NumPy Data type: can_cast() function
numpy.can_cast() function
The can_cast() function returns True if cast between data types can occur according to the casting rule. If from is a scalar or array scalar, also returns True if the scalar value can be cast without overflow or truncation to an integer.
Version: 1.15.0
Syntax:
numpy.can_cast(from_, to, casting='safe')
Parameter:
Name | Description | Required / Optional |
---|---|---|
from_ | Data type, scalar, or array to cast from. | Required |
to | Data type to cast to. | Required |
casting | Controls what kind of data casting may occur.
|
Optional |
Return value:
out : bool - True if cast can occur according to the casting rule.
Example-1: numpy.can_cast() function
>>> np.can_cast(np.int32, np.int64)
True
>>> np.can_cast(np.float64, complex)
True
>>> np.can_cast(complex, float)
False
>>>
>>> np.can_cast('i8', 'f8')
True
>>> np.can_cast('i8', 'f4')
False
>>> np.can_cast('i4', 'S4')
False
Pictorial Presentation:
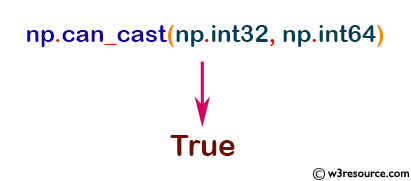
Pictorial Presentation:
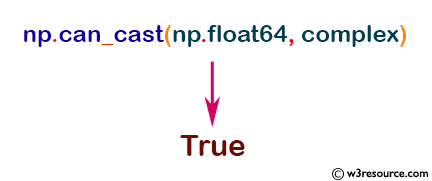
Pictorial Presentation:
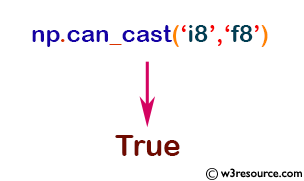
Pictorial Presentation:
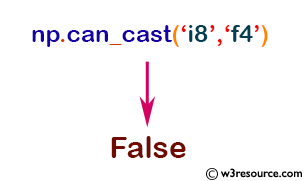
Example-2: numpy.can_cast() function
>>> np.can_cast(100, 'i1')
True
>>> np.can_cast(150, 'i1')
False
>>> np.can_cast(150, 'u1')
True
>>>
>>> np.can_cast(3.5e100, np.float32)
False
>>> np.can_cast(1000.0, np.float32)
True
Example-3: numpy.can_cast() function
>>> np.can_cast(np.array(1000.0), np.float32)
True
>>> np.can_cast(np.array([1000.0]), np.float32)
False
Example-4: numpy.can_cast() function
>>> import numpy as np
>>> np.can_cast('i8', 'i8', 'no')
True
>>> np.can_cast('<i8', '>i8', 'no')
False
>>>
>>> np.can_cast('<i8', '>i8', 'equiv')
True
>>> np.can_cast('<i4', '>i8', 'equiv')
False
>>>
>>> np.can_cast('<i4', '>i8', 'safe')
True
>>> np.can_cast('<i8', '>i4', 'safe')
False
>>>
>>> np.can_cast('<i8', '>i4', 'same_kind')
True
>>> np.can_cast('<i8', '>u4', 'same_kind')
False
>>>
>>> np.can_cast('<i8', '>u4', 'unsafe')
True
Python - NumPy Code Editor:
Previous:
NumPy Data type Home
Next:
promote_types()
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics