C#: Compute the sum of values in a given array of integers except the number 17
Sum Excluding 17
Write a C# Sharp program to compute the sum of values in a given array of integers except 17. Return 0 if the given array has no integers.
Visual Presentation:
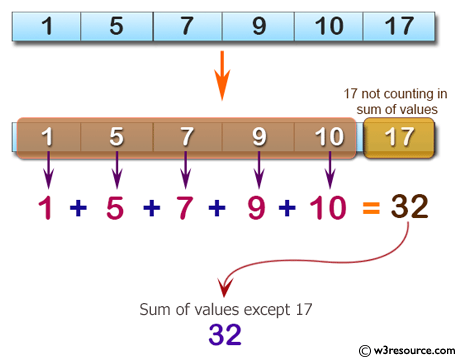
Sample Solution:-
C# Sharp Code:
using System; // Importing the System namespace
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Displaying a message indicating the purpose of the output
Console.WriteLine("Sum of values in the array of integers except the number 17: ");
// Outputting the result of the 'test' method with an integer array as an argument
Console.WriteLine(test(new[] { 1, 5, 7, 9, 10, 17 }));
}
// Method to calculate the sum of values in the array excluding the number 17
static int test(int[] nums)
{
int sum = 0; // Initializing a variable to hold the sum of values in the array
// Looping through each element of the array
for (int i = 0; i < nums.Length; i++)
{
// Checking if the current element is not equal to 17
if (nums[i] != 17)
{
sum += nums[i]; // Adding the current element to the sum if it's not 17
}
}
return sum; // Returning the total sum of values in the array excluding 17
}
}
}
Sample Output:
Sum of values in the array of integers except the number 17: 32
Flowchart:
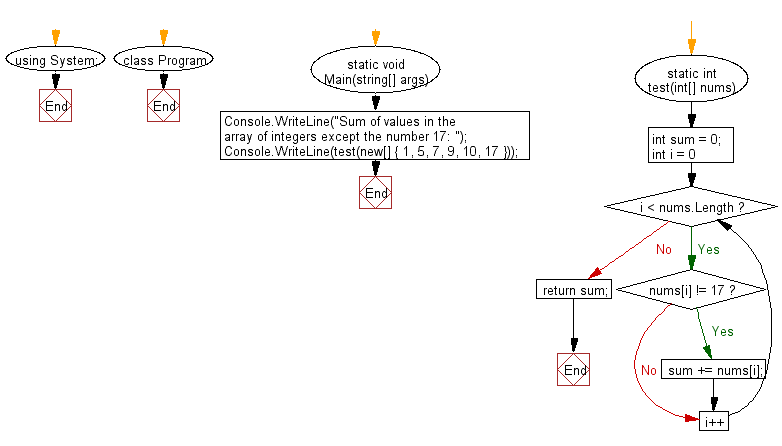
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to compute the difference between the largest and smallest values in a gvien array of integers and length one or more.
Next: Write a C# Sharp program to compute the sum of the numbers in a given array except those numbers starting with 5 followed by at least one 6. Return 0 if the given array has no integer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.