Java ArrayList.get() Method
public E get(int index)
The ArrayList.get() method is used to get the element of a specified position within the list.
Package: java.util
Java Platform: Java SE 8
Syntax:
get(int index)
Parameters:
Name | Description | Type |
---|---|---|
index | index of the element to return | int |
Return Value:
The element at the specified position in this list
Throws:
IndexOutOfBoundsException - if the index is out of range (index < 0 || index >= size())
Pictorial presentation of ArrayList.get() Method
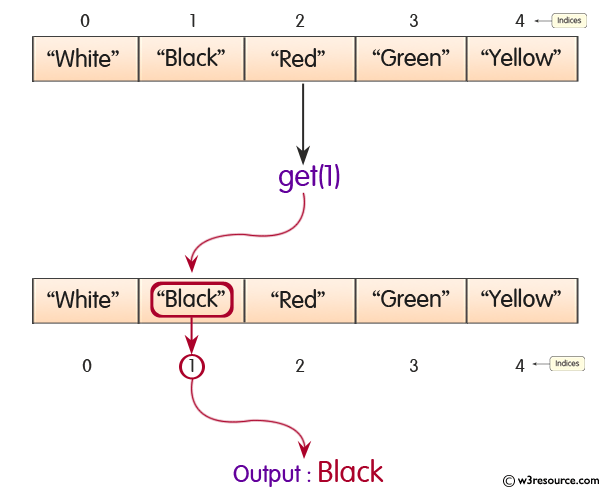
Example: ArrayList.get Method
The following example shows the usage of java.util.Arraylist.get() method method.
import java.util.*;
public class test {
public static void main(String[] args) {
// create an empty array list with an initial capacity
ArrayList<String> color_list = new ArrayList<String>(5);
// use add() method to add values in the list
color_list.add("White");
color_list.add("Black");
color_list.add("Red");
color_list.add("White");
color_list.add("Yellow");
// Print out the colors in the ArrayList.
for (int i = 0; i < 5; i++)
{
System.out.println(color_list.get(i).toString());
}
}
}
Output:
F:\java>javac test.java F:\java>java test White Black Red White Yellow
Example of Throws: get() Method
IndexOutOfBoundsException - if the index is out of range (index < 0 || index >= size()).
Let
int i = 0; i < 6; i++
in the above example.
Output:
White Black Red White Yellow Exception in thread "main" java.lang.IndexOutOfBoundsEx ception: Index: 5, Size: 5 at java.util.ArrayList.rangeCheck(ArrayList.jav a:635) at java.util.ArrayList.get(ArrayList.java:411) at test.main(test.java:19)
Java Code Editor:
Previous:toArray(T[] a) Method
Next:set Method
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics