SQL Correlated Subqueries
Correlated Subqueries
SQL Correlated Subqueries retrieve data from a table referenced in the outer query. They are termed "correlated" because the subquery's execution is influenced by the outer query's rows. When using correlated subqueries, it's essential to employ a table alias (or correlation name) to clarify the table reference intended for use within the subquery.
In an uncorrelated subquery, the subquery is executed independently first, and its result is then used as a value in the outer query. In contrast, a correlated subquery is linked to the outer query by referencing a column and is executed repeatedly, once for each row processed by the outer query.
An alias is a shorthand or nickname assigned to a table by placing it immediately after the table name in the FROM clause. This practice is useful when retrieving data from multiple tables simultaneously.
Example: SQL Correlated Subqueries
The following correlated subqueries retrieve ord_num, ord_amount, cust_code, and agent_code from the table orders ('a' and 'b' are aliases for the orders and agents tables), based on the following conditions -
The agent_code in the orders table must match the agent_code in the agents table, and the agent_name in the agents table must be 'Alex',
the following SQL statement can be used:
Sample table: ordersORD_NUM ORD_AMOUNT ADVANCE_AMOUNT ORD_DATE CUST_CODE AGENT_CODE ORD_DESCRIPTION ---------- ---------- -------------- --------- --------------- --------------- ----------------- 200114 3500 2000 15-AUG-08 C00002 A008 200122 2500 400 16-SEP-08 C00003 A004 200118 500 100 20-JUL-08 C00023 A006 200119 4000 700 16-SEP-08 C00007 A010 200121 1500 600 23-SEP-08 C00008 A004 200130 2500 400 30-JUL-08 C00025 A011 200134 4200 1800 25-SEP-08 C00004 A005 200108 4000 600 15-FEB-08 C00008 A004 200103 1500 700 15-MAY-08 C00021 A005 200105 2500 500 18-JUL-08 C00025 A011 200109 3500 800 30-JUL-08 C00011 A010 200101 3000 1000 15-JUL-08 C00001 A008 200111 1000 300 10-JUL-08 C00020 A008 200104 1500 500 13-MAR-08 C00006 A004 200106 2500 700 20-APR-08 C00005 A002 200125 2000 600 10-OCT-08 C00018 A005 200117 800 200 20-OCT-08 C00014 A001 200123 500 100 16-SEP-08 C00022 A002 200120 500 100 20-JUL-08 C00009 A002 200116 500 100 13-JUL-08 C00010 A009 200124 500 100 20-JUN-08 C00017 A007 200126 500 100 24-JUN-08 C00022 A002 200129 2500 500 20-JUL-08 C00024 A006 200127 2500 400 20-JUL-08 C00015 A003 200128 3500 1500 20-JUL-08 C00009 A002 200135 2000 800 16-SEP-08 C00007 A010 200131 900 150 26-AUG-08 C00012 A012 200133 1200 400 29-JUN-08 C00009 A002 200100 1000 600 08-JAN-08 C00015 A003 200110 3000 500 15-APR-08 C00019 A010 200107 4500 900 30-AUG-08 C00007 A010 200112 2000 400 30-MAY-08 C00016 A007 200113 4000 600 10-JUN-08 C00022 A002 200102 2000 300 25-MAY-08 C00012 A012Sample table : agents
+------------+----------------------+--------------------+------------+-----------------+---------+ | AGENT_CODE | AGENT_NAME | WORKING_AREA | COMMISSION | PHONE_NO | COUNTRY | +------------+----------------------+--------------------+------------+-----------------+---------+ | A007 | Ramasundar | Bangalore | 0.15 | 077-25814763 | | | A003 | Alex | London | 0.13 | 075-12458969 | | | A008 | Alford | New York | 0.12 | 044-25874365 | | | A011 | Ravi Kumar | Bangalore | 0.15 | 077-45625874 | | | A010 | Santakumar | Chennai | 0.14 | 007-22388644 | | | A012 | Lucida | San Jose | 0.12 | 044-52981425 | | | A005 | Anderson | Brisban | 0.13 | 045-21447739 | | | A001 | Subbarao | Bangalore | 0.14 | 077-12346674 | | | A002 | Mukesh | Mumbai | 0.11 | 029-12358964 | | | A006 | McDen | London | 0.15 | 078-22255588 | | | A004 | Ivan | Torento | 0.15 | 008-22544166 | | | A009 | Benjamin | Hampshair | 0.11 | 008-22536178 | | +------------+----------------------+--------------------+------------+-----------------+---------+
SQL Code:
-- Selecting specific columns from the orders table and aliasing it as 'a'
SELECT a.ord_num, a.ord_amount, a.cust_code, a.agent_code
-- Filtering orders based on agent_code
FROM orders a
-- Specifying the condition for filtering
WHERE a.agent_code = (
-- Selecting agent_code from agents table and aliasing it as 'b'
SELECT b.agent_code
-- Filtering agents based on agent_name
FROM agents b
-- Specifying the condition for filtering
WHERE b.agent_name = 'Alex'
);
Explanation:
- This SQL query retrieves specific columns from the "orders" table, aliasing it as 'a'.
- It filters the orders based on the agent_code.
- The subquery selects the agent_code from the "agents" table, aliasing it as 'b'.
- It filters the agents based on the agent_name being 'Alex'.
- The main query then selects orders where the agent_code matches the result of the subquery, meaning it selects orders handled by the agent with the name 'Alex'.
Output:
ORD_NUM ORD_AMOUNT CUST_CODE AGENT_CODE ---------- ---------- ---------- ---------- 200127 2500 C00015 A003 200100 1000 C00015 A003
The inner of the above query returns the 'agent_code' A003.
The simplified form of above code is:
SQL Code:
SELECT a.ord_num,a.ord_amount,a.cust_code,a.agent_code
FROM orders a
WHERE a.agent_code='A003';
Visual Presentation:
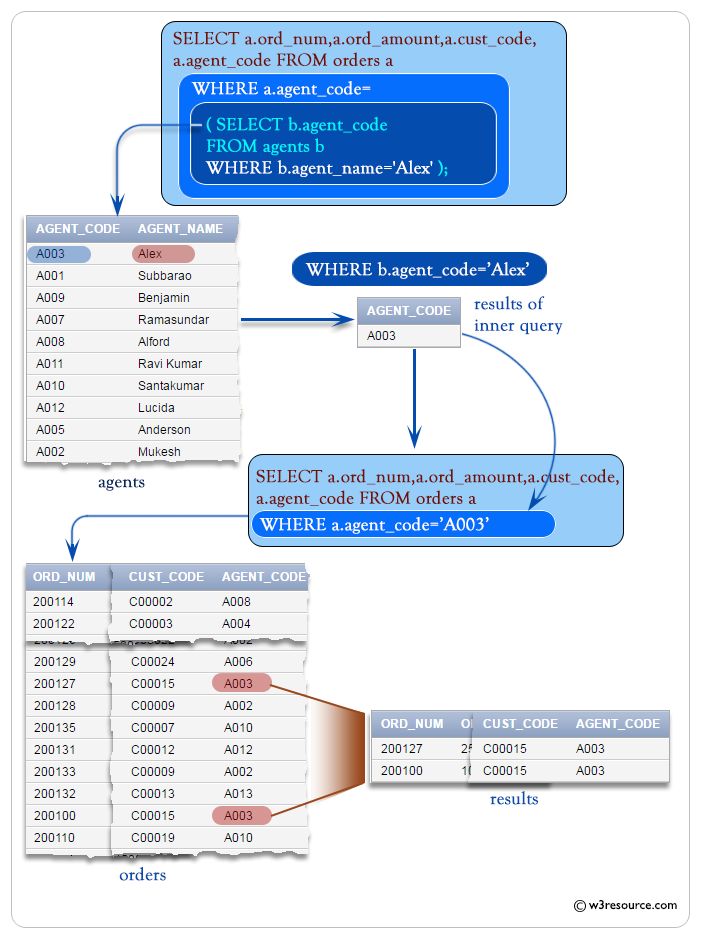
Using EXISTS with a Correlated Subquery
We have already used the EXISTS operator to check the existence of a result of a subquery. EXISTS operator can be used in correlated subqueries also. Using EXISTS the following query display the employee_id, manager_id, first_name and last_name of those employees who manage other employees.
Sample table: employeesemployee_id first_name last_name email phone_number hire_date job_id salary commission_pct manager_id department_id ----------- ---------- ---------- ---------- ------------ ---------- ---------- ---------- -------------- ---------- ------------- 100 Steven King SKING 515.123.4567 6/17/1987 AD_PRES 24000 90 101 Neena Kochhar NKOCHHAR 515.123.4568 6/18/1987 AD_VP 17000 100 90 102 Lex De Haan LDEHAAN 515.123.4569 6/19/1987 AD_VP 17000 100 90 103 Alexander Hunold AHUNOLD 590.423.4567 6/20/1987 IT_PROG 9000 102 60 104 Bruce Ernst BERNST 590.423.4568 6/21/1987 IT_PROG 6000 103 60 105 David Austin DAUSTIN 590.423.4569 6/22/1987 IT_PROG 4800 103 60 106 Valli Pataballa VPATABAL 590.423.4560 6/23/1987 IT_PROG 4800 103 60 107 Diana Lorentz DLORENTZ 590.423.5567 6/24/1987 IT_PROG 4200 103 60 108 Nancy Greenberg NGREENBE 515.124.4569 6/25/1987 FI_MGR 12000 101 100 109 Daniel Faviet DFAVIET 515.124.4169 6/26/1987 FI_ACCOUNT 9000 108 100 110 John Chen JCHEN 515.124.4269 6/27/1987 FI_ACCOUNT 8200 108 100 111 Ismael Sciarra ISCIARRA 515.124.4369 6/28/1987 FI_ACCOUNT 7700 108 100 112 Jose Manue Urman JMURMAN 515.124.4469 6/29/1987 FI_ACCOUNT 7800 108 100 113 Luis Popp LPOPP 515.124.4567 6/30/1987 FI_ACCOUNT 6900 108 100 114 Den Raphaely DRAPHEAL 515.127.4561 7/1/1987 PU_MAN 11000 100 30 115 Alexander Khoo AKHOO 515.127.4562 7/2/1987 PU_CLERK 3100 114 30 116 Shelli Baida SBAIDA 515.127.4563 7/3/1987 PU_CLERK 2900 114 30 117 Sigal Tobias STOBIAS 515.127.4564 7/4/1987 PU_CLERK 2800 114 30 118 Guy Himuro GHIMURO 515.127.4565 7/5/1987 PU_CLERK 2600 114 30 119 Karen Colmenares KCOLMENA 515.127.4566 7/6/1987 PU_CLERK 2500 114 30 120 Matthew Weiss MWEISS 650.123.1234 7/7/1987 ST_MAN 8000 100 50 121 Adam Fripp AFRIPP 650.123.2234 7/8/1987 ST_MAN 8200 100 50 122 Payam Kaufling PKAUFLIN 650.123.3234 7/9/1987 ST_MAN 7900 100 50 123 Shanta Vollman SVOLLMAN 650.123.4234 7/10/1987 ST_MAN 6500 100 50 124 Kevin Mourgos KMOURGOS 650.123.5234 7/11/1987 ST_MAN 5800 100 50 125 Julia Nayer JNAYER 650.124.1214 7/12/1987 ST_CLERK 3200 120 50 126 Irene Mikkilinen IMIKKILI 650.124.1224 7/13/1987 ST_CLERK 2700 120 50 127 James Landry JLANDRY 650.124.1334 7/14/1987 ST_CLERK 2400 120 50 128 Steven Markle SMARKLE 650.124.1434 7/15/1987 ST_CLERK 2200 120 50 129 Laura Bissot LBISSOT 650.124.5234 7/16/1987 ST_CLERK 3300 121 50 130 Mozhe Atkinson MATKINSO 650.124.6234 7/17/1987 ST_CLERK 2800 121 50 131 James Marlow JAMRLOW 650.124.7234 7/18/1987 ST_CLERK 2500 121 50 132 TJ Olson TJOLSON 650.124.8234 7/19/1987 ST_CLERK 2100 121 50 133 Jason Mallin JMALLIN 650.127.1934 7/20/1987 ST_CLERK 3300 122 50 134 Michael Rogers MROGERS 650.127.1834 7/21/1987 ST_CLERK 2900 122 50 135 Ki Gee KGEE 650.127.1734 7/22/1987 ST_CLERK 2400 122 50 136 Hazel Philtanker HPHILTAN 650.127.1634 7/23/1987 ST_CLERK 2200 122 50 137 Renske Ladwig RLADWIG 650.121.1234 7/24/1987 ST_CLERK 3600 123 50 138 Stephen Stiles SSTILES 650.121.2034 7/25/1987 ST_CLERK 3200 123 50 139 John Seo JSEO 650.121.2019 7/26/1987 ST_CLERK 2700 123 50 140 Joshua Patel JPATEL 650.121.1834 7/27/1987 ST_CLERK 2500 123 50 141 Trenna Rajs TRAJS 650.121.8009 7/28/1987 ST_CLERK 3500 124 50 142 Curtis Davies CDAVIES 650.121.2994 7/29/1987 ST_CLERK 3100 124 50 143 Randall Matos RMATOS 650.121.2874 7/30/1987 ST_CLERK 2600 124 50 144 Peter Vargas PVARGAS 650.121.2004 7/31/1987 ST_CLERK 2500 124 50 145 John Russell JRUSSEL 011.44.1344. 8/1/1987 SA_MAN 14000 0.4 100 80 146 Karen Partners KPARTNER 011.44.1344. 8/2/1987 SA_MAN 13500 0.3 100 80 147 Alberto Errazuriz AERRAZUR 011.44.1344. 8/3/1987 SA_MAN 12000 0.3 100 80 148 Gerald Cambrault GCAMBRAU 011.44.1344. 8/4/1987 SA_MAN 11000 0.3 100 80 149 Eleni Zlotkey EZLOTKEY 011.44.1344. 8/5/1987 SA_MAN 10500 0.2 100 80 150 Peter Tucker PTUCKER 011.44.1344. 8/6/1987 SA_REP 10000 0.3 145 80 151 David Bernstein DBERNSTE 011.44.1344. 8/7/1987 SA_REP 9500 0.25 145 80 152 Peter Hall PHALL 011.44.1344. 8/8/1987 SA_REP 9000 0.25 145 80 153 Christophe Olsen COLSEN 011.44.1344. 8/9/1987 SA_REP 8000 0.2 145 80 154 Nanette Cambrault NCAMBRAU 011.44.1344. 8/10/1987 SA_REP 7500 0.2 145 80 155 Oliver Tuvault OTUVAULT 011.44.1344. 8/11/1987 SA_REP 7000 0.15 145 80 156 Janette King JKING 011.44.1345. 8/12/1987 SA_REP 10000 0.35 146 80 157 Patrick Sully PSULLY 011.44.1345. 8/13/1987 SA_REP 9500 0.35 146 80 158 Allan McEwen AMCEWEN 011.44.1345. 8/14/1987 SA_REP 9000 0.35 146 80 159 Lindsey Smith LSMITH 011.44.1345. 8/15/1987 SA_REP 8000 0.3 146 80 160 Louise Doran LDORAN 011.44.1345. 8/16/1987 SA_REP 7500 0.3 146 80 161 Sarath Sewall SSEWALL 011.44.1345. 8/17/1987 SA_REP 7000 0.25 146 80 162 Clara Vishney CVISHNEY 011.44.1346. 8/18/1987 SA_REP 10500 0.25 147 80 163 Danielle Greene DGREENE 011.44.1346. 8/19/1987 SA_REP 9500 0.15 147 80 164 Mattea Marvins MMARVINS 011.44.1346. 8/20/1987 SA_REP 7200 0.1 147 80 165 David Lee DLEE 011.44.1346. 8/21/1987 SA_REP 6800 0.1 147 80 166 Sundar Ande SANDE 011.44.1346. 8/22/1987 SA_REP 6400 0.1 147 80 167 Amit Banda ABANDA 011.44.1346. 8/23/1987 SA_REP 6200 0.1 147 80 168 Lisa Ozer LOZER 011.44.1343. 8/24/1987 SA_REP 11500 0.25 148 80 169 Harrison Bloom HBLOOM 011.44.1343. 8/25/1987 SA_REP 10000 0.2 148 80 170 Tayler Fox TFOX 011.44.1343. 8/26/1987 SA_REP 9600 0.2 148 80 171 William Smith WSMITH 011.44.1343. 8/27/1987 SA_REP 7400 0.15 148 80 172 Elizabeth Bates EBATES 011.44.1343. 8/28/1987 SA_REP 7300 0.15 148 80 173 Sundita Kumar SKUMAR 011.44.1343. 8/29/1987 SA_REP 6100 0.1 148 80 174 Ellen Abel EABEL 011.44.1644. 8/30/1987 SA_REP 11000 0.3 149 80 175 Alyssa Hutton AHUTTON 011.44.1644. 8/31/1987 SA_REP 8800 0.25 149 80 176 Jonathon Taylor JTAYLOR 011.44.1644. 9/1/1987 SA_REP 8600 0.2 149 80 177 Jack Livingston JLIVINGS 011.44.1644. 9/2/1987 SA_REP 8400 0.2 149 80 178 Kimberely Grant KGRANT 011.44.1644. 9/3/1987 SA_REP 7000 0.15 149 179 Charles Johnson CJOHNSON 011.44.1644. 9/4/1987 SA_REP 6200 0.1 149 80 180 Winston Taylor WTAYLOR 650.507.9876 9/5/1987 SH_CLERK 3200 120 50 181 Jean Fleaur JFLEAUR 650.507.9877 9/6/1987 SH_CLERK 3100 120 50 182 Martha Sullivan MSULLIVA 650.507.9878 9/7/1987 SH_CLERK 2500 120 50 183 Girard Geoni GGEONI 650.507.9879 9/8/1987 SH_CLERK 2800 120 50 184 Nandita Sarchand NSARCHAN 650.509.1876 9/9/1987 SH_CLERK 4200 121 50 185 Alexis Bull ABULL 650.509.2876 9/10/1987 SH_CLERK 4100 121 50 186 Julia Dellinger JDELLING 650.509.3876 9/11/1987 SH_CLERK 3400 121 50 187 Anthony Cabrio ACABRIO 650.509.4876 9/12/1987 SH_CLERK 3000 121 50 188 Kelly Chung KCHUNG 650.505.1876 9/13/1987 SH_CLERK 3800 122 50 189 Jennifer Dilly JDILLY 650.505.2876 9/14/1987 SH_CLERK 3600 122 50 190 Timothy Gates TGATES 650.505.3876 9/15/1987 SH_CLERK 2900 122 50 191 Randall Perkins RPERKINS 650.505.4876 9/16/1987 SH_CLERK 2500 122 50 192 Sarah Bell SBELL 650.501.1876 9/17/1987 SH_CLERK 4000 123 50 193 Britney Everett BEVERETT 650.501.2876 9/18/1987 SH_CLERK 3900 123 50 194 Samuel McCain SMCCAIN 650.501.3876 9/19/1987 SH_CLERK 3200 123 50 195 Vance Jones VJONES 650.501.4876 9/20/1987 SH_CLERK 2800 123 50 196 Alana Walsh AWALSH 650.507.9811 9/21/1987 SH_CLERK 3100 124 50 197 Kevin Feeney KFEENEY 650.507.9822 9/22/1987 SH_CLERK 3000 124 50 198 Donald OConnell DOCONNEL 650.507.9833 9/23/1987 SH_CLERK 2600 124 50 199 Douglas Grant DGRANT 650.507.9844 9/24/1987 SH_CLERK 2600 124 50 200 Jennifer Whalen JWHALEN 515.123.4444 9/25/1987 AD_ASST 4400 101 10 201 Michael Hartstein MHARTSTE 515.123.5555 9/26/1987 MK_MAN 13000 100 20 202 Pat Fay PFAY 603.123.6666 9/27/1987 MK_REP 6000 201 20 203 Susan Mavris SMAVRIS 515.123.7777 9/28/1987 HR_REP 6500 101 40 204 Hermann Baer HBAER 515.123.8888 9/29/1987 PR_REP 10000 101 70 205 Shelley Higgins SHIGGINS 515.123.8080 9/30/1987 AC_MGR 12000 101 110 206 William Gietz WGIETZ 515.123.8181 10/1/1987 AC_ACCOUNT 8300 205 110
SQL Code:
-- Selecting specific columns from the employees table and aliasing it as 'a'
SELECT employee_id, manager_id, first_name, last_name
-- Filtering employees based on the EXISTS condition
FROM employees a
-- Specifying the condition for filtering using the EXISTS keyword
WHERE EXISTS (
-- Selecting employee_id from employees table and aliasing it as 'b'
SELECT employee_id
-- Filtering employees based on manager_id
FROM employees b
-- Specifying the condition for filtering
WHERE b.manager_id = a.employee_id
);
Explanation:
- This SQL query retrieves specific columns from the "employees" table, aliasing it as 'a'.
- It filters the employees based on the existence of records in a correlated subquery.
- The subquery selects the employee_id from the "employees" table, aliasing it as 'b'.
- It filters the employees based on whether there exists an employee with a manager_id that matches the employee_id from the outer query.
- The main query then selects employees where the subquery returns at least one record, meaning it selects employees who manage other employees.
Visual Presentation:
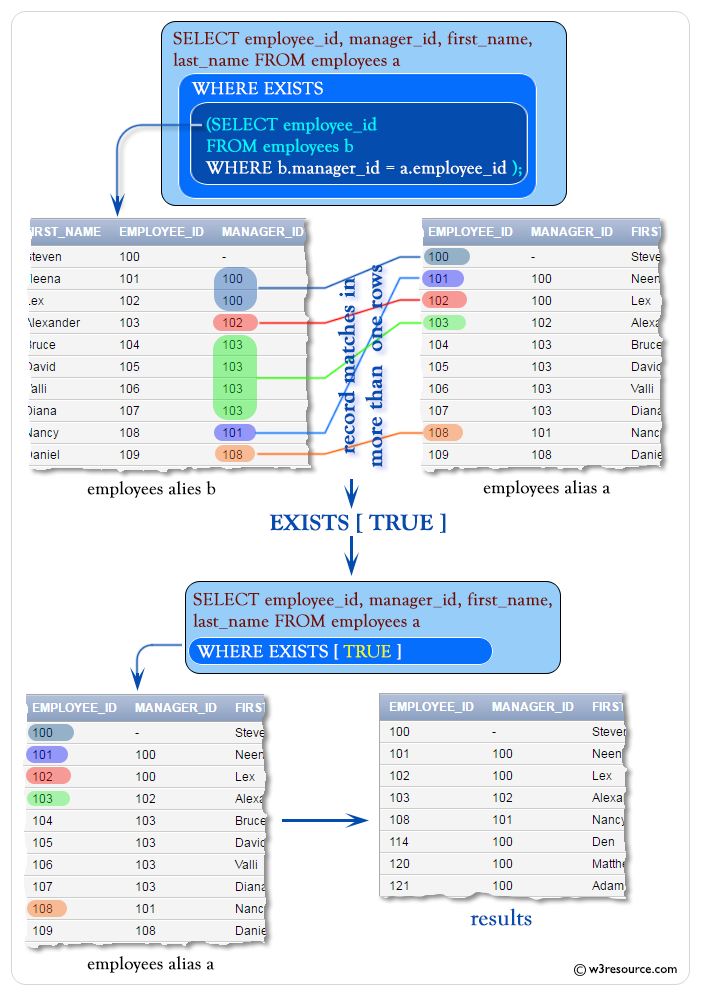
Output:
EMPLOYEE_ID MANAGER_ID FIRST_NAME LAST_NAME ----------- ---------- -------------------- --------------- 100 Steven King 101 100 Neena Kochhar 102 100 Lex De Haan 103 102 Alexander Hunold 108 101 Nancy Greenberg 114 100 Den Raphaely 120 100 Matthew Weiss 121 100 Adam Fripp 122 100 Payam Kaufling 123 100 Shanta Vollman 124 100 Kevin Mourgos 145 100 John Russell 146 100 Karen Partners 147 100 Alberto Errazuriz 148 100 Gerald Cambrault 149 100 Eleni Zlotkey 201 100 Michael Hartstein 205 101 Shelley Higgins
Using NOT EXISTS with a Correlated Subquery
NOT EXISTS is logically opposite of EXISTS operator. NOT EXISTS is used when we need to check if rows do not exist in the results returned by a subquery. Using NOT EXISTS the following query display the employee_id, manager_id, first_name and last_name of those employees who have no manager status. This query is opposite to the previous one.
Sample table: employeesemployee_id first_name last_name email phone_number hire_date job_id salary commission_pct manager_id department_id ----------- ---------- ---------- ---------- ------------ ---------- ---------- ---------- -------------- ---------- ------------- 100 Steven King SKING 515.123.4567 6/17/1987 AD_PRES 24000 90 101 Neena Kochhar NKOCHHAR 515.123.4568 6/18/1987 AD_VP 17000 100 90 102 Lex De Haan LDEHAAN 515.123.4569 6/19/1987 AD_VP 17000 100 90 103 Alexander Hunold AHUNOLD 590.423.4567 6/20/1987 IT_PROG 9000 102 60 104 Bruce Ernst BERNST 590.423.4568 6/21/1987 IT_PROG 6000 103 60 105 David Austin DAUSTIN 590.423.4569 6/22/1987 IT_PROG 4800 103 60 106 Valli Pataballa VPATABAL 590.423.4560 6/23/1987 IT_PROG 4800 103 60 107 Diana Lorentz DLORENTZ 590.423.5567 6/24/1987 IT_PROG 4200 103 60 108 Nancy Greenberg NGREENBE 515.124.4569 6/25/1987 FI_MGR 12000 101 100 109 Daniel Faviet DFAVIET 515.124.4169 6/26/1987 FI_ACCOUNT 9000 108 100 110 John Chen JCHEN 515.124.4269 6/27/1987 FI_ACCOUNT 8200 108 100 111 Ismael Sciarra ISCIARRA 515.124.4369 6/28/1987 FI_ACCOUNT 7700 108 100 112 Jose Manue Urman JMURMAN 515.124.4469 6/29/1987 FI_ACCOUNT 7800 108 100 113 Luis Popp LPOPP 515.124.4567 6/30/1987 FI_ACCOUNT 6900 108 100 114 Den Raphaely DRAPHEAL 515.127.4561 7/1/1987 PU_MAN 11000 100 30 115 Alexander Khoo AKHOO 515.127.4562 7/2/1987 PU_CLERK 3100 114 30 116 Shelli Baida SBAIDA 515.127.4563 7/3/1987 PU_CLERK 2900 114 30 117 Sigal Tobias STOBIAS 515.127.4564 7/4/1987 PU_CLERK 2800 114 30 118 Guy Himuro GHIMURO 515.127.4565 7/5/1987 PU_CLERK 2600 114 30 119 Karen Colmenares KCOLMENA 515.127.4566 7/6/1987 PU_CLERK 2500 114 30 120 Matthew Weiss MWEISS 650.123.1234 7/7/1987 ST_MAN 8000 100 50 121 Adam Fripp AFRIPP 650.123.2234 7/8/1987 ST_MAN 8200 100 50 122 Payam Kaufling PKAUFLIN 650.123.3234 7/9/1987 ST_MAN 7900 100 50 123 Shanta Vollman SVOLLMAN 650.123.4234 7/10/1987 ST_MAN 6500 100 50 124 Kevin Mourgos KMOURGOS 650.123.5234 7/11/1987 ST_MAN 5800 100 50 125 Julia Nayer JNAYER 650.124.1214 7/12/1987 ST_CLERK 3200 120 50 126 Irene Mikkilinen IMIKKILI 650.124.1224 7/13/1987 ST_CLERK 2700 120 50 127 James Landry JLANDRY 650.124.1334 7/14/1987 ST_CLERK 2400 120 50 128 Steven Markle SMARKLE 650.124.1434 7/15/1987 ST_CLERK 2200 120 50 129 Laura Bissot LBISSOT 650.124.5234 7/16/1987 ST_CLERK 3300 121 50 130 Mozhe Atkinson MATKINSO 650.124.6234 7/17/1987 ST_CLERK 2800 121 50 131 James Marlow JAMRLOW 650.124.7234 7/18/1987 ST_CLERK 2500 121 50 132 TJ Olson TJOLSON 650.124.8234 7/19/1987 ST_CLERK 2100 121 50 133 Jason Mallin JMALLIN 650.127.1934 7/20/1987 ST_CLERK 3300 122 50 134 Michael Rogers MROGERS 650.127.1834 7/21/1987 ST_CLERK 2900 122 50 135 Ki Gee KGEE 650.127.1734 7/22/1987 ST_CLERK 2400 122 50 136 Hazel Philtanker HPHILTAN 650.127.1634 7/23/1987 ST_CLERK 2200 122 50 137 Renske Ladwig RLADWIG 650.121.1234 7/24/1987 ST_CLERK 3600 123 50 138 Stephen Stiles SSTILES 650.121.2034 7/25/1987 ST_CLERK 3200 123 50 139 John Seo JSEO 650.121.2019 7/26/1987 ST_CLERK 2700 123 50 140 Joshua Patel JPATEL 650.121.1834 7/27/1987 ST_CLERK 2500 123 50 141 Trenna Rajs TRAJS 650.121.8009 7/28/1987 ST_CLERK 3500 124 50 142 Curtis Davies CDAVIES 650.121.2994 7/29/1987 ST_CLERK 3100 124 50 143 Randall Matos RMATOS 650.121.2874 7/30/1987 ST_CLERK 2600 124 50 144 Peter Vargas PVARGAS 650.121.2004 7/31/1987 ST_CLERK 2500 124 50 145 John Russell JRUSSEL 011.44.1344. 8/1/1987 SA_MAN 14000 0.4 100 80 146 Karen Partners KPARTNER 011.44.1344. 8/2/1987 SA_MAN 13500 0.3 100 80 147 Alberto Errazuriz AERRAZUR 011.44.1344. 8/3/1987 SA_MAN 12000 0.3 100 80 148 Gerald Cambrault GCAMBRAU 011.44.1344. 8/4/1987 SA_MAN 11000 0.3 100 80 149 Eleni Zlotkey EZLOTKEY 011.44.1344. 8/5/1987 SA_MAN 10500 0.2 100 80 150 Peter Tucker PTUCKER 011.44.1344. 8/6/1987 SA_REP 10000 0.3 145 80 151 David Bernstein DBERNSTE 011.44.1344. 8/7/1987 SA_REP 9500 0.25 145 80 152 Peter Hall PHALL 011.44.1344. 8/8/1987 SA_REP 9000 0.25 145 80 153 Christophe Olsen COLSEN 011.44.1344. 8/9/1987 SA_REP 8000 0.2 145 80 154 Nanette Cambrault NCAMBRAU 011.44.1344. 8/10/1987 SA_REP 7500 0.2 145 80 155 Oliver Tuvault OTUVAULT 011.44.1344. 8/11/1987 SA_REP 7000 0.15 145 80 156 Janette King JKING 011.44.1345. 8/12/1987 SA_REP 10000 0.35 146 80 157 Patrick Sully PSULLY 011.44.1345. 8/13/1987 SA_REP 9500 0.35 146 80 158 Allan McEwen AMCEWEN 011.44.1345. 8/14/1987 SA_REP 9000 0.35 146 80 159 Lindsey Smith LSMITH 011.44.1345. 8/15/1987 SA_REP 8000 0.3 146 80 160 Louise Doran LDORAN 011.44.1345. 8/16/1987 SA_REP 7500 0.3 146 80 161 Sarath Sewall SSEWALL 011.44.1345. 8/17/1987 SA_REP 7000 0.25 146 80 162 Clara Vishney CVISHNEY 011.44.1346. 8/18/1987 SA_REP 10500 0.25 147 80 163 Danielle Greene DGREENE 011.44.1346. 8/19/1987 SA_REP 9500 0.15 147 80 164 Mattea Marvins MMARVINS 011.44.1346. 8/20/1987 SA_REP 7200 0.1 147 80 165 David Lee DLEE 011.44.1346. 8/21/1987 SA_REP 6800 0.1 147 80 166 Sundar Ande SANDE 011.44.1346. 8/22/1987 SA_REP 6400 0.1 147 80 167 Amit Banda ABANDA 011.44.1346. 8/23/1987 SA_REP 6200 0.1 147 80 168 Lisa Ozer LOZER 011.44.1343. 8/24/1987 SA_REP 11500 0.25 148 80 169 Harrison Bloom HBLOOM 011.44.1343. 8/25/1987 SA_REP 10000 0.2 148 80 170 Tayler Fox TFOX 011.44.1343. 8/26/1987 SA_REP 9600 0.2 148 80 171 William Smith WSMITH 011.44.1343. 8/27/1987 SA_REP 7400 0.15 148 80 172 Elizabeth Bates EBATES 011.44.1343. 8/28/1987 SA_REP 7300 0.15 148 80 173 Sundita Kumar SKUMAR 011.44.1343. 8/29/1987 SA_REP 6100 0.1 148 80 174 Ellen Abel EABEL 011.44.1644. 8/30/1987 SA_REP 11000 0.3 149 80 175 Alyssa Hutton AHUTTON 011.44.1644. 8/31/1987 SA_REP 8800 0.25 149 80 176 Jonathon Taylor JTAYLOR 011.44.1644. 9/1/1987 SA_REP 8600 0.2 149 80 177 Jack Livingston JLIVINGS 011.44.1644. 9/2/1987 SA_REP 8400 0.2 149 80 178 Kimberely Grant KGRANT 011.44.1644. 9/3/1987 SA_REP 7000 0.15 149 179 Charles Johnson CJOHNSON 011.44.1644. 9/4/1987 SA_REP 6200 0.1 149 80 180 Winston Taylor WTAYLOR 650.507.9876 9/5/1987 SH_CLERK 3200 120 50 181 Jean Fleaur JFLEAUR 650.507.9877 9/6/1987 SH_CLERK 3100 120 50 182 Martha Sullivan MSULLIVA 650.507.9878 9/7/1987 SH_CLERK 2500 120 50 183 Girard Geoni GGEONI 650.507.9879 9/8/1987 SH_CLERK 2800 120 50 184 Nandita Sarchand NSARCHAN 650.509.1876 9/9/1987 SH_CLERK 4200 121 50 185 Alexis Bull ABULL 650.509.2876 9/10/1987 SH_CLERK 4100 121 50 186 Julia Dellinger JDELLING 650.509.3876 9/11/1987 SH_CLERK 3400 121 50 187 Anthony Cabrio ACABRIO 650.509.4876 9/12/1987 SH_CLERK 3000 121 50 188 Kelly Chung KCHUNG 650.505.1876 9/13/1987 SH_CLERK 3800 122 50 189 Jennifer Dilly JDILLY 650.505.2876 9/14/1987 SH_CLERK 3600 122 50 190 Timothy Gates TGATES 650.505.3876 9/15/1987 SH_CLERK 2900 122 50 191 Randall Perkins RPERKINS 650.505.4876 9/16/1987 SH_CLERK 2500 122 50 192 Sarah Bell SBELL 650.501.1876 9/17/1987 SH_CLERK 4000 123 50 193 Britney Everett BEVERETT 650.501.2876 9/18/1987 SH_CLERK 3900 123 50 194 Samuel McCain SMCCAIN 650.501.3876 9/19/1987 SH_CLERK 3200 123 50 195 Vance Jones VJONES 650.501.4876 9/20/1987 SH_CLERK 2800 123 50 196 Alana Walsh AWALSH 650.507.9811 9/21/1987 SH_CLERK 3100 124 50 197 Kevin Feeney KFEENEY 650.507.9822 9/22/1987 SH_CLERK 3000 124 50 198 Donald OConnell DOCONNEL 650.507.9833 9/23/1987 SH_CLERK 2600 124 50 199 Douglas Grant DGRANT 650.507.9844 9/24/1987 SH_CLERK 2600 124 50 200 Jennifer Whalen JWHALEN 515.123.4444 9/25/1987 AD_ASST 4400 101 10 201 Michael Hartstein MHARTSTE 515.123.5555 9/26/1987 MK_MAN 13000 100 20 202 Pat Fay PFAY 603.123.6666 9/27/1987 MK_REP 6000 201 20 203 Susan Mavris SMAVRIS 515.123.7777 9/28/1987 HR_REP 6500 101 40 204 Hermann Baer HBAER 515.123.8888 9/29/1987 PR_REP 10000 101 70 205 Shelley Higgins SHIGGINS 515.123.8080 9/30/1987 AC_MGR 12000 101 110 206 William Gietz WGIETZ 515.123.8181 10/1/1987 AC_ACCOUNT 8300 205 110
SQL Code:
-- Selecting specific columns from the employees table and aliasing it as 'a'
SELECT employee_id, manager_id, first_name, last_name
-- Filtering employees based on the NOT EXISTS condition
FROM employees a
-- Specifying the condition for filtering using the NOT EXISTS keyword
WHERE NOT EXISTS (
-- Selecting employee_id from employees table and aliasing it as 'b'
SELECT employee_id
-- Filtering employees based on manager_id
FROM employees b
-- Specifying the condition for filtering
WHERE b.manager_id = a.employee_id
);
Explanation:
- This SQL query retrieves specific columns from the "employees" table, aliasing it as 'a'.
- It filters the employees based on the non-existence of records in a correlated subquery.
- The subquery selects the employee_id from the "employees" table, aliasing it as 'b'.
- It filters the employees based on whether there does not exist an employee with a manager_id that matches the employee_id from the outer query.
- The main query then selects employees where the subquery returns no records, meaning it selects employees who do not manage other employees.
Visual Presentation:
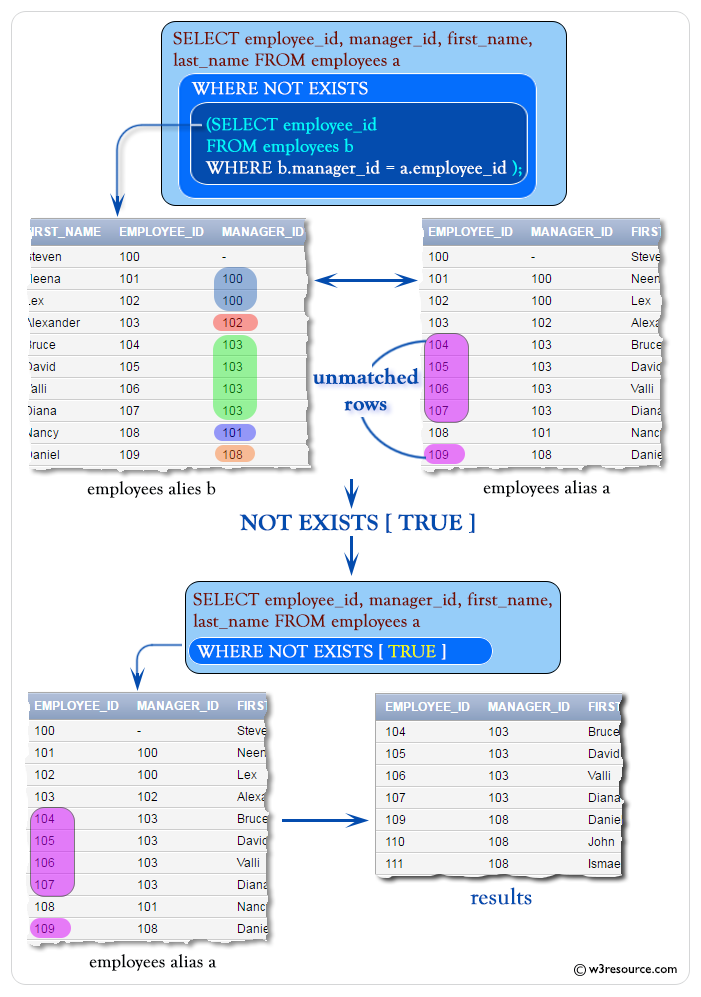
Output:
EMPLOYEE_ID MANAGER_ID FIRST_NAME LAST_NAME ----------- ---------- -------------------- -------------- 104 103 Bruce Ernst 105 103 David Austin 106 103 Valli Pataballa 107 103 Diana Lorentz 109 108 Daniel Faviet 110 108 John Chen 111 108 Ismael Sciarra 112 108 Jose Manuel Urman 113 108 Luis Popp 115 114 Alexander Khoo 116 114 Shelli Baida 117 114 Sigal Tobias 118 114 Guy Himuro 119 114 Karen Colmenares 125 120 Julia Nayer 126 120 Irene Mikkilineni 127 120 James Landry 128 120 Steven Markle 129 121 Laura Bissot 130 121 Mozhe Atkinson 131 121 James Marlow ........ .......
Example: Performance Consideration with Correlated Subqueries
Find orders where the order amount exceeds the average order amount handled by the same agent
SQL Code:
-- Selects order number, order amount, and agent code from the orders table aliased as 'o'.
-- Filters orders where the order amount is greater than the average order amount handled by the same agent.
SELECT ord_num, ord_amount, agent_code
FROM orders o
WHERE ord_amount > (
-- Subquery: Calculates the average order amount for orders handled by the agent of the current row in the outer query.
SELECT AVG(ord_amount)
FROM orders
WHERE agent_code = o.agent_code
);
Explanation:
- This SQL query retrieves ord_num, ord_amount, and agent_code from the orders table, aliased as 'o'.
- It filters the results to include only those orders where the ord_amount is greater than the average ord_amount handled by the same agent_code.
- The subquery calculates the average ord_amount for orders associated with the agent_code of each row (o.agent_code) in the outer query.
- This demonstrates the use of a correlated subquery to compare values within the same table based on a condition from the outer query.
Real-World Use Case of Correlated Subquery
List employees who have been with the company longer than any of their direct reports
SQL Code:
-- Selects employee_id, first_name, and last_name from the employees table aliased as 'e'.
-- Filters employees where the hire date is greater than all hire dates of employees managed by them.
SELECT employee_id, first_name, last_name
FROM employees e
WHERE hire_date > ALL (
-- Subquery: Retrieves hire dates of employees who report to the manager of the current row in the outer query.
SELECT hire_date
FROM employees
WHERE manager_id = e.employee_id
);
Explanation:
- This SQL query selects employee_id, first_name, and last_name from the employees table, aliased as 'e'.
- It filters the results to include only those employees whose hire_date is greater than the hire dates of all employees who report to them.
- The subquery retrieves hire_date values from employees who have the same manager_id as the employee_id of the current row (e.employee_id) in the outer query.
- This query demonstrates the use of the ALL operator with a correlated subquery to compare values across related rows in the same table based on a condition from the outer query.
Frequently Asked Questions (FAQ) - SQL Correlated Subqueries
1. What are SQL Correlated Subqueries?
SQL Correlated Subqueries are used to select data from a table referenced in the outer query. The subquery in a correlated subquery is related to the outer query, hence the term "correlated". Each execution of the subquery in the correlated subquery depends on the row processed by the outer query.
2. How do Correlated Subqueries differ from Uncorrelated Subqueries?
In SQL, an uncorrelated subquery executes independently and provides a single value or set of values to the outer query. In contrast, a correlated subquery references columns from the outer query and executes once for each row processed by the outer query.
3. What is a table alias (correlation name) used for in Correlated Subqueries?
A table alias, or correlation name, specifies which table reference is to be used in the context of a correlated subquery. It helps differentiate columns from the outer query and the subquery when both reference the same table.
4. When should I use SQL Correlated Subqueries?
Correlated subqueries are useful when you need to filter rows based on conditions evaluated from related rows in another table. They are typically used in scenarios where the filtering condition depends on values from the current row being processed by the outer query.
5. What are some keywords commonly used with Correlated Subqueries?
Commonly used keywords include EXISTS and NOT EXISTS, which help to check for the existence or non-existence of rows returned by a subquery in the context of a correlated subquery.
Check out our 1000+ SQL Exercises with solution and explanation to improve your skills.
Previous: Multiple Row and Column Subqueries
Next: Nested subqueries
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics