MongoDb : Python Driver
Introduction
The PyMongo distribution contains tools for interacting with MongoDB database from Python. This tutorial covers installing PyMongo on various operating systems, connection and basic database operation like insert, update, delete and searching with PyMongo.
Installing with pip
pip is a tool for installing and managing Python packages. Use pip to install PyMongo on platforms other than Windows.
$ pip install pymongo
To get a specific version of pymongo:
$ pip install pymongo==2.6.3
To upgrade using pip :
$ pip install --upgrade pymongo
Installing with easy_install
Setuptools provides download, build, install, upgrade, and uninstall Python packages. To use easy_install from setuptools do :
$ easy_install pymongo
To upgrade do :
$ easy_install -U pymongo
MS Windows installers
The PyMongo distribution in windows is supported and tested on Python 2.x (where x >= 4) and Python 3.x (where x >= 1). PyMongo versions <= 1.3 also supported Python 2.3, but that is no longer supported.
Before installing the driver on Windows :
- To complete the installation operation, root or administrator privileges may be required.
- Before installing the Python distribution on your system, enable python.exe in Windows %PATH% setting ( path environment variable) or manually add it to, if it is not enabled.
Installing the Windows Driver :
Select and download the MSI installer packages from here as per your requirement.
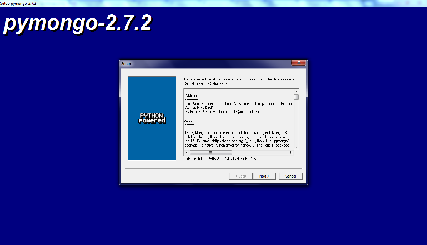
Working with MongoDB and PyMongo
We assumes that a MongoDB instance is running on the default host and port. Before you start, make sure that you have the PyMongo distribution installed. In the Python shell, the following should run without raising an exception :
>>> import pymongo
>>>
Making a Connection with MongoClient
To create a MongoClient to the running mongod instance execute the following code :
>>> from pymongo import MongoClient
>>> client = MongoClient()
The above code will connect on the default host and port.
You can specify the host and port explicitly, as follows :
>>> from pymongo import MongoClient
>>> client = MongoClient('localhost', 27017)
You can also use the MongoDB URI format :
>>> from pymongo import MongoClient
>>> client = MongoClient('mongodb://localhost:27017/')
Getting a Database
A single instance of MongoDB can support multiple independent databases. Here is the command :
>>> db = client.testdb
or (using dictionary style access):
>>> db = client['testdb']
Insert a document
To insert a document into a collection you can use the insert() method. When a document is inserted a special key, "_id", is automatically added if the document does not already contain an "_id" key. The value of "_id" must be unique across the collection. insert() returns the value of "_id" for the inserted document.
>>> import pymongo
>>> from pymongo import MongoClient
>>> client = MongoClient()
>>> db = client.testdb
>>> student1 = {"name": "Arun",
"classid": 'V',
"rollno": 1}
>>> students = db.students
>>> students_id = students.insert(student1)
>>> students_id
ObjectId('548c02cd838d1f11b0d17d52')
>>>
Add two more records :
>>> student2 = {"name": "David",
"classid": 'V',
"rollno": 2}
>>> student3 = {"name": "Shekhar",
"classid": 'V',
"rollno": 3}
>>> students = db.students
>>> students_id = students.insert(student2)
>>> students_id = students.insert(student3)
Find a document
The find_one() method returns a single document matching a query (or none if there are no matches). It is useful when you know there is only one matching document. Here find_one() method is used to get the first document from the students collection :
>>> import pymongo
>>> from pymongo import MongoClient
>>> client = MongoClient()
>>> db = client.testdb
>>> students = db.students
>>> students.find_one()
{'classid': 'V', '_id': ObjectId('548c02cd838d1f11b0d17d52'), 'name': 'Arun', 'rollno': 1}
>>>
The returned document contains an "_id", which was automatically added on insert.
Find a specific document :
find_one() method also supports querying on specific elements that the resulting document must match. Let search the student "Shekhar".
>>> import pymongo
>>> from pymongo import MongoClient
>>> client = MongoClient()
>>> db = client.testdb
>>> students = db.students
>>> students.find_one({"name": "Shekhar"})
{'classid': 'V', '_id': ObjectId('548c058a838d1f11b0d17d54'), 'name': 'Shekhar', 'rollno': 3}
>>>
You can also find a student by its _id, which in our example is an ObjectId.
Multiple documents Query
To get more than one document as the result of a query we use the find() method. find() returns a cursor instance, which allows us to iterate over all matching documents. For example, we can iterate over every document in the students collection :
>>> import pymongo
>>> from pymongo import MongoClient
>>> client = MongoClient()
>>> db = client.testdb
>>> students = db.students
>>> for student in students.find():
student
{'classid': 'V', '_id': ObjectId('548c02cd838d1f11b0d17d52'), 'name': 'Arun', 'rollno': 1}
{'classid': 'V', '_id': ObjectId('548c0584838d1f11b0d17d53'), 'name': 'David', 'rollno': 2}
{'classid': 'V', '_id': ObjectId('548c058a838d1f11b0d17d54'), 'name': 'Shekhar', 'rollno': 3}
>>>
Range Queries
You can use the query to get a set of documents from our collection. For example, if you want to get all documents where "rollno" >= 2, the code will be :
>>> import pymongo
>>> from pymongo import MongoClient
>>> client = MongoClient()
>>> db = client.testdb
>>> students = db.students
>>> for student in students.find({"rollno": {"$gte": 2}}):
print(student)
{'classid': 'V', '_id': ObjectId('548c0584838d1f11b0d17d53'), 'name': 'David', 'rollno': 2}
{'classid': 'V', '_id': ObjectId('548c058a838d1f11b0d17d54'), 'name': 'Shekhar', 'rollno': 3}
>>>
Count documents
Using count() operation you can count the number of documents in a collection.
>>> students.count()
3
>>>
Update a specific document
You can edit a specific document using update() operation.
>>> students.find_one({"name": "Shekhar"})
{'classid': 'V', '_id': ObjectId('548c058a838d1f11b0d17d54'), 'name': 'Shekhar', 'rollno': 3}
>>> students.update({"name": "Shekhar"}, {'$set':{'rollno': 12}})
{'updatedExisting': True, 'nModified': 1, 'ok': 1, 'n': 1}
>>> students.find_one({"name": "Shekhar"})
{'classid': 'V', '_id': ObjectId('548c058a838d1f11b0d17d54'), 'name': 'Shekhar', 'rollno': 12}
>>>
Remove a specific document
You can remove a specific document using remove() operation.
>>> students.remove({"rollno": 12})
{'ok': 1, 'n': 1}
>>> for student in students.find():
student
{'classid': 'V', '_id': ObjectId('548c02cd838d1f11b0d17d52'), 'name': 'Arun', 'rollno': 1}
{'classid': 'V', '_id': ObjectId('548c0584838d1f11b0d17d53'), 'name': 'David', 'rollno': 2}
>>>
Previous:
Java DRIVER
Next:
PHP DRIVER
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics