NumPy String: numpy.char.isupper() function
numpy.char.isupper() function
The numpy.char.isupper() function is used to check whether the given string is in uppercase letters or not. It returns an array of boolean values where each value represents whether the corresponding element in the input array is in uppercase or not.
Syntax:
numpy.char.isupper(a)
Parameter:
Name | Description | Required / Optional |
---|---|---|
a: array_like of str or unicode | Input an array_like of string or unicode | Required |
Return value:
out : ndarray - Output array of bools
Example: Checking if a string has all uppercase letters using numpy.char.isupper()
>>> import numpy as np
>>> x = np.char.isupper('the quick brown fox')
>>> x
array(False)
In the above code, the input string has lowercase letters, so the method returns a boolean array with False for each character that is not an uppercase letter. Therefore, the output is array(False)
Pictorial Presentation:
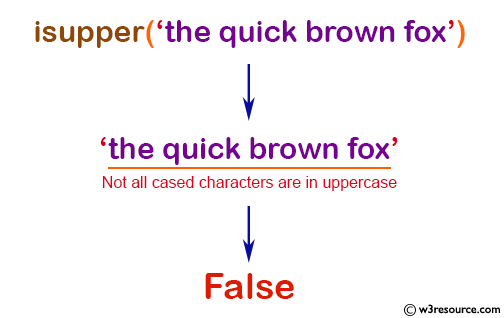
Example: Checking uppercase characters in a string using numpy.char.isupper()
>>> import numpy as np
>>> y = np.char.isupper('THE QUICK BROWN FOX')
>>> y
array(True)
In the above example the method returns an array of all True values, indicating that all the characters in the string are uppercase.
Pictorial Presentation:
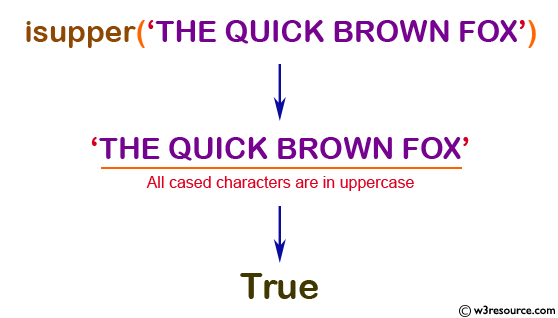
Example: Checking uppercase letters in a string using numpy.char.isupper()
>>> import numpy as np
>>> z = np.char.isupper('THE quick brown fox')
>>> z
array(False)
In the above code, the string 'THE quick brown fox' is passed to the function. The function returns True only when all the characters in the string are in uppercase. Here, since the 'q' in 'quick' is in lowercase, the function returns False.
Pictorial Presentation:
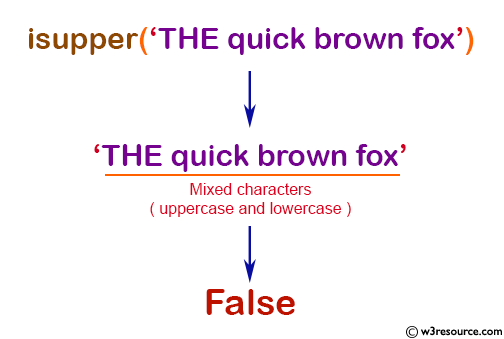
Python - NumPy Code Editor: