C#: Check whether a given array of integers contains a 3 or a 5
Check If Array Contains 3 or 5
Write a C# Sharp program to check whether a given array of integers contains a 3 or a 5.
Visual Presentation:
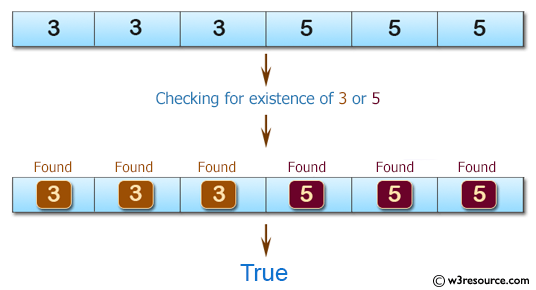
Sample Solution:
C# Sharp Code:
using System; // Importing the System namespace
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Outputting the result of the 'test' method with different integer arrays as arguments
Console.WriteLine(test(new[] { 5, 5, 5, 5, 5 })); // Testing the method with an array containing all 5s
Console.WriteLine(test(new[] { 3, 3, 3, 3 })); // Testing the method with an array containing all 3s
Console.WriteLine(test(new[] { 3, 3, 3, 5, 5, 5 })); // Testing the method with an array containing both 3s and 5s
Console.WriteLine(test(new[] { 1, 6, 8, 10 })); // Testing the method with an array containing values other than 3 or 5
}
// Method to check if all elements in the array are either 3 or 5
static bool test(int[] nums)
{
for (int i = 0; i < nums.Length; i++)
{
if (nums[i] != 3 && nums[i] != 5) return false; // If any element is not 3 or 5, return false
}
return true; // Return true if all elements in the array are either 3 or 5
}
}
}
Sample Output:
True True True False
Flowchart:
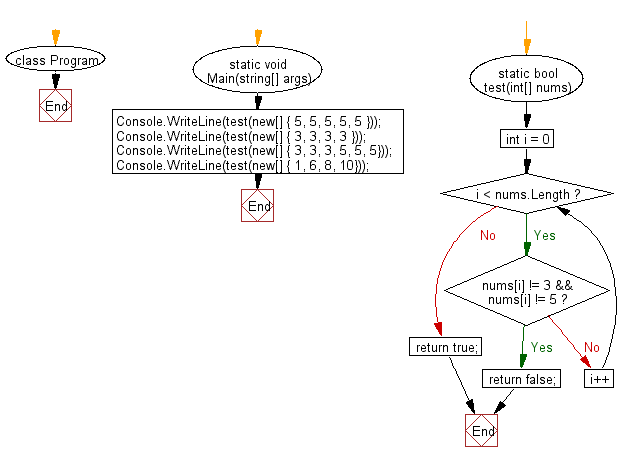
Go to:
PREV : Check If 3's Outnumber 5's.
NEXT : Check If Array Contains No 3 or 5.
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.