Java ArrayDeque Class: add() Method
public boolean add(E e)
The add() method is used to insert an given element at the end of this deque.
Note: This method is equivalent to addLast(E).
Package: java.util
Java Platform: Java SE 8
Syntax:
add(E e)Parameters:
Name | Description |
---|---|
e | The element to add. |
Return Value:
true (as specified by Collection.add(E))
Return Value Type: boolean
NullPointerException - if the specified element is nullThrows:
Pictorial Presentation
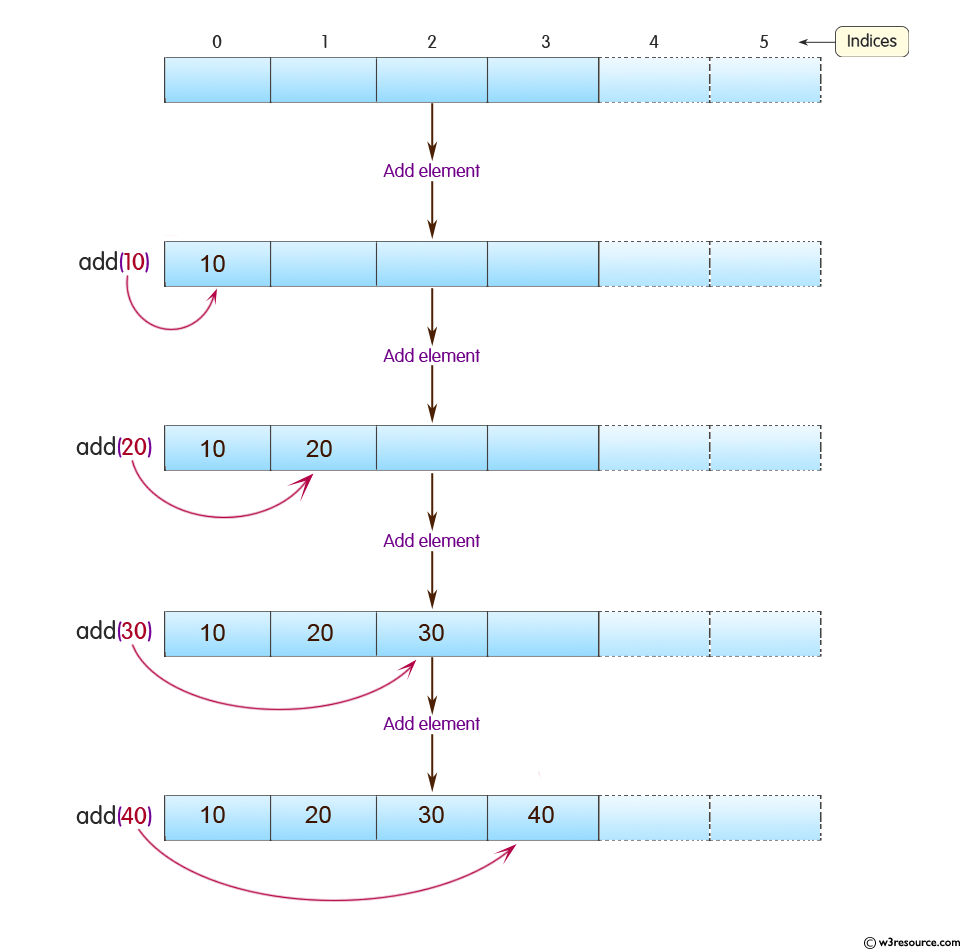
Example: Java ArrayDeque Class: add() Method
import java.util.ArrayDeque;
import java.util.Deque;
public class Main {
public static void main(String[] args) {
// Create an empty array deque with an initial capacity 5
Deque<Integer> deque = new ArrayDeque<Integer>(5);
// Use add() method to add 7 elements in the deque
deque.add(10);
deque.add(20);
deque.add(30);
deque.add(40);
deque.add(50);
deque.add(60);
deque.add(70);
// Print all the elements of deque
for (Integer n : deque) {
System.out.println(n);
}
}
}
Output:
10 20 30 40 50 60 70
Java Code Editor:
Previous:Arraydeque Class Methods Home
Next:addFirst Method
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/java-tutorial/util/arraydeque/java_arraydeque_add.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics