Java ArrayDeque Class: offer()Method
public boolean offer(E e)
The offer() method is used to insert an element at the end of a given deque.
This method is equivalent to offerLast(E).
Package: java.util
Java Platform: Java SE 8
Syntax:
offer(E e)
Parameters:
Name | Description |
---|---|
e | the element to add |
Return Value:
true (as specified by Queue.offer(E))
Return Value Type: boolean
Throws:
NullPointerException - if the specified element is null
Pictorial Presentation
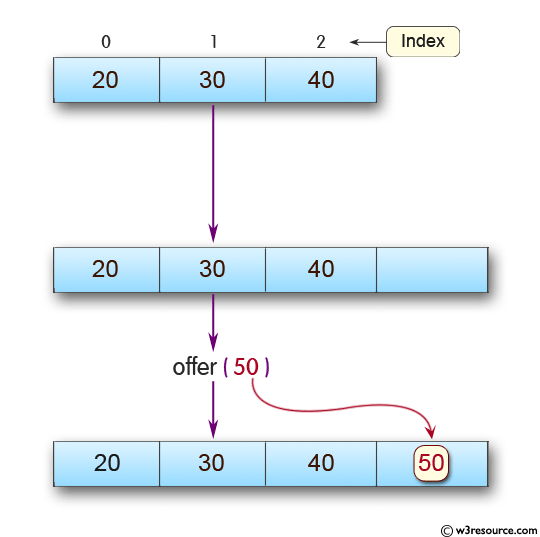
Example: Java ArrayDeque Class: offer() Method
import java.util.ArrayDeque;
import java.util.Deque;
public class Main {
public static void main(String[] args) {
// Create an empty array deque
Deque<Integer> deque = new ArrayDeque<Integer>(8);
// Use add() method to add elements in the deque
deque.add(20);
deque.add(30);
deque.add(40);
deque.add(35);
// Print all the elements of the original deque
System.out.println("Elements of the original deque:");
for (Integer n : deque) {
System.out.println(n);
}
// Insert 50 at the end of the deque using offer() method.
deque.offer(50);
// Print all the elements available in deque
System.out.println("Elements of the deque after insert an element at the end of the deque:");
for (Integer n : deque) {
System.out.println(n);
}
}
}
Output:
Elements of the original deque: 20 30 40 35 Elements of the deque after insert an element at the end of the deque: 20 30 40 35 50
Java Code Editor:
Previous:iterator Method
Next:offerFirst Method
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics