Java ArrayList.indexOf() Method
public int indexOf(Object o)
The indexOf() method is used to get the index of the first occurrence of an element in an ArrayList object.
Package: java.util
Java Platform: Java SE 8
Syntax:
indexOf(Object o)
Parameters:
Name | Description |
---|---|
o | The element whose index is to be returned. |
Return Value:
The index of the first occurrence an element in ArrayList, or -1 if the element does not exist.
Return Value Type: int
Pictorial presentation of ArrayList.indexOf() Method
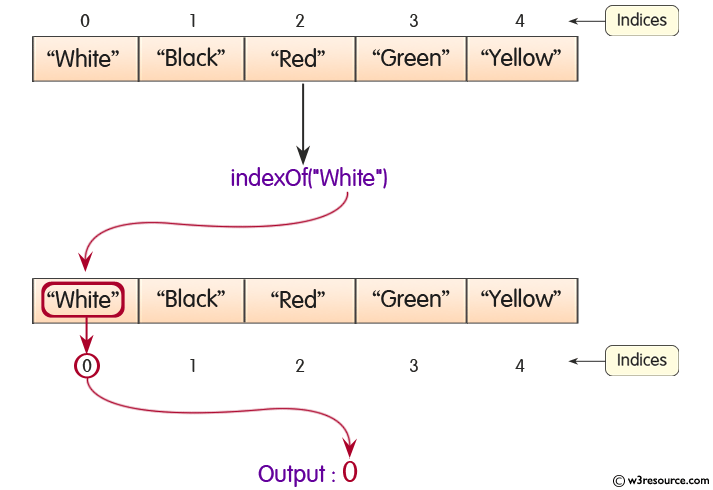
Example: ArrayList.indexOf Method
The following example creates an ArrayList containing various colors. It then tries to determine the position of a certain color.
import java.util.*;
public class test {
public static void main(String[] args) {
// create an empty array list with an initial capacity
ArrayList<String> color_list = new ArrayList<String>(5);
// use add() method to add values in the list
color_list.add("White");
color_list.add("Black");
color_list.add("Red");
color_list.add("Green");
color_list.add("Yellow");
System.out.println("Size of list: " + color_list.size());
// Print the colors in the list
for (String value : color_list) {
System.out.println("Value = " + value);
}
// Get the index of element "White"
int pos=color_list.indexOf("White");
System.out.println("The color White is at index " + pos);
// Get the index of element "Green"
pos=color_list.indexOf("Green");
System.out.println("The color Green is at index " + pos);
}
}
Output :
F:\java>javac test.java F:\java>java test Size of list: 5 Value = White Value = Black Value = Red Value = Green Value = Yellow The color White is at index 0 The color Green is at index 3
Java Code Editor:
Previous:contains Method
Next:lastIndexOf Method