C#: Compute the difference between the largest and smallest values in a given array of integers of length one or more
Difference Between Largest and Smallest Values
Write a C# Sharp program to compute the difference between the largest and smallest values in a given array of integers and lengths of one or more.
Visual Presentation:
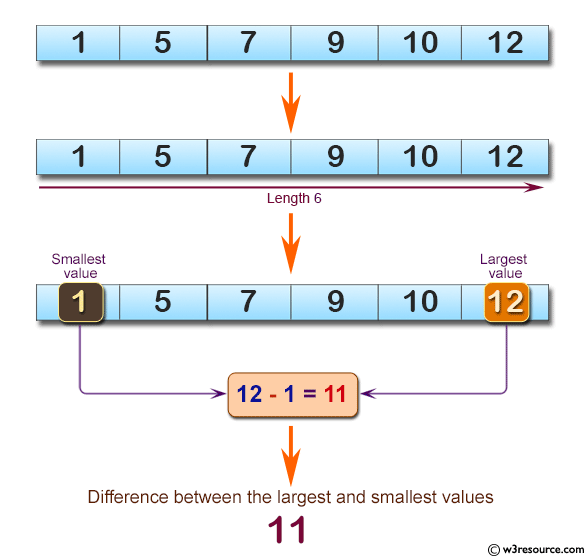
Sample Solution:-
C# Sharp Code:
using System; // Importing the System namespace
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Displaying a message indicating the purpose of the output
Console.WriteLine("Difference between the largest and smallest values: ");
// Outputting the result of the 'test' method with an integer array as an argument
Console.WriteLine(test(new[] { 1, 5, 7, 9, 10, 12 }));
}
// Method to calculate the difference between the largest and smallest values in the given array
static int test(int[] nums)
{
int small_num = 0, biggest_num = 0; // Initializing variables for the smallest and largest numbers
// Checking if the array has elements to avoid accessing empty arrays
if (nums.Length > 0)
{
small_num = biggest_num = nums[0]; // Assigning the first element as the initial smallest and largest numbers
}
// Looping through the array to find the smallest and largest numbers
for (int i = 1; i < nums.Length; i++)
{
small_num = Math.Min(small_num, nums[i]); // Updating the smallest number found so far
biggest_num = Math.Max(biggest_num, nums[i]); // Updating the largest number found so far
}
return biggest_num - small_num; // Returning the difference between the largest and smallest numbers
}
}
}
Sample Output:
Difference between the largest and smallest values: 11
Flowchart:
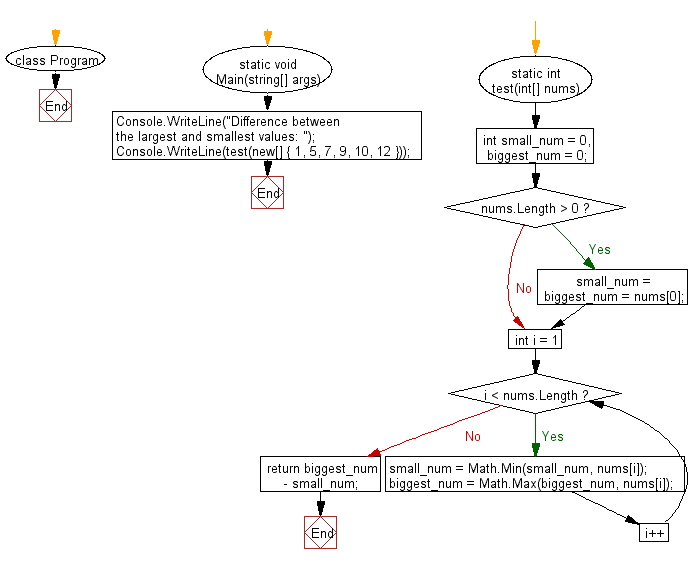
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to count even number of elements in a given array of integers.
Next: Write a C# Sharp program to compute the sum of values in a given array of integers except the number 17. Return 0 if the given array has no integer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics