Java String: copyValueOf() Method
copyValueOf() Method - Array of characters converted to a string
Contents:
public static String copyValueOf(char[] data)
Equivalent to valueOf(char[]).
The copyValueOf() method is used to create a string that represents the character sequence in the array specified.
Visual presentation of Java String copyValueOf() Method
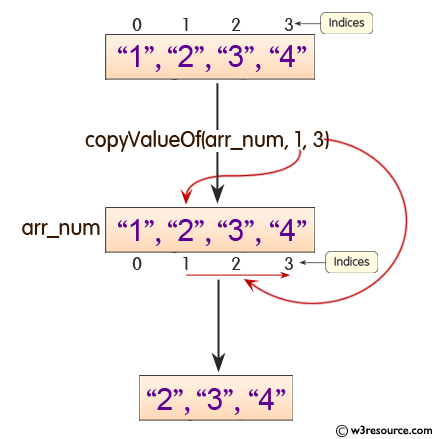
Java Platform: Java SE 8 and above
Syntax copyValueOf() method
copyValueOf(char[] data)
Parameters copyValueOf() method
Name | Description | Type |
---|---|---|
data | The character array. | String |
Return Value copyValueOf() method
- A String that contains the characters of the character array.
Return Value Type: String
Example: Java String copyValueOf() Method
The following example shows the usage of java String() method.
public class Example {
public static void main(String[] args)
{
// Character array with data.
char[] arr_num = new char[] { '1', '2', '3', '4' };
// Create a String containig the contents of arr_num
// starting at index 1 for length 2.
String str = String.copyValueOf(arr_num, 1, 3);
// Display the results of the new String.
System.out.println("\nThe book contains " + str +" pages.\n");
}
}
Output:
The book contains 234 pages.
public static String copyValueOf(char[] data, int offset, int count)
Equivalent to valueOf(char[], int, int).
Java Platform: Java SE 8
Syntax:
copyValueOf(char[] data, int offset, int count)
Parameters:
Name | Description | Type |
---|---|---|
data | the character array. | String |
offset | initial offset of the subarray. | String |
count | the length of the subarray. | String |
Return Value :
- A String that contains the characters of the specified subarray of the character array.
Return Value Type: String
Throws:
IndexOutOfBoundsException - if offset is negative, or count is negative, or offset+count is larger than data.length.
Example: Java String copyValueOf() Method
The following example shows the usage of java String() method.
public class Example {
public static void main(String[] arg) {
char[] textArray = { 'w', '3', 'r', 'e', 's', 'o', 'u', 'c', 'o', 'm', ' ', 'j', 'a', 'v', 'a',
'r', 'c','e', '.', 'c', 'o', 'm' };
String text = String.copyValueOf(textArray, 6, 2);
System.out.println();
System.out.println(text);
System.out.println();
}
}
Output:
uc
Java Code Editor:
Previous:contentEquals Method
Next:endsWith Method
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics