NumPy String: numpy.char.center() function
numpy.char.center() function
The numpy.char.center() function create a copy of a given array with its elements centered in a string of length width.
This function is useful to improve the appearance and readability of text data, such as when creating tables, reports, or other structured text outputs. By center-aligning the elements in a string array, you can create a consistent layout across rows and columns.
Syntax:
numpy.char.center(a, width, fillchar=' ')
Parameters:
Name | Description | Required / Optional |
---|---|---|
a: array_like of str or unicode | Required | |
width: int | The length of the resulting strings. | Required |
fillchar: str or unicode | The padding character to use (default is space). | Optional |
Return value:
out : ndarray - Output array of str or unicode, depending on input types
Example-1: numpy.char.center() function
>>> import numpy as np
>>> a = np.char.center('w3resource', 16, fillchar = '#')
>>> a
array('###w3resource###', dtype='<U16')
In the above code the numpy.char.center() function is called with the input string 'w3resource', a width parameter of 16, and a fill character of '#'. This function centers the input string within a width of 16 characters by adding padding characters ('#') to the left and right of the string. The resulting string has a length of 16 characters, as specified by the width parameter.
The function returns a 0-dimensional array (scalar) containing the centered and padded string '###w3resource###', with a data type of '<U16', which represents a Unicode string with a maximum length of 16 characters.
Pictorial Presentation:
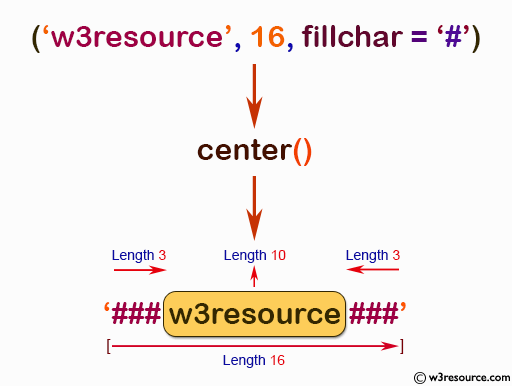
Example: Centering a string with padding using NumPy's char.center() and custom fill character
>>> import numpy as np
>>> a = np.char.center('w3resource', 30, fillchar = '.')
>>> a
array('..........w3resource..........', dtype='<U30')
In the above code the numpy.char.center() function is called with the input string 'w3resource', a width parameter of 30, and a fill character of '.'. This function centers the input string within a width of 30 characters by adding padding characters ('.') to the left and right of the string.
The function returns a 0-dimensional array (scalar) containing the centered and padded string '..........w3resource..........', with a data type of '<U30', which represents a Unicode string with a maximum length of 30 characters.
Pictorial Presentation:
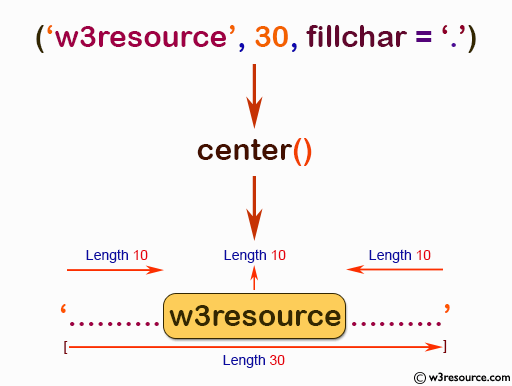
Example: Centering a String with Uneven Padding using NumPy's char.center()
>>> import numpy as np
>>> a = np.char.center('w3resource', 15, fillchar = '#')
>>> a
array('###w3resource##', dtype='<U15')
In the above code the numpy.char.center() function is called with the input string 'w3resource', a width parameter of 15, and a fill character of '#'. This function centers the input string within a width of 15 characters by adding padding characters ('#') to the left and right of the string. Since the string length is 10, the padding is uneven with 2 extra characters on the right side.
Pictorial Presentation:
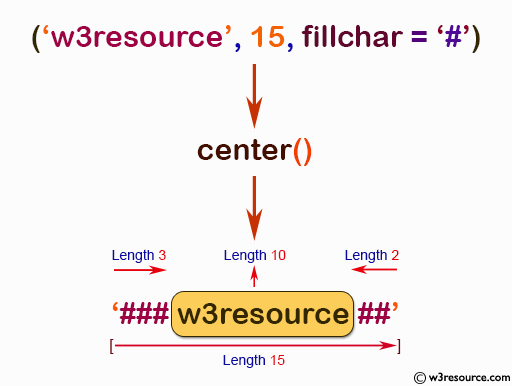
Python - NumPy Code Editor:
Previous:
capitalize()
Next:
decode()