Python Exercises, Practice, Solution
This resource offers a total of 9475 Python problems for practice. It includes 2029 main exercises, each accompanied by solutions, detailed explanations, and upto four related problems.
Python Exercises:
Python is a versatile, high-level language known for its readability and concise syntax. It supports multiple programming paradigms, including object-oriented, imperative, and functional styles. It features dynamic typing, automatic memory management, and a robust standard library. This section is dedicated to practice exercises for those with beginner to intermediate Python skills. Happy coding as you enhance your programming abilities!
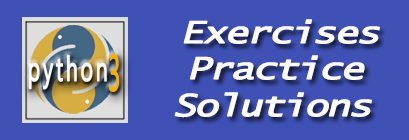
You may read our Python tutorial before solving the following exercises.
List of Python Exercises :
Python Basics- Python Basic (Part -I) [ 750 Exercises ]
- Python Basic (Part -II) [ 750 Exercises ]
- Python Programming Puzzles [ 500 Exercises ]
- Mastering Python [ 100 Exercises ]
- Python Data Types - String [ 565 Exercises ]
- Python JSON [ 45 Exercises ]
- Python Data Types - List [ 1400 Exercises ]
- Python Data Types - List Advanced [ 75 Exercises ]
- Python Data Types - Dictionary [ 450 Exercises ]
- Python Data Types - Tuple [ 165 Exercises ]
- Python Data Types - Sets [ 150 Exercises ]
- Python Data Types - Collections [ 180 Exercises ]
- Python Array [ 120 Exercises ]
- Python Enum [ 25 Exercises ]
- Python Unit test [ 50 Exercises ]
- Python Exception Handling [ 50 exercises ]
- Python Object-Oriented Programming [ 55 Exercises ]
- Python Decorator [ 60 Exercises ]
- Python functions [ 105 Exercises ]
- Python Lambda [ 260 Exercises ]
- Python Map [ 85 Exercises ]
- Python Itertools [ 220 exercises ]
- Python - Filter Function [ 55 Exercises ]
- Search and Sorting [ 195 Exercises ]
- Linked List [ 70 Exercises ]
- Binary Search Tree [ 30 Exercises ]
- Python heap queue algorithm [ 145 exercises ]
- Python Bisect [ 45 Exercises ]
- Python Boolean Data Type [ 50 Exercises ]
- Python None Data Type [ 50 Exercises ]
- Python Bytes and Byte Arrays Data Type [ 50 Exercises ]
- Python Memory Views Data Type [ 50 Exercises ]
- Python frozenset Views [ 50 Exercises ]
- Python NamedTuple [ 45 Exercises ]
- Python OrderedDict [ 50 Exercises ]
- Python Counter [ 50 Exercises ]
- Python Ellipsis (...) [ 45 Exercises ]
- Python built-in Modules [ 155 Exercises ]
- Python Operating System Services [ 90 Exercises ]
- Python Math [ 470 Exercises ]
- Python Requests [ 45 exercises ]
- Python SQLite Database [ 65 Exercises ]
- Python SQLAlchemy [ 70 exercises ]
- Python - PPrint [ 30 Exercises ]
Python GUI Tkinter, PyQt
- Python Tkinter Home
- Python Tkinter Basic [ 16 Exercises ]
- Python Tkinter layout management [ 11 Exercises ]
- Python Tkinter widgets [ 22 Exercises ]
- Python Tkinter Dialogs and File Handling [ 13 Exercises ]
- Python Tkinter Canvas and Graphics [ 14 Exercises ]
- Python Tkinter Events and Event Handling [ 13 Exercises ]
- Python Tkinter Customs Widgets and Themes [ 12 Exercises ]
- Python Tkinter - File Operations and Integration [12 exercises ]
- Python PyQt Basic [10 exercises ]
- Python PyQt Widgets[12 exercises ]
- Python PyQt Connecting Signals to Slots [15 exercises ]
- Python PyQt Event Handling [10 exercises ]
Python Challenges :
- Python Challenges: Part -1 [ 1- 64 ]
- More to come
Python Projects :
- Python Numbers : [ 11 Mini Projects ]
- Python Web Programming: [ 12 Mini Projects ]
- 100 Python Projects for Beginners with solution.
- Python Projects: Novel Coronavirus (COVID-19) [ 14 Exercises ]
- More to come
Learn Python packages using Exercises, Practice, Solution and explanation
Python urllib3 :
Python Metaprogramming :
Python GeoPy Package :
Python BeautifulSoup :
Python Arrow Module :
Python Web Scraping :
Python Natural Language Toolkit :
Python NumPy :
- Python NumPy Basic [ 59 Exercises ]
- Python NumPy arrays [ 205 Exercises ]
- Python NumPy Mathematics [ 41 Exercises ]
- Python NumPy Linear Algebra [ 19 Exercises ]
- Python NumPy Statistics [ 14 Exercises ]
- Python NumPy Random [ 17 Exercises ]
- Python NumPy Sorting and Searching [ 9 Exercises ]
- NumPy Advanced Indexing [ 20 exercises ]
- Python NumPy DateTime [ 7 Exercises ]
- Python NumPy String [ 22 Exercises ]
- NumPy Broadcasting [ 20 exercises ]
- NumPy Memory Layout [ 19 exercises ]
- NumPy Performance Optimization [ 20 exercises ]
- NumPy Interoperability [ 20 exercises ]
- NumPy I/O Operations [ 20 exercises ]
- NumPy Universal Functions [ 20 exercises ]
- NumPy Masked Arrays [ 20 exercises ]
- NumPy Structured Arrays [ 20 exercises ]
- NumPy Integration with SciPy [ 19 exercises ]
- Advanced NumPy [ 33 exercises ]
- Mastering NumPy [ 100 Exercises with ]
- More to come
Basic Operations and Arrays
Mathematics, Linear Algebra, and Statistics
Random Numbers
Sorting, Searching, and Indexing
Datetime and String Operations
Broadcasting and Memory Layout
Performance Optimization and Interoperability
Input/Output (I/O) Operations
Functions and Masked Arrays
Structured Arrays and SciPy Integration
Advanced Topics and Mastery
Python Pandas :
- Python Pandas Home
- Pandas Data Series [ 40 exercises with solution ]
- Pandas DataFrame [ 81 exercises with solution ]
- Pandas Index [ 26 exercises with solution ]
- Pandas String and Regular Expression [ 41 exercises with solution ]
- Pandas Joining and merging DataFrame [ 15 exercises with solution ]
- Pandas Grouping and Aggregating [ 32 exercises with solution ]
- Pandas Time Series [ 32 exercises with solution ]
- Pandas Filter [ 27 exercises with solution ]
- Pandas GroupBy [ 32 exercises with solution ]
- Pandas Handling Missing Values [ 20 exercises with solution ]
- Pandas Style [ 15 exercises with solution ]
- Pandas Excel Data Analysis [ 25 exercises with solution ]
- Pandas Pivot Table [ 32 exercises with solution ]
- Pandas Datetime [ 25 exercises with solution ]
- Pandas Plotting [ 19 exercises with solution ]
- Pandas Performance Optimization [ 20 exercises with solution ]
- Pandas Advanced Indexing and Slicing [ 15 exercises with solution ]
- Pandas SQL database Queries [ 24 exercises with solution ]
- Pandas Resampling and Frequency Conversion [ 15 exercises with solution ]
- Pandas Advanced Grouping and Aggregation [ 15 exercises with solution ]
- Pandas IMDb Movies Queries [ 17 exercises with solution ]
- Mastering NumPy: 100 Exercises with solutions for Python numerical computing
- Pandas Practice Set-1 [ 65 exercises with solution ]
- More to come
Pandas and NumPy Exercises :
- Pandas and NumPy for Data Analysis [ 37 Exercises ]
- More to come
Python Machine Learning :
More...
Note : Download Python from https://www.python.org/ftp/python/3.2/ and install in your system to execute the Python programs. You can read our Python Installation on Fedora Linux and Windows 7, if you are unfamiliar to Python installation.
You may accomplish the same task (solution of the exercises) in various ways, therefore the ways described here are not the only ways to do stuff. Rather, it would be great, if this helps you anyway to choose your own methods.
List of Exercises with Solutions :
- HTML CSS Exercises, Practice, Solution
- JavaScript Exercises, Practice, Solution
- jQuery Exercises, Practice, Solution
- jQuery-UI Exercises, Practice, Solution
- CoffeeScript Exercises, Practice, Solution
- Twitter Bootstrap Exercises, Practice, Solution
- C Programming Exercises, Practice, Solution
- C# Sharp Programming Exercises, Practice, Solution
- PHP Exercises, Practice, Solution
- Python Exercises, Practice, Solution
- R Programming Exercises, Practice, Solution
- Java Exercises, Practice, Solution
- SQL Exercises, Practice, Solution
- MySQL Exercises, Practice, Solution
- PostgreSQL Exercises, Practice, Solution
- SQLite Exercises, Practice, Solution
- MongoDB Exercises, Practice, Solution
More to Come !
Do not submit any solution of the above exercises at here, if you want to contribute go to the appropriate exercise page.
[ Want to contribute to Python exercises? Send your code (attached with a .zip file) to us at w3resource[at]yahoo[dot]com. Please avoid copyrighted materials.]
Test your Python skills with w3resource's quiz