PHP: array_chunk() function
PHP: Split an array into chunks
The array_chunk() function is used to split an array into arrays with size elements. The last chunk may contain less than size elements.
Version:
(PHP 4 and above)
Syntax:
array_chunk(input_array, size, preserve_keys)
Parameters:
Name | Description | Required / Optional |
Type |
---|---|---|---|
input_array | Specifies the array to split. | Required | Array |
size | The size of each chunk (i.e. the number of elements) | Required | Integer |
preserve_keys | If we set preserve_keys as TRUE, array_chunk function preserves the original array keys. The default is FALSE which will reindex the chunk numerically. | Optional | Boolean |
Return value:
A multidimensional numerically indexed array.
Value Type: Array
Example - 1:
<?php
$input_array = array('name1', 'name2', 'name3', 'name4', 'name5');
print_r(array_chunk($input_array,2));
?>
Output:
Array ( [0] => Array ( [0] => name1 [1] => name2 ) [1] => Array ( [0] => name3 [1] => name4) [2] => Array ([0] => name5 ))
Pictorial Presentation:
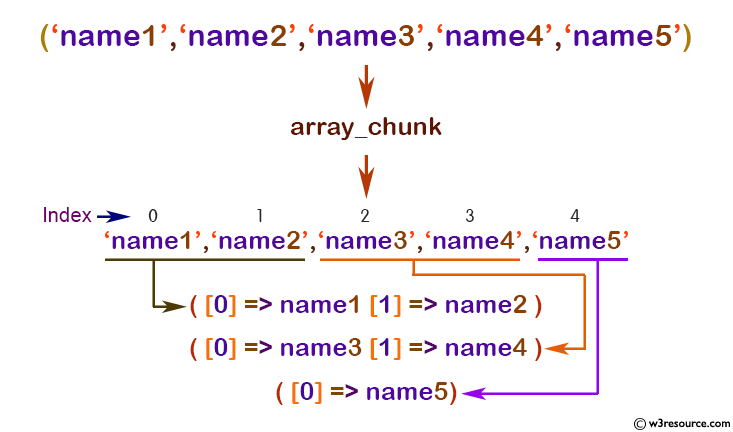
View the example in the browser
Example - 2:
In the following example, we set preserve_keys as TRUE, therefore, array_chunk function preserves the original array keys.
<?php
$input_array = array('name1', 'name2', 'name3', 'name4', 'name5');
print_r(array_chunk($input_array,2,true));
?>
Output:
Array ( [0] => Array ( [0] => name1 [1] => name2 ) [1] => Array ( [2] => name3 [3] => name4 ) [2] => Array ( [4] => name5 ) )
View the example in the browser
Practice here online :
See also
Previous: array_change_ key_case
Next: array_combine