Java ArrayDeque Class: iterator() Method
public Iterator<E> iterator()
Returns an iterator over the elements in this deque.
The elements will be ordered from first (head) to last (tail).
Package: java.util
Java Platform: Java SE 8
Syntax:
iterator()
Return Value:
an iterator over the elements in this deque
Return Value Type:Iterator<E - the type of elements held in this collection>
Pictorial Presentation
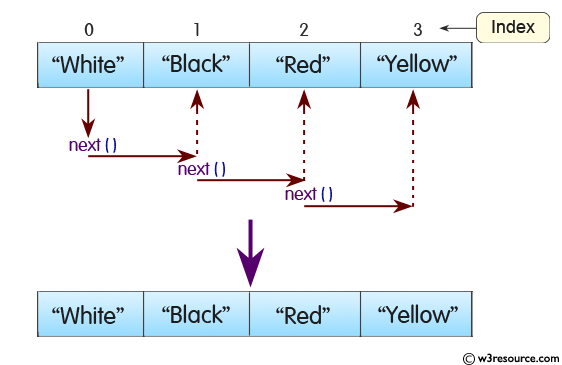
Example: Java ArrayDeque Class: iterator() Method
import java.util.ArrayDeque;
import java.util.Deque;
import java.util.Iterator;
public class Main {
public static void main(String[] args) {
// Create an empty array deque with an initial capacity
Deque<Integer> deque = new ArrayDeque<Integer>(8);
// Use add() method to add elements in the deque
deque.add(10);
deque.add(20);
deque.add(30);
deque.add(25);
// Print all the elements of the original deque.
System.out.println("Original elements of the deque: ");
for (Integer n : deque) {
System.out.println(n);
}
// iterator() is used to print all the elements
System.out.println("Print all the elements using iterator:");
for(Iterator itr = deque.iterator();itr.hasNext();) {
System.out.println(itr.next());
}
}
}
Output:
Original elements of the deque: 10 20 30 25 Print all the elements using iterator: 10 20 30 25
Java Code Editor:
Previous:isEmpty Method
Next:offer Method