Python String
String
Python has a built-in string class named "str" with many useful features. String literals can be enclosed by either single or double, although single quotes are more commonly used.
Backslash escapes work the usual way within both single and double quoted literals -- e.g. \n \' \". A double quoted string literal can contain single quotes without any fuss (e.g. "I wouldn't be a painter") and likewise single quoted string can contain double quotes.
A string literal can span multiple lines, use triple quotes to start and end them. You can use single quotes too, but there must be a backslash \ at the end of each line to escape the newline.
Contents :
- String commands
- Initialize string literals in Python
- Access character(s) from a string
- Python strings are immutable
- Python string concatenation
- Using '*' operator
- String length
- Traverse string with a while or for loop
- String slices
- Search a character in a string
- Python Data Types: String - Exercises, Practice, Solution
String: Commands
# Strips all whitespace characters from both ends. <str> = <str>.strip() # Strips all passed characters from both ends. <str> = <str>.strip('<chars>')
# Splits on one or more whitespace characters. <list> = <str>.split() # Splits on 'sep' str at most 'maxsplit' times. <list> = <str>.split(sep=None, maxsplit=-1) # Splits on line breaks. Keeps them if 'keepends'. <list> = <str>.splitlines(keepends=False) # Joins elements using string as separator. <str> = <str>.join(<coll_of_strings>)
# Checks if string contains a substring. <bool> = <sub_str> in <str> # Pass tuple of strings for multiple options. <bool> = <str>.startswith(<sub_str>) # Pass tuple of strings for multiple options. <bool> = <str>.endswith(<sub_str>) # Returns start index of first match or -1. <int> = <str>.find(<sub_str>) # Same but raises ValueError if missing. <int> = <str>.index(<sub_str>)
# Replaces 'old' with 'new' at most 'count' times. <str> = <str>.replace(old, new [, count]) # True if str contains only numeric characters. <bool> = <str>.isnumeric() # Nicely breaks string into lines. <list> = textwrap.wrap(<str>, width)
- Also: 'lstrip()', 'rstrip()'.
- Also: 'lower()', 'upper()', 'capitalize()' and 'title()'.
Char
# Converts int to unicode char. <str> = chr(<int>) # Converts unicode char to int. <int> = ord(<str>)
>>> ord('0'), ord('9') (48, 57) >>> ord('A'), ord('Z') (65, 90) ord('a'), ord('z') (97, 122)
Initialize string literals in Python:
>>> s = "Python string"
>>> print(s)
Python string
>>> #using single quote and double quote
>>> s = "A Poor Woman's Journey."
>>> print(s)
A Poor Woman's Journey.
>>> s = "I read the article, 'A Poor Woman's Journey.'"
>>> print(s)
I read the article, 'A Poor Woman's Journey.'
>>> s = 'dir "c:\&temp\*.sas" /o:n /b > "c:\&temp\abc.txt"'
>>> print(s)
dir "c:\&temp\*.sas" /o:n /b > "c:\&tempbc.txt"
>>> #print multiple lines
>>> s = """jQuery exercises
JavaScript tutorial
Python tutorial and exercises ..."""
>>> print(s)
jQuery exercises
JavaScript tutorial
Python tutorial and exercises ...
>>> s = 'jQuery exercises\n JavaScript tutorial\n Python tutorial and exercises ...'
>>> print(s)
jQuery exercises
JavaScript tutorial
Python tutorial and exercises ...
>>>
Access character(s) from a string:
Characters in a string can be accessed using the standard [ ] syntax, and like Java and C++, Python uses zero-based indexing, so if str is 'hello' str[2] is 'l'. If the index is out of bounds for the string, Python raises an error.
>>> a = "Python string"
>>> print (a)
Python string
>>> b = a[2]
>>> print(b)
t
>>> a[0]
'P'
>>>
Explanation :
- The above statement selects character at position number 2 from a and assigns it to b.
- The expression in brackets is called an index that indicates which character we are interested.
- The index is an offset from the beginning of the string, and the offset of the first letter is zero.
We can use any expression, including variables and operators, as an index
>>> a = "Python string"
>>> b = a[4+3]
>>> print(b)
s
>>>
The value of the index has to be an integer.
>>> a = "Python string"
>>> a[2.3]
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: string indices must be integers
>>>
Negative indices:
Alternatively, we can use negative indices, which count backward from the end of the
Index -1 gives the last character.
>>> a = "Python string"
>>> a[-2]
'n'
>>> a[-8]
'n'
>>>
Python strings are immutable:
- Python strings are "immutable" which means they cannot be changed after they are created
- Therefore we can not use [ ] operator on the left side of an assignment.
- We can create a new string that is a variation of the original.
>>> a = "PYTHON"
>>> a[0] = "x"
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: 'str' object does not support item assignment
>>> a = "PYTHON"
# Concatenates a new first letter on to a slice of greeting.
>>> b = "X" + a[1:]
>>> print(b)
XYTHON
# It has no effect on the original string.
>>> print(a)
PYTHON
>>>
Python String concatenation:
The '+' operator is used to concatenate two strings.
>>> a = "Python" + "String"
>>> print(a)
PythonString
>>>
You can also use += to concatenate two strings.
>>> a = "Java"
>>> b = "Script"
>>> a+=b
>>> print(a)
JavaScript
>>>
Using '*' operator:
>>> a = "Python" + "String"
>>> b = "<" + a*3 + ">"
>>> print(b)
<PythonStringPythonStringPythonString>
>>>
String length:
len() is a built-in function which returns the number of characters in a string:
>>> a = "Python string"
>>> len(a)
13
>>> a[13]
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
IndexError: string index out of range
>>> a[12]
'g'
>>>
Traverse string with a while or for loop
- A lot of computations involve processing a string character by character at a time.
- They start at the beginning, select each character, in turn, do something to it, and continue until the end.
- This pattern of processing is called a traversal.
- One can traverse string with a while or for loop:
Code:
a = "STRING"
i = 0
while i < len(a):
c = a[i]
print(c)
i = i + 1
Output:
>>> S T R I N G >>>
Traversal with a for loop :
Print entire string on one line using for loop.
Code:
a = "Python"
i = 0
new=" "
for i in range (0,len(a)):
b=a[i]
# + used for concatenation
new = new+b
i = i + 1
# prints each char on one line
print(b)
print(new)
Output:
>>> P P y Py t Pyt h Pyth o Pytho n Python >>>
String slices:
A segment of a string is called a slice. The "slice" syntax is used to get sub-parts of a string. The slice s[start:end] is the elements beginning at the start (p) and extending up to but not including end (q).
- The operator [p:q] returns the part of the string from the pth character to the qth character, including the first but excluding the last.
- If we omit the first index (before the colon), the slice starts at the beginning of the string.
- If you omit the second index, the slice goes to the end of the string:
- If the first index is greater than or equal to the second the result is an empty string, represented by two quotation marks.
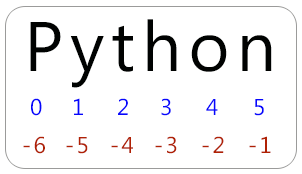
>> s = "Python"
- s[1:4] is 'yth' -- chars starting at index 1 and extending up to but not including index 4
- s[1:] is 'ython' -- omitting either index defaults to the start or end of the string
- s[:] is 'Python' -- Copy of the whole thing
- s[1:100] is 'ython' -- an index that is too big is truncated down to the string length
Python uses negative numbers to give easy access to the chars at the end of the string. Negative index numbers count back from the end of the string:
- s[-1] is 'n' -- last char (1st from the end)
- s[-4] is 't' -- 4th from the end
- s[:-3] is 'pyt' -- going up to but not including the last 3 chars
- s[-3:] is 'hon' -- starting with the 3rd char from the end and extending to the end of the string.
Example-2
>>> a = "Python String"
>>> print (a[0:5])
Pytho
>>> print(a[6:11])
Stri
>>> print(a[5:13])
n String
>>>
>>> a = "Python String"
>>> print(a[:8])
Python S
>>> print(a[4:])
on String
>>> print(a[6:3])
>>>
Example-3
>>> a = "PYTHON"
>>> a[3]
'H'
>>> a[0:4]
'PYTH'
>>> a[3:5]
'HO'
>>> # The first two characters
...
>>> a[:2]
'PY'
>>> # All but the first three characters
...
>>> a[3:]
'HON'
>>>
Search a character in a string:
Code:
def search(char,str):
L=len(str)
print(L)
i = 0
while i < L:
if str[i]== char:
return 1
i = i + 1
return -1
print(search("P","PYTHON"))
Output:
>>> 6 1 >>>
- It takes a character and finds the index where that character appears in a string.
- If the character is not found, the function returns -1.
Another example
def search(char,str):
L=len(str)
print(L)
i = 0
while i < L:
if str[i]== char:
return 1
i = i + 1
return -1
print(search("S","PYTHON"))
Output:
>>> 6 -1 >>>
Test your Python skills with w3resource's quiz
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics