C#: Check whether a given array of integers contains no 3 or a 5
Check If Array Contains No 3 or 5
Write a C# Sharp program to check if a given array of integers contains no 3 or 5.
Visual Presentation:
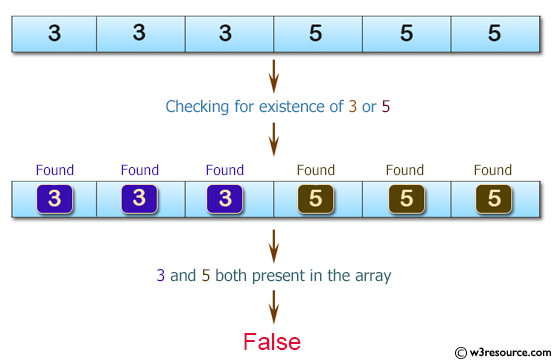
Sample Solution:
C# Sharp Code:
using System; // Importing the System namespace
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Outputting the result of the 'test' method with different integer arrays as arguments
Console.WriteLine(test(new[] { 5, 5, 5, 5, 5 })); // Testing the method with an array containing all 5s
Console.WriteLine(test(new[] { 3, 3, 3, 3 })); // Testing the method with an array containing all 3s
Console.WriteLine(test(new[] { 3, 3, 3, 5, 5, 5 })); // Testing the method with an array containing both 3s and 5s
Console.WriteLine(test(new[] { 1, 6, 8, 10 })); // Testing the method with an array containing values other than 3 or 5
}
// Method to check if an array contains both 3 and 5 but not both
static bool test(int[] nums)
{
var three = false; // Variable to track if number 3 is present
var five = false; // Variable to track if number 5 is present
for (int i = 0; i < nums.Length; i++)
{
if (nums[i] == 3) {three = true;} // Setting 'three' to true if 3 is found
if (nums[i] == 5) {five = true;} // Setting 'five' to true if 5 is found
if (three && five) return false; // If both 3 and 5 are present, return false
}
return true; // Return true if either 3 or 5 (but not both) is present in the array
}
}
}
Sample Output:
True True False True
Flowchart:
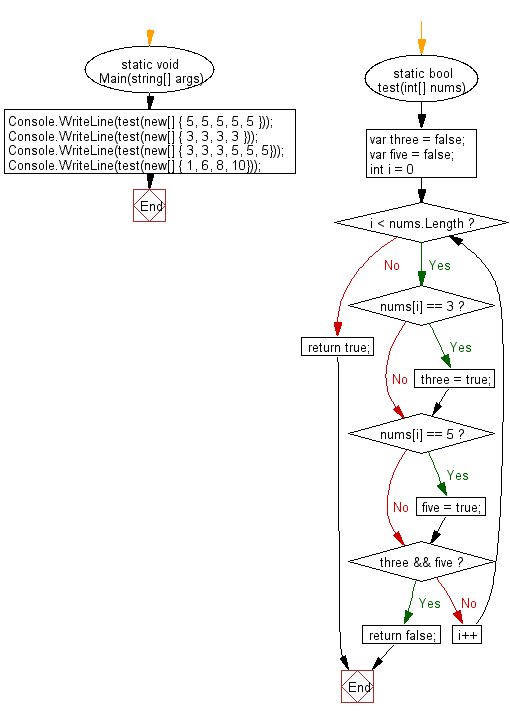
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to check whether a given array of integers contains a 3 or a 5
Next: Write a C# Sharp program to check whether an array of integers contains a 3 next to a 3 or a 5 next to a 5 or both.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics