Python: Check if the letters of a given string can be rearranged so that two characters that are adjacent to each other are different
15. Rearrange String (No Adjacent Duplicates)
Write a Python program to check if the letters in a given string can be rearranged. This is to make sure that two characters that are adjacent to each other are different using the heap queue algorithm.
Note:
If there is no output return the empty string.
Sample Solution:
Python Code:
import heapq
from collections import Counter
def reorganizeString(S):
ctr = Counter(S)
heap = [(-value, key) for key, value in ctr.items()]
heapq.heapify(heap)
if (-heap[0][0]) * 2 > len(S) + 1:
return ""
ans = []
while len(heap) >= 2:
nct1, char1 = heapq.heappop(heap)
nct2, char2 = heapq.heappop(heap)
ans.extend([char1, char2])
if nct1 + 1: heapq.heappush(heap, (nct1 + 1, char1))
if nct2 + 1: heapq.heappush(heap, (nct2 + 1, char2))
return "".join(ans) + (heap[0][1] if heap else "")
print(reorganizeString("aab"))
print(reorganizeString("abc"))
print(reorganizeString("aabb"))
print(reorganizeString("abccdd"))
Sample Output:
aba abc abab cdabcd
Flowchart:
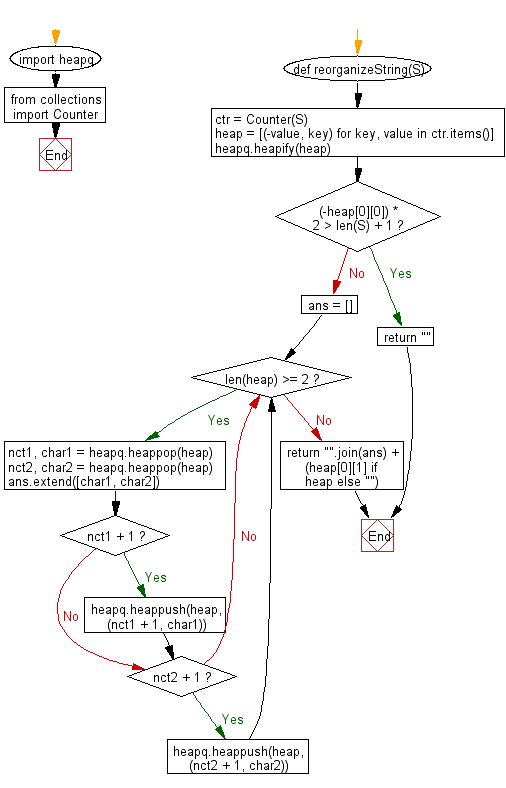
For more Practice: Solve these Related Problems:
- Write a Python program to determine if the letters of a string can be rearranged so that no two identical letters are adjacent using a max-heap.
- Write a Python script to rearrange a string using heapq so that identical characters are separated, and then print the rearranged string.
- Write a Python function to check if a valid rearrangement exists for a string using a heap-based algorithm and return a boolean value.
- Write a Python program to use a heap to rearrange characters in a string such that no two adjacent characters are the same, handling cases with high frequency letters.
Go to:
Previous: Write a Python program to get the k most frequent elements from a given non-empty list of words using Heap queue algorithm.
Next: Write a Python program which add integer numbers from the data stream to a heapq and compute the median of all elements.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.