C#: Compute the sum of the numbers in a given array except those numbers starting with 5 followed by at least one 6
Sum Excluding Numbers Starting with 5 and 6
Write a C# Sharp program to compute the sum of the numbers in a given array except those that begin with 5 followed by at least one 6. Return 0 if the given array has no integers.
Visual Presentation:
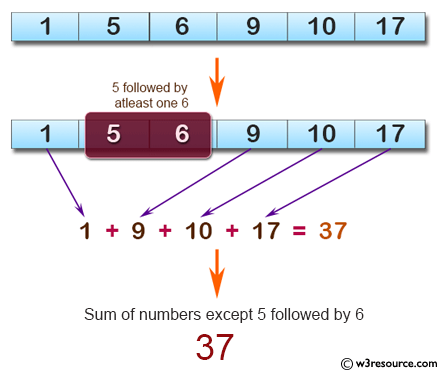
Sample Solution:-
C# Sharp Code:
using System; // Importing the System namespace
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Displaying a message indicating the purpose of the output
Console.WriteLine("Sum of the numbers of the said array except those numbers starting with 5 followed by at least one 6: ");
// Outputting the results of the 'test' method with various integer arrays as arguments
Console.WriteLine(test(new[] { 5, 6, 1, 5, 6, 9, 10, 17, 5, 6 }));
Console.WriteLine(test(new[] { 5, 6, 1, 5, 6, 9, 10, 17 }));
Console.WriteLine(test(new[] { 1, 5, 6, 9, 10, 17, 5, 6 }));
Console.WriteLine(test(new[] { 1, 5, 9, 10, 17, 5, 6 }));
Console.WriteLine(test(new[] { 1, 5, 9, 10, 17, 5}));
}
// Method to calculate the sum of numbers in the array, excluding those starting with 5 followed by at least one 6
static int test(int[] nums)
{
int sum = 0; // Initializing a variable to hold the sum of numbers in the array
int inSection = 0; // Variable to track if the code is within a specific section
int flag = 0; // Flag to identify the start of the section
// Looping through each element of the array
for (int i = 0; i < nums.Length; i++)
{
inSection = 0; // Resetting the section flag at the beginning of each iteration
// Checking if the current element is 5
if (nums[i] == 5)
{
inSection = 0; // Resetting the inSection flag
flag = 1; // Setting the flag to indicate the start of the section
}
// Checking if the current element is 6 and not in the section
if (inSection == 0 && nums[i] == 6)
{
// Checking if the flag indicates the start of the section
if (flag == 1)
{
sum = sum - 5; // Adjusting the sum by subtracting 5 (to exclude 5 from the sum)
inSection = 1; // Marking the section
}
flag = 0; // Resetting the flag
}
// Checking if the code is not in the section, then adding the number to the sum
if (inSection == 0)
{
sum += nums[i];
}
}
return sum; // Returning the total sum of numbers in the array excluding the specified pattern
}
}
}
Sample Output:
Sum of the numbers of the said array except those numbers starting with 5 followed by at least one 6: 37 37 37 42 47
Flowchart:
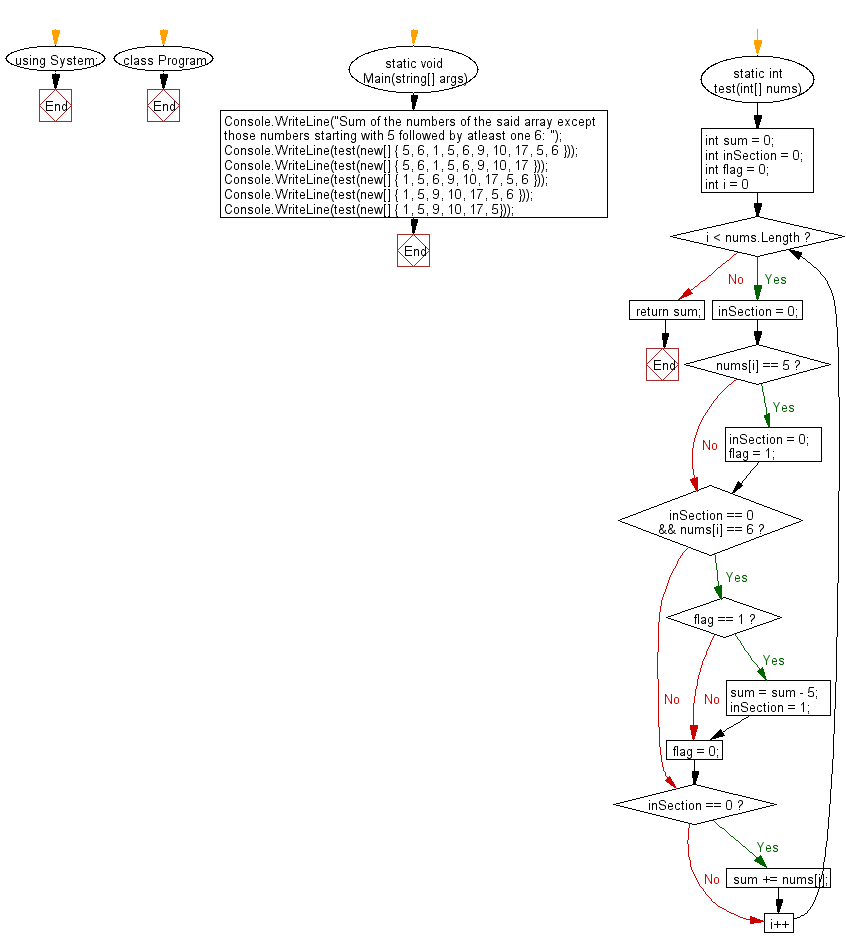
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to compute the sum of values in a given array of integers except the number 17. Return 0 if the given array has no integer.
Next: Write a C# Sharp program to check if a given array of integers contains 5 next to a 5 somewhere.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics