NumPy: numpy.column_stack() function
numpy.column_stack() function
Stack 1-D arrays as columns into a 2-D array.
Take a sequence of 1-D arrays and stack them as columns to make a single 2-D array. 2-D arrays are stacked as-is, just like with hstack. 1-D arrays are turned into 2-D columns first.
This function is useful when we want to combine two arrays in a column-wise fashion, which means we combine the arrays by their columns, i.e., we stack one array's columns next to the other array's columns.
The numpy.column_stack() function takes a sequence of 1-D or 2-D arrays and stacks them as columns into a 2-D array. If the input arrays are 1-D, then they will be converted to 2-D arrays first.
Syntax:
numpy.column_stack(tup)
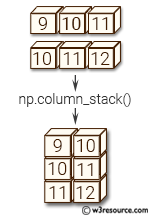
Parameter:
Name | Description | Required / Optional |
---|---|---|
tup | Arrays to stack. All of them must have the same first dimension. | Required |
Return value:
stacked : 2-D array The array formed by stacking the given arrays.
Example: Column stacking two numpy arrays using numpy.column_stack()
>>> import numpy as np
>>> x = np.array((3,4,5))
>>> y = np.array((4,5,6))
>>> np.column_stack((x,y))
array([[3, 4],
[4, 5],
[5, 6]])
The above code creates two numpy arrays "x" and "y" with values (3,4,5) and (4,5,6) respectively. Then, it calls the "np.column_stack()" function and passes both the arrays as arguments.
The "np.column_stack()" function stacks two 1-D arrays as columns into a 2-D array. The output of the function is a 2-D array with shape (3, 2) in this case, where each row contains elements from both input arrays.
Here, the function returns a 2-D array where the first column contains elements from array "x" and the second column contains elements from array "y". The resulting array is [[3, 4], [4, 5], [5, 6]]
Pictorial Presentation:
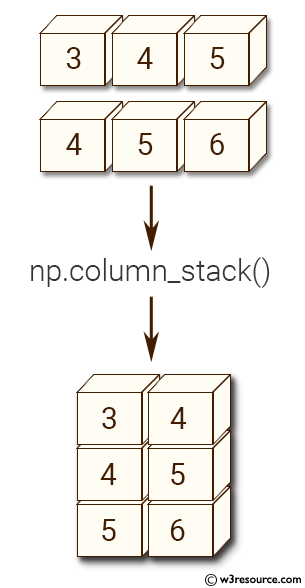
Python - NumPy Code Editor: