Python CGI Programming
Preface
This tutorial is aimed to get you started with Python CGI Programming. When it comes to running your Python Scripts on the web, you have to learn how Python can be executed as CGI Script. We will discuss how to configure your web server to make Python run as CGI, how a simple Python CGI Script looks like, what are the different components of the CGI script, what kind of errors you may find while running CGI script and what are the fixes, how to debug when things go wrong, about locking, sessions, cookies, how to generate HTML, and some basic issues about performance.
Configure Apache Web server for CGI
To get your server run CGI scripts properly, you have to configure your Web server. We will discuss how to configure your Apache web server to run CGI scripts.
Using ScriptAlias
You may set a directory as ScriptAlias Directive (options for configuring Apache). This way, Apache understands that all the files residing within that directory are CGI scripts. This may be the most simple way to run CGI Scripts on Apache. A typical ScriptAlias line looks like following in httpd.conf file of your Apache web server.
ScriptAlias /cgi-bin/ /usr/local/apache2/cgi-bin/
So, search ScriptAlias in your httpd.conf file and uncomment the line (remove preceding #) if you want to keep all your CGI files in the default directory specified by Apache. But this may not meet your requirement always. So, we will look some other options also for running Python as CGI.
Running CGI from a particular directory other than default one
You may use the following to prepare a particular directory to run CGI files.
<Directory /usr/local/apache2/htdocs/somedir>Options +ExecCGI</Directory>
Where 'somedir' is the directory of your preference.
If you use the above configuration. then you have to specify the following also to tell server extensions of the CGI files you winch to run.
AddHandler cgi-script .cgi .pl
So, the above tells Apache to run files with .cgi and .pl extensions as CGI
User Directories
If you want to run CGI files from user's directory, then you may use the following:
<Directory /home/*/public_html> Options +ExecCGI AddHandler cgi-script .cgi </Directory>
The above says Apache to run all files with .cgi extension present within user's directory as CGI.
Again, if you wish to run all files placed within user's directory as CGI, then you may use the following:
<Directory /home/*/public_html/cg-bin> Options +ExecCGI SetHandler cgi-script </Directory>
Using .htaccess
If you don't have access to your httpd.conf file, you may use a .htaccess file to run CGI scripts. To use files with certain extensions as CGI, configure you .htaccess file as following:
Options +ExecCGI AddHandler cgi-script cgi pl
If you would like to run all files residing within a directory as CGI, you may use the following:
Options +ExecCGI SetHandler cgi-script
Configure IIS Web server for CGI
We will discus now, how to configure Internet Information Server to run CGI scripts. We have used Windows 7 for this tutorial, which you may find similar while working on Windows Server 2008 or Windows Server 2008 R2 or Windows Vista. Note that Python interpreter must be installed on your Windows OS.
From Taskbar go to the 'Control Panel'. In 'Control Panel', click on 'Programs', then click 'Turn Windows features on or off. It may take while to open the 'Windows features'. In 'Windows features', under Internet Information Services, go to the 'Application Development Features' and click on the checkbox next to CGI. You may also select other options you want to configure along with.
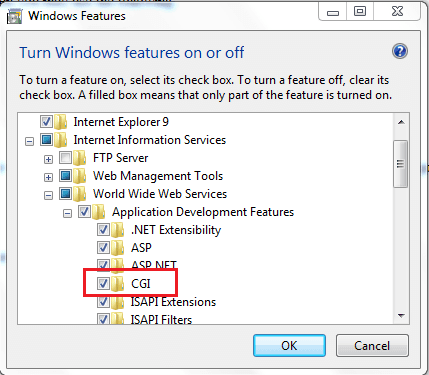
Click 'OK' and wait till the features are installed. Now run 'inetmgr' from Start and in the IIS manager window, go to the 'Default website' on the left hand side pane. Right click 'Default Web Site' and click on 'Add Application'.
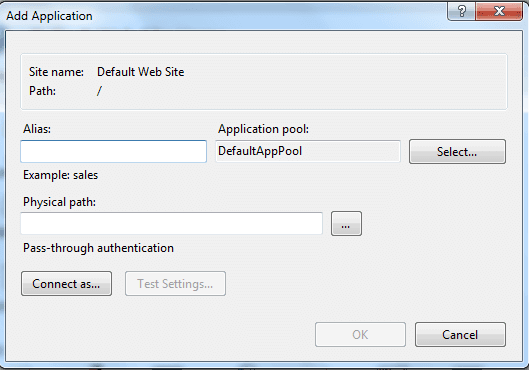
In the 'Alias' field, provide a name (e.g. PythonApp) and in the 'Physical path:' supply the path where your application PythonApp is placed. Click 'OK' now.
Back to the 'Default Web Site' in the left hand side pane now. Then in the middle pane, under 'IIS' heading, double click on 'Handler Mappings'. Select 'CGI-exe' and write click. Click on 'Add Script Map'. In the request path provide "*.py" and in 'Executable' provide Python installation path and its parameters (e.g. "C:\Python27\Python.exe %s %s"). Click 'OK'. Now you may keep your Python files within the said 'Pythonapp' folder and run it on IIS.
Configure LIGHTHTTPD Web server for CGI
Default configuration for running CGI on LightHttpd server looks like following:
$HTTP["url"] =~ "^/cgi-bin/" { cgi.assign = ( "" => "" ) }
If you want to run CGI from user directories, configure like following:
$HTTP["url"] =~ "^(/~[^/]+)?/cgi-bin/" { cgi.assign = ("" => "") }
Configure NGINX Web server for CGI
Unlike other web servers discussed here, NGINX does not execute external programs like CGI directly. But you may run Python as fcgi or uWSGI module.
Running your first Python file as CGI
Now you have configured your web server to run Python as CGI. try to run a simple Python file on your web server. Make sure you follow the points bellow before you do so:
1. Use an editor which supports proper EOL (End of Line) Conversion. This is important, particularly when you are creating the Python file on a different OS and then you upload or transfer it to another OS. Make sure you create the file with EOL conversion as your destination OS. So, if you are creating a file on Windows and later you want to run it in a Linux environment, make sure your EOL conversion is UNIX/OSX format. Notepad++ is an excellent editor for selecting EOL conversion if you are working on Windows and later want to transfer the files to a Linux / Unix machine.
2. When you are uploading the file to a server make sure you do so by ASCII mode. All the standard FTP clients (e.g. FileZilla) allows you to transfer files in ASCII mode.
3. If your machine, from where you will run your files is Linux/Unix or if you are working with a Windows Server-based domain environment, make sure the file has read, write, execute for Administrator, and read and execute for group and others.
4. Make sure your Python script do not have any syntax errors.
5. Make sure you have configured your web server properly for running CGI properly and also that you are placing your files to be run as CGI in the proper directory.
Here is an HTML file containing a simple HTML form pointing to a Python Script in cgi-bin folder.
<!doctype html>
<html>
<head>
<title>demo web file python3.2</title>
</head>
<body>
<form name="pyform" method="POST" action="/cgi-bin/form.py">
<input type="text" name="fname" />
<input type="submit" name="submit" value="Submit" />
</form>
</body>
</html>
The Python Script which the HTML form points to, is following:
#!/usr/local/bin/python3.2
print("Content-Type: text/html")
print()
import cgi,cgitb
cgitb.enable() #for debugging
form = cgi.FieldStorage()
name = form.getvalue('fname')
print("Name of the user is:",name)
Explanation of your first CGI file
The first line states the location of your Python installation. Then on second and third line the content type and a blank line are printed. Then cgi and cgitb modules are imported. cgitb.enable() helps you when things go wrong. But note that it does not help you if you have permission or file EOL conversion issues or sometimes even if you have syntactical errors in your python files. We will discuss that in detail later. The, for now, this will do the job.
Then we have stored FieldStoage class of cgi module in a form variable. Now, a form may behave like a dictionary. So, we extract the name supplied by the user in the HTML form using form.getvalue('fname'). Where fname is the name of the input field in the HTML form.
And now we print the name supplied by the user using print(). Note that the string 'name of the user is ' is wrapped by the double quote, ',' is used for concatenation and name is a variable thus kept outside any quotation.
You may see a live demo of the example shown above.
We are in the process of publishing series of tutorials discussing more in depth and real life-based stuff, so, let us know how useful it is for you and subscribe to our RSS feed for more tutorials on Python CGI Programming.
Previous: Python IDLE
Next: Python Syntax
Test your Python skills with w3resource's quiz