NumPy: numpy.hsplit() function
numpy.hsplit() function
The numpy.hsplit() function is used to split an array into multiple sub-arrays horizontally (column-wise).
hsplit is equivalent to split with axis=1, the array is always split along the second axis regardless of the array dimension.
The function is a useful tool for manipulating and analyzing multi-dimensional arrays in NumPy.
Syntax:
numpy.hsplit(ary, indices_or_sections)
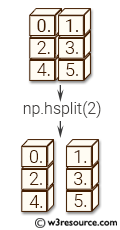
Parameters:
Name | Description | Required / Optional |
---|---|---|
ary | Input array | Required |
indices_or_sections | Indices or sections |
Return value:
Example: Splitting a NumPy array horizontally using numpy.hsplit()
>>> import numpy as np
>>> a = np.arange(16.0).reshape(4,4)
>>> np.hsplit(a, 2)
[array([[ 0., 1.],
[ 4., 5.],
[ 8., 9.],
[ 12., 13.]]), array([[ 2., 3.],
[ 6., 7.],
[ 10., 11.],
[ 14., 15.]])]
In the above code, a NumPy array 'a' is created using arange() function, and then reshaped to a 4x4 matrix. The np.hsplit() function is then used to horizontally split the array into 2 parts.
Pictorial Presentation:
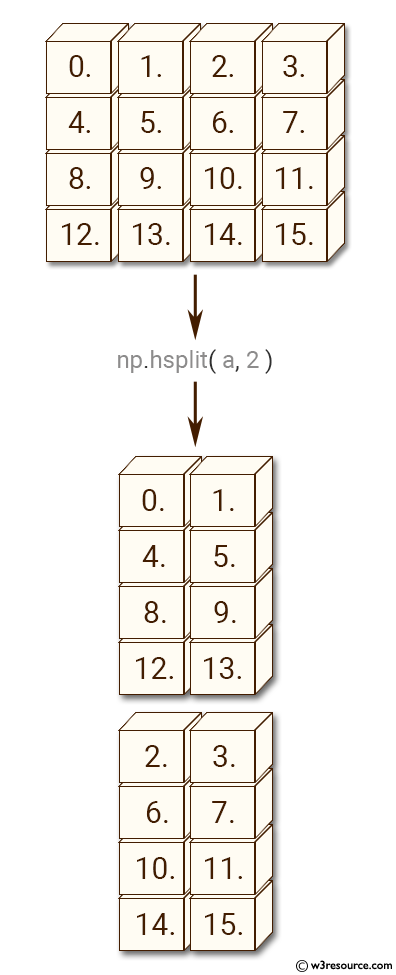
Example: Splitting a numpy array horizontally with numpy.hsplit() and specific indices
>>> import numpy as np
>>> a = np.arange(16.0).reshape(4,4)
>>> np.hsplit(a, np.array([3,6]))
[array([[ 0., 1., 2.],
[ 4., 5., 6.],
[ 8., 9., 10.],
[ 12., 13., 14.]]), array([[ 3.],
[ 7.],
[ 11.],
[ 15.]]), array([], shape=(4, 0), dtype=float64)]
In the above code the np.hsplit() function is called with the array 'a' and an array of specific indices [3,6]. This will split the array 'a' into three arrays at positions 3 and 6 along the horizontal axis.
- The first array contains the first three columns of the original array 'a'.
- The second array contains the fourth column of the original array 'a'.
- The third array is empty because there are no columns between the indices 6 and 4.
Python - NumPy Code Editor:
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/numpy/manipulation/hsplit.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics