C#: Check whether the number of 3's is greater than the number of 5's
Check If 3's Outnumber 5's
Write a C# Sharp program to check whether the number of 3's is greater than the number of 5's.
Visual Presentation:
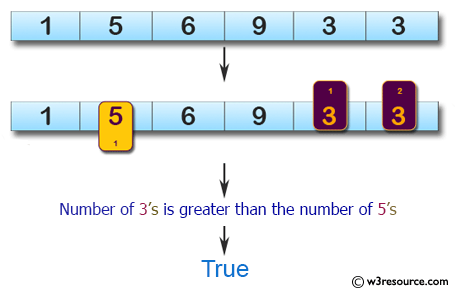
Sample Solution:
C# Sharp Code:
using System; // Importing the System namespace
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Outputting the result of the 'test' method with different integer arrays as arguments
Console.WriteLine(test(new[] { 1, 5, 6, 9, 3, 3 })); // Testing the method with an array
Console.WriteLine(test(new[] { 1, 5, 5, 5, 10, 17 })); // Testing the method with another array
Console.WriteLine(test(new[] { 1, 3, 3, 5, 5, 5 })); // Testing the method with a third array
}
// Method to check if the count of occurrences of the number 3 is greater than the count of occurrences of the number 5 in the array
static bool test(int[] nums)
{
int no_3 = 0, no_5 = 0;
// Looping through the array to count occurrences of numbers 3 and 5
for (int i = 0; i < nums.Length; i++)
{
if (nums[i] == 3) no_3++; // Incrementing no_3 when encountering the number 3
if (nums[i] == 5) no_5++; // Incrementing no_5 when encountering the number 5
}
// Returning true if the count of occurrences of the number 3 is greater than the count of occurrences of the number 5, else false
return no_3 > no_5;
}
}
}
Sample Output:
True False False
Flowchart:
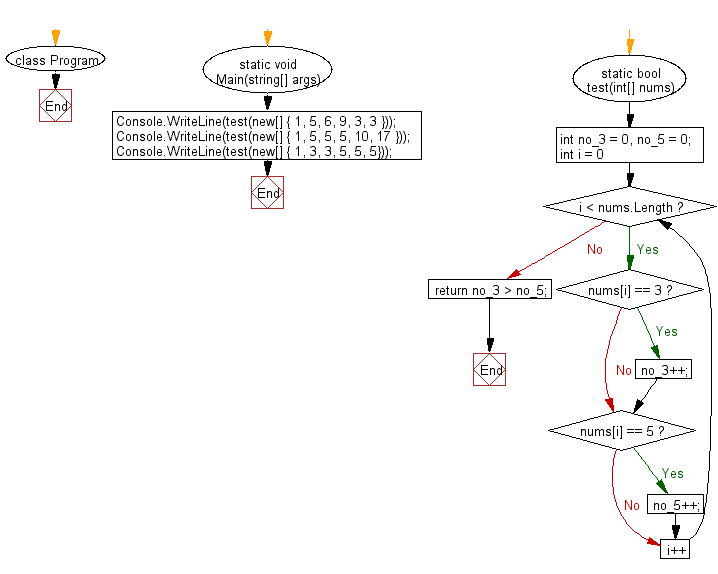
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to check whether the sum of all 5' in the array exactly 15 in a given array of integers.
Next: Write a C# Sharp program to check whether a given array of integers contains a 3 or a 5.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.