_list.add("White");
color_list.add("Black");
color_list.add("Red");
color_list.add("White");
color_list.add("Yellow");
System.out.println("Size of list: " + color_list.size());
// Print the colors in the list
for (String value : color_list) {
System.out.println("Value = " + value);
}
// Get the last occurrence of "White" color in color_list
int pos=color_list.lastIndexOf("White");
System.out.println("The last occurrence of White color is at :" + pos);
// Get the last occurrence of "Red" color in color_list
pos=color_list.lastIndexOf("Red");
System.out.println("The last occurrence of Red color is at :" + pos);
}
}
Output:
F:\java>javac test.java F:\java>java test Size of list: 5 Value = White Value = Black Value = Red Value = White Value = Yellow The last occurrence of White color is at :3 The last occurrence of Red color is at :2
Java Code Editor:
Previous:indexOf Method
Next:clone Method
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics