Java ArrayDeque Class: element() Method
public E element()
The element() method is used to retrieve the head of the queue represented by this deque. This method differs from peek only in that it throws an exception if this deque is empty.
Note: This method is equivalent to getFirst().
Package: java.util
Java Platform: Java SE 8
Syntax:
element()
Return Value:
the head of the queue represented by this deque
Return Value Type: E - the type of elements held in this collection
Throws:
NoSuchElementException - if this deque is empty
Pictorial Presentation
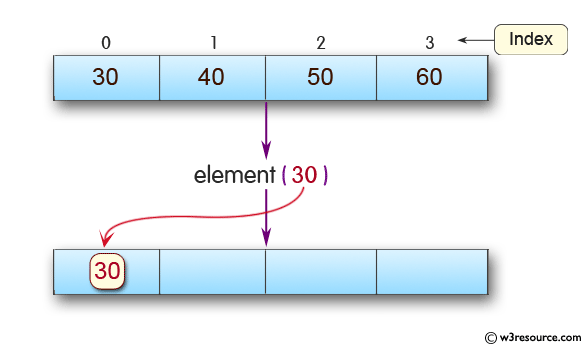
Example: Java ArrayDeque Class: element() Method
import java.util.ArrayDeque;
import java.util.Deque;
import java.util.Iterator;
public class Main {
public static void main(String[] args) {
// Create an empty array deque with an initial capacity
ArrayDeque<Integer> deque = new ArrayDeque<Integer>(8);
// Use add() method to add elements in the deque
deque.add(10);
deque.add(20);
deque.add(30);
deque.add(40);
// Print all the elements of the original deque
System.out.println("Original elements of the deque: ");
for (Integer n : deque) {
System.out.println(n);
}
// Retrieve the first element of the deque.
int e = deque.element();
System.out.println("Retrieved element: " + e);
}
}
Output:
Original elements of the deque: 10 20 30 40 Retrieved element: 10
Java Code Editor:
Previous: descendingIterator Method
Next: getFirst Method