Python JSON: Convert Python object to JSON data
Python JSON: Exercise-2 with Solution
Write a Python program to convert Python object to JSON data.
Sample Solution:-
Python Code:
import json
# a Python object (dict):
python_obj = {
"name": "David",
"class":"I",
"age": 6
}
print(type(python_obj))
# convert into JSON:
j_data = json.dumps(python_obj)
# result is a JSON string:
print(j_data)
Output:
<class 'dict'> {"name": "David", "class": "I", "age": 6}
Flowchart:
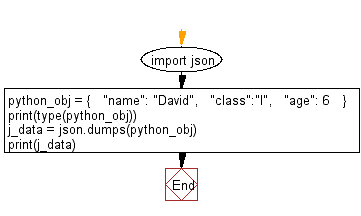
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to convert JSON data to Python object.
Next: Write a Python program to convert Python objects into JSON strings. Print all the values.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/python-exercises/python-json-exercise-2.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics