Python JSON: Convert JSON data to Python object
Python JSON: Exercise-1 with Solution
Write a Python program to convert JSON data to Python object.
Sample Solution:-
Python Code:
import json
json_obj = '{ "Name":"David", "Class":"I", "Age":6 }'
python_obj = json.loads(json_obj)
print("\nJSON data:")
print(python_obj)
print("\nName: ",python_obj["Name"])
print("Class: ",python_obj["Class"])
print("Age: ",python_obj["Age"])
Output:
JSON data: {'Name': 'David', 'Class': 'I', 'Age': 6} Name: David Class: I Age: 6
Flowchart:
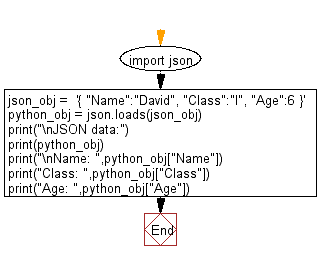
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Python JSON Exercises Home.
Next: Write a Python program to convert Python object to JSON data.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/python-exercises/python-json-exercise-1.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics