Python: Get the k most frequent elements from a given non-empty list of words using Heap queue algorithm
Write a Python program to get the k most frequent elements from a given non-empty list of words using the heap queue algorithm
Note:
Range of k, 1 ≤ k ≤ number of unique elements and input words contain only lowercase letters.
Sample Solution:
Python Code:
import heapq
from collections import Counter
class Solution:
def top_K_Frequent(self, words, k):
"""
:type words: List[str]
:type k: int
:return type: List[str]
"""
ctr = Counter(words)
heap = [(-ctr[word], word) for word in ctr]
heapq.heapify(heap)
return [heapq.heappop(heap)[1] for _ in range(k)]
if __name__ == '__main__':
words = ["a", "abc", "abcdef", "a", "abcd", "abcd", "abc", "abcdefg"]
k = 3
s = Solution()
print("3 most frequent elements:")
print(s.top_K_Frequent(words, k))
Sample Output:
3 most frequent elements: ['a', 'abc', 'abcd']
Flowchart:
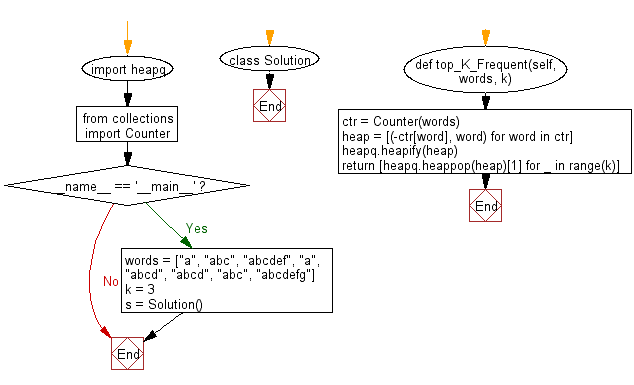
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to find the nth super ugly number from a given prime list of size k using Heap queue algorithm.
Next: Write a Python program to check if the letters of a given string can be rearranged so that two characters that are adjacent to each other are different using Heap queue algorithm.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics