NumPy: numpy.tri() function
numpy.tri() function
The numpy.tri() function returns an array with ones at and below the k-th diagonal and zeros elsewhere, where k is the given parameter.
Syntax:
numpy.tri(N, M=None, k=0, dtype=<class 'float'>)
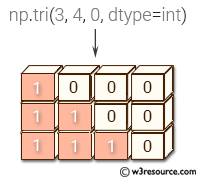
Parameters:
Name | Description | Required / Optional |
---|---|---|
N | Number of rows in the array. | int |
M | Number of columns in the array. By default, M is taken equal to N. | optional |
k | The sub-diagonal at and below which the array is filled. k = 0 is the main diagonal, while k < 0 is below it, and k > 0 is above. The default is 0. | optional |
dtype | Data type of the returned array. The default is float. | optional |
Return value:
tri : ndarray of shape (N, M) - Array with its lower triangle filled with ones and zero elsewhere; in other words T[i,j] == 1 for i <= j + k, 0 otherwise.
Example: Creating a triangular array with NumPy using np.tri()
>>> import numpy as np
>>> np.tri(4, 6, 0, dtype=int)
array([[1, 0, 0, 0, 0, 0],
[1, 1, 0, 0, 0, 0],
[1, 1, 1, 0, 0, 0],
[1, 1, 1, 1, 0, 0]])
The code creates a 4 x 6 triangular array using the NumPy function np.tri().
The first two arguments of np.tri() specify the number of rows and columns, respectively, in the resulting array (4 rows and 6 columns in this case).
The third argument, k=0, specifies the diagonal offset. When k=0, the main diagonal is used, which means that the elements above the diagonal are set to 0 and the elements below the diagonal are set to 1.
Finally, the dtype=int argument specifies the data type of the array as integers.
Pictorial Presentation:
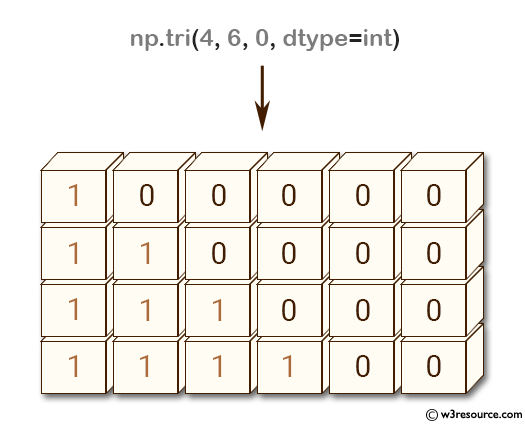
Example: Creating a Triangular Matrix with Numpy using np.tri()
>>> import numpy as np
>>> np.tri(4, 6, 1, dtype=int)
array([[1, 1, 0, 0, 0, 0],
[1, 1, 1, 0, 0, 0],
[1, 1, 1, 1, 0, 0],
[1, 1, 1, 1, 1, 0]])
In the above example, we create a 4x6 triangular matrix with k=1 (the diagonal above the main diagonal) and dtype=int. The resulting array has ones in the diagonal above the main diagonal, and zeros elsewhere, forming an upper triangular matrix.
Pictorial Presentation:
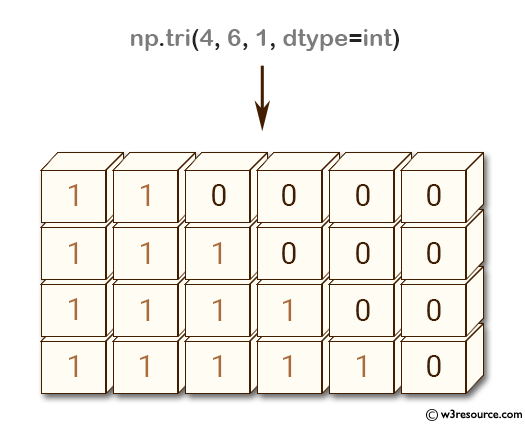
Example: Creating a Lower Triangular Matrix using Numpy's tri function
>>> import numpy as np
>>> np.tri(4, 6, -1, dtype=int)
array([[0, 0, 0, 0, 0, 0],
[1, 0, 0, 0, 0, 0],
[1, 1, 0, 0, 0, 0],
[1, 1, 1, 0, 0, 0]])
In the above code, the function np.tri(4, 6, -1, dtype=int) creates a 4x6 matrix with -1 offset, which means that the first lower sub-diagonal is filled with ones and the rest with zeros. The dtype parameter is set to int to create an integer array.
Pictorial Presentation:
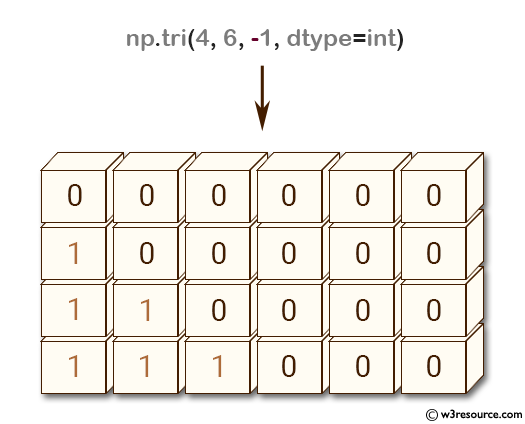
Python - NumPy Code Editor:
Previous: diagflat()
Next: tril()