Java ArrayDeque Class: peekLast() Method
public E peekLast()
The peekLast() method is used to retrieve the last element of a given deque.
Package: java.util
Java Platform: Java SE 8
Syntax:
peekLast()
Return Value:
the tail of this deque, or null if this deque is empty
Return Value Type: E - the type of elements held in this collection
Pictorial Presentation
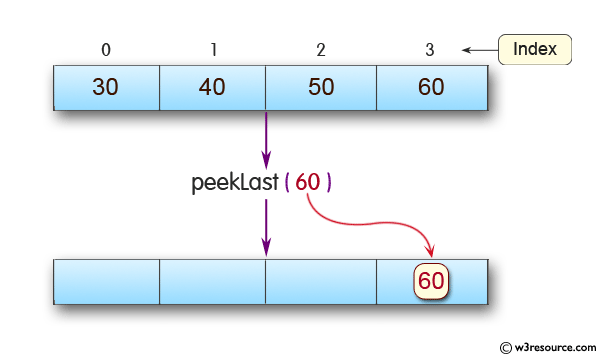
- Example: Java ArrayDeque Class: peekLast() Method
import java.util.ArrayDeque;
import java.util.Deque;
public class Main {
public static void main(String[] args) {
// Create an empty array deque
Deque<Integer> deque = new ArrayDeque<Integer>(8);
// Use add() method to add elements in the deque
deque.add(20);
deque.add(30);
deque.add(40);
deque.add(35);
// Print all the elements of the original deque
System.out.println("Elements of the original deque:");
for (Integer n : deque) {
System.out.println(n);
}
// Retrieve head of the queue
int val = deque.peekLast();
System.out.println("Retrieved Element is " + val);
// Print all the elements available in deque
System.out.println("Elements of the deque:");
for (Integer n : deque) {
System.out.println(n);
}
}
}
Output:
Elements of the original deque: 20 30 40 35 Retrieved Element is 20 Elements of the deque: 20 30 40 35
Java Code Editor:
Previous:peekFirst Method
Next:poll Method