Java ArrayList.iterator() Method
public Iterator<E> iterator()
This method returns an iterator over the elements in this list in proper sequence.
Package:java.util
Java Platform: Java SE 8
Syntax:
iterator()
Return Value:
An iterator over the elements in this list in proper sequence.
Pictorial presentation of ArrayList.iterator() Method
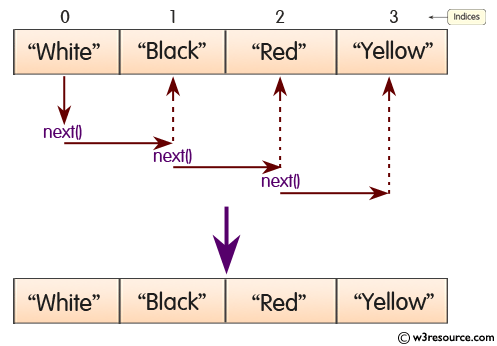
Example: Java ArrayList.iterator() Method
The following example returns an iterator over the elements in this list.
import java.util.*;
public class test {
public static void main(String[] args)
{
// create an empty array list with an initial capacity
ArrayList<String> color_list = new ArrayList<String>(7);
Iterator<String> color_Iter;
// use add() method to add values in the list
color_list.add("White");
color_list.add("Black");
color_list.add("Red");
color_list.add("White");
color_list.add("Yellow");
color_list.add("Red");
color_list.add("White");
color_Iter = color_list.iterator();
System.out.println("Elements in Color list are : ");
while(color_Iter.hasNext()){
System.out.println(color_Iter.next());
}
}
}
Output:
F:\java>javac test.java F:\java>java test Elements in Color list are : White Black Red White Yellow Red White
Java Code Editor:
Previous:listIterator() Method
Next:subList Method