Java ArrayList.listIterator() Method
public ListIterator<E> listIterator()
The listIterator() method is used to get a list iterator over the elements in this list (in proper sequence).
Package: java.util
Java Platform: Java SE 8
Syntax:
listIterator()
Return Value:
A list iterator over the elements in this list (in proper sequence)
Pictorial presentation of ArrayList.listIterator() Method
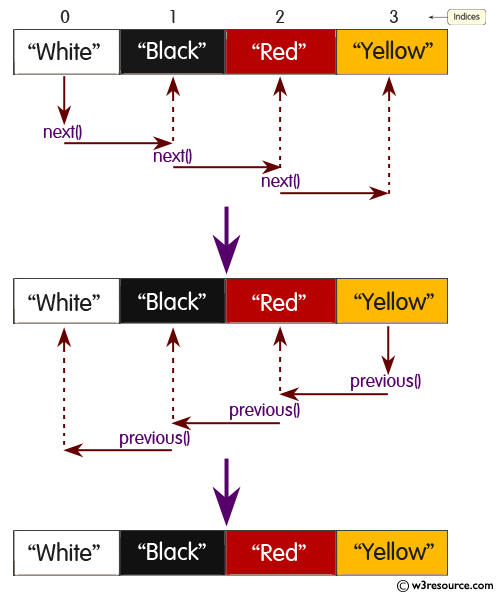
Example: Java ArrayList.listIterator Method
The following example creates an ArrayList with a capacity of 50 elements. Four elements are then added to the ArrayList and the ArrayList is trimmed accordingly.
import java.util.*;
public class test {
public static void main(String[] args) {
// create an empty array list
ArrayList<String> color_list = new ArrayList<String>();
// use add() method to add values in the list
color_list.add("White");
color_list.add("Black");
color_list.add("Red");
System.out.println("List of the colors :" + color_list);
// using listIterator() method get a ListIterator object
ListIterator itrr = color_list.listIterator();
//Use hasNext() and next() methods to iterate through the elements in forward direction.
System.out.println("Iterating in forward direction");
while(itrr.hasNext())
System.out.println(itrr.next());
// Use hasPrevious() and previous() methods to iterate through the elements in backward direction.
System.out.println("Iterating in backward direction");
while(itrr.hasPrevious())
System.out.println(itrr.previous());
}
}
Output:
F:\java>javac test.java F:\java>java test List of the colors :[White, Black, Red] Iterating in forward direction White Black Red Iterating in backward direction Red Black White
Java Code Editor:
Previous:listIterator(index) Method
Next:iterator Method