Java ArrayList.clear() Method
public void clear()
The clear() method is used to remove all of the elements from a list. The list will be empty after this call returns.
Package: java.util
Java Platform : Java SE 8
Syntax:
clear()
Return Value:
This method does not return any value.
Pictorial presentation of ArrayList.clear() Method
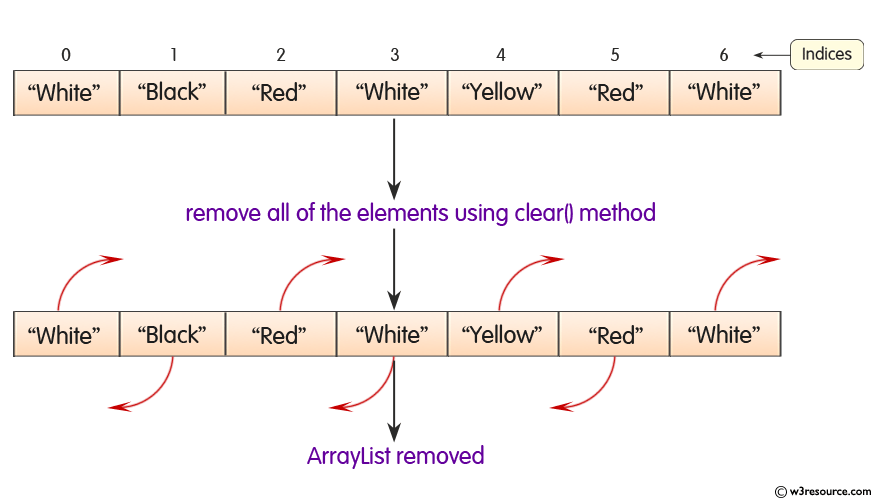
Example: ArrayList.clear() Method
The following example creates an ArrayList with a capacity of 7 elements. After adding some elements we have remove all the contents using clear(0 method.
import java.util.*;
public class test {
public static void main(String[] args) {
// create an empty array list with an initial capacity
ArrayList<String> color_list = new ArrayList<String>(7);
// use add() method to add values in the list
color_list.add("White");
color_list.add("Black");
color_list.add("Red");
color_list.add("White");
color_list.add("Yellow");
color_list.add("Red");
color_list.add("White");
// Print out the colors in the ArrayList
System.out.println("****Color list****");
for (int i = 0; i < 7; i++)
{
System.out.println(color_list.get(i).toString());
}
// Remove all the elements using clear()
color_list.clear();
//Now size of the list
System.out.println("After using clear() method, the size of the list is: " + color_list.size());
}
}
Output:
F:\java>javac test.java F:\java>java test ****Color list**** White Black Red White Yellow Red White After using clear() method, the size of the list is: 0
Java Code Editor:
Previous:remove(Object o) Method
Next:addAll(Collection c) Method