Java ArrayDeque Class: remove() Method
public E remove()
The remove() method is used to retrieve and remove the head of the queue represented by a given deque.
This method differs from poll only in that it throws an exception if this deque is empty.
Package: java.util
Java Platform: Java SE 8
Syntax:
remove()
Return Value:
athe head of the queue represented by this deque
Return Value Type: E - the type of elements held in this collection
Throws:
NoSuchElementException - if this deque is empty
Pictorial Presentation
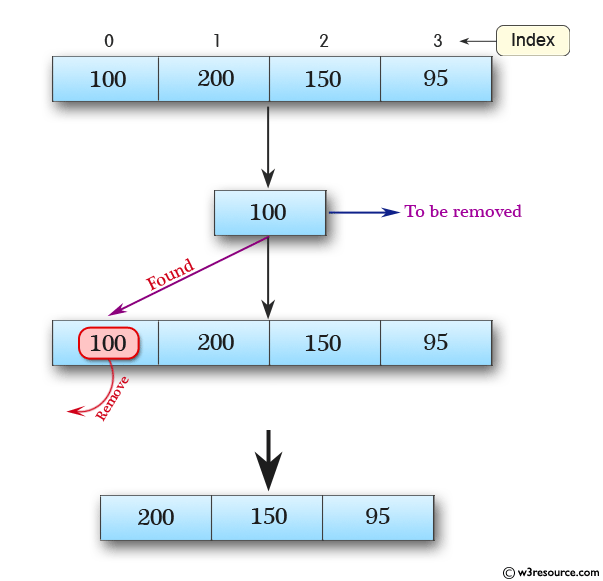
Example: Java ArrayDeque Class: remove() Method
import java.util.ArrayDeque;
import java.util.Deque;
public class Main {
public static void main(String[] args) {
// Create an array deque
Deque<Integer> deque = new ArrayDeque<Integer>(8);
// Use add() method to add elements in the deque
deque.add(100);
deque.add(200);
deque.add(150);
deque.add(95);
// Print all the elements of the original deque
System.out.println("Elements of the original deque:");
for (Integer number : deque) {
System.out.println("Number = " + number);
}
// Using remove() remove element at the first(head) postion
int re = deque.remove();
System.out.println("Element removed is: " + re);
// Print all the elements available in deque after pushing two elements using remove()
System.out.println("New deque:");
for (Integer number : deque) {
System.out.println("Number = " + number);
}
}
}
Output:
Elements of the original deque: Number = 100 Number = 200 Number = 150 Number = 95 Element removed is: 100 New deque: Number = 200 Number = 150 Number = 95
public boolean remove(Object o)
Removes a single instance of the specified element from this deque.
If the deque does not contain the element, it is unchanged. More formally, removes the first element e such that o.equals(e) (if such an element exists). Returns true if this deque contained the specified element (or equivalently, if this deque changed as a result of the call).
This method is equivalent to removeFirstOccurrence(Object).
Package: java.util
Java Platform: Java SE 8
Syntax:
remove(Object o)
Parameters:
Name | Description |
---|---|
o | element to be removed from this deque, if present |
Return Value:
true if this deque contained the specified element
Return Value Type: boolean
Example: Java ArrayDeque class: remove() Method
The following example fills a Java ArrayDeque class: remove() Method.
https://www.tutorialspoint.com/java/util/arraydeque_remove_object.htm
import java.util.ArrayDeque;
import java.util.Deque;
public class ArrayDequeDemo {
public static void main(String[] args) {
// create an empty array deque with an initial capacity
Deque<Integer> deque = new ArrayDeque<Integer>(8);
// use add() method to add elements in the deque
deque.add(25);
deque.add(30);
deque.add(20);
deque.add(40);
// printing all the elements available in deque
for (Integer number : deque) {
System.out.println("Number = " + number);
}
// deque contains element 30, returns true
boolean retval = deque.remove(30);
if (retval == true) {
System.out.println("element 30 is removed from the deque");
} else {
System.out.println("element 30 is not contained in the deque");
}
// deque does not contain element 15, returns false
boolean returnval = deque.remove(15);
if (returnval == true) {
System.out.println("element 15 is removed from the deque");
} else {
System.out.println("element 15 is not contained in the deque");
}
// printing remaining elements
for (Integer number : deque) {
System.out.println("Number = " + number);
}
}
}
http://www.technicalkeeda.com/java-tutorials/java-arraydeque-remove-method-examples
import java.util.ArrayDeque;
public class App {
public static void main(String[] args) {
ArrayDeque < String > celebrities = new ArrayDeque < String > ();
celebrities.add("Tom Cruise");
celebrities.add("Will Smith");
celebrities.add("Akshay Kumar");
celebrities.add("Jackie Chan");
celebrities.add("Will Smith");
System.out.println("Removed element is :- " + celebrities.remove());
System.out.println("Elements of deque after remove :- " + celebrities);
}
}
Output:
Java Code Editor:
Previous:push Method
Next:removeFirst Method
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/java-tutorial/util/arraydeque/java_arraydeque_remove.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics