Java ArrayList.toArray() Method
public Object[] toArray()
The toArray() method is used to get an array which contains all the elements in ArrayList object in proper sequence (from first to last element).
Package: java.util
Java Platform: Java SE 8
Syntax:
Object[] toArray()
Return Value:
An array containing the elements of an ArrayList object in proper sequence
Pictorial presentation of ArrayList.toArray() Method
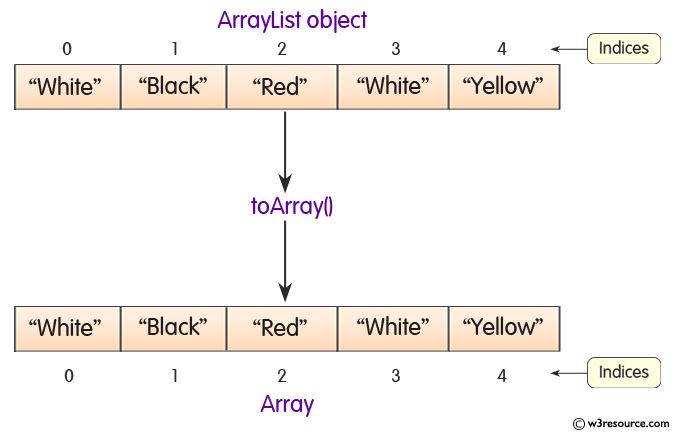
Example : ArrayList.toArray Method
The following example converts an ArrayList into an array and displays the contents of the array.
import java.util.*;
public class test {
public static void main(String[] args) {
// create an empty array list with an initial capacity
ArrayList<String> color_list = new ArrayList<String>(5);
// use add() method to add values in the list
color_list.add("White");
color_list.add("Black");
color_list.add("Red");
color_list.add("White");
color_list.add("Yellow");
System.out.println("Size of list: " + color_list.size());
// Print the colors in the list
for (String value : color_list) {
System.out.println("Color = " + value);
}
// Create an array from the ArrayList
Object[] obj = color_list.toArray();
// Display the contents of the array
System.out.println("Printing elements from first to last:");
for (Object value : obj) {
System.out.println("Color = " + value);
}
}
}
Output:
Sample Output:
F:\java>javac test.java F:\java>java test Size of list: 5 Color = White Color = Black Color = Red Color = White Color = Yellow Printing elements from first to last: Color = White Color = Black Color = Red Color = White Color = Yellow
Java Code Editor:
Previous:clone Method
Next:toArray(T[] a) Method