NumPy: numpy.char.multiply() function
numpy.char.multiply() function
numpy.char.multiply() is a function is used to repeat each element of a string array (a) a specified number of times. Return (a * i), that is string multiple concatenation, element-wise. Values in i of less than 0 are treated as 0 (which yields an empty string). The resulting array has the same shape as the input array.
The applications of numpy.char.multiply() can include:
1.
Generating repeated patterns or sequences of characters in text data, which can be useful for creating visually distinct separators or markers in text outputs.
2. Formatting and aligning text data by repeating specific characters like spaces, tabs, or other symbols to achieve a desired layout or appearance.
Syntax:
numpy.char.multiply(a, i)
Parameters:
Name | Description | Required / Optional |
---|---|---|
a: array_like of str or unicode | Input array. | Required |
i: array_like of ints | Input array | Required |
Return value:
out : ndarray - Output array of str or unicode, depending on input types
Example: Repeating elements in string arrays using NumPy's char.multiply()
import numpy as np
a1 = ['aaa', 'bbb', 'ccc']
a2 = ['ppp', 'qqq', 'rrr']
print("\na1 : ", a1)
print("\na2 : ", a2)
print("\na1 : ", np.char.multiply(a1, 2))
print ("\na1 : ", np.char.multiply(a1, [2, 4, 3]))
print ("\na2 : ", np.char.multiply(a2, 3))
Output:
a1 : ['aaa', 'bbb', 'ccc'] a2 : ['ppp', 'qqq', 'rrr'] a1 : ['aaaaaa' 'bbbbbb' 'cccccc'] a1 : ['aaaaaa' 'bbbbbbbbbbbb' 'ccccccccc'] a2 : ['ppppppppp' 'qqqqqqqqq' 'rrrrrrrrr']
In the above code:
- The numpy.char.multiply() function is called with a1 and the integer 2 as arguments. This repeats each element of a1 two times.
- The numpy.char.multiply() function is called with a1 and a list of integers [2, 4, 3] as arguments. This repeats each element of a1 element-wise with the corresponding integer in the list.
- The numpy.char.multiply() function is called with a2 and the integer 3 as arguments. This repeats each element of a2 three times.
Pictorial Presentation:
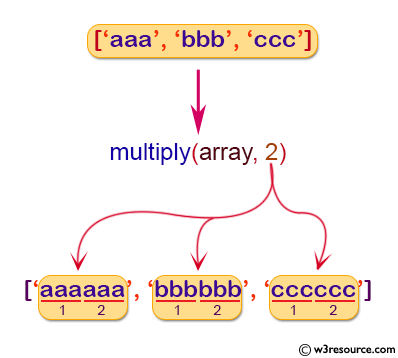
Pictorial Presentation:
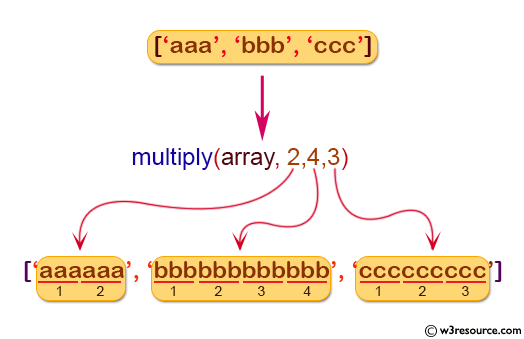
Pictorial Presentation:
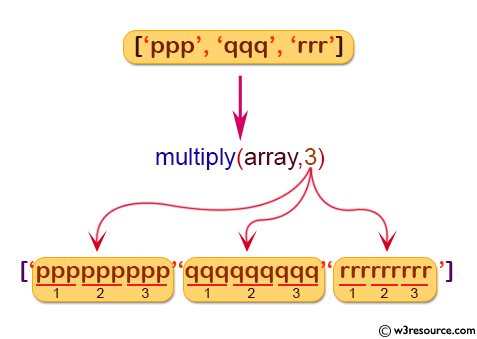
Example: Repeating strings using NumPy's char.multiply()
import numpy as np
arr1 = 'Python'
arr2 = 'NumPy Tutorial '
print ("\narr1 : ", np.char.multiply(arr1, 2))
print ("\narr2 : ", np.char.multiply(arr2, 4))
Output:
arr1 : PythonPython arr2 : NumPy Tutorial NumPy Tutorial NumPy Tutorial NumPy Tutorial
In the above code-
- Two strings, arr1 and arr2, are created.
- The numpy.char.multiply() function is called with arr1 and the integer 2 as arguments. This repeats the arr1 string two times.
- Again the numpy.char.multiply() function is called with arr2 and the integer 4 as arguments. This repeats the arr2 string four times.
Python - NumPy Code Editor:
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/numpy/string-operations/multiply.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics