Python: Merge multiple sorted inputs into a single sorted iterator (over the sorted values) using Heap queue algorithm
11. Merge Sorted Inputs
Write a Python program that merges multiple sorted inputs into a single sorted iterator (over the sorted values) using the heap queue algorithm.
Sample Solution:
Python Code:
import heapq
num1 = [25, 24, 15, 4, 5, 29, 110]
num2 = [19, 20, 11, 56, 25, 233, 154]
num3 = [24, 26, 54, 48]
num1 = sorted(num1)
num2 = sorted(num2)
num3 = sorted(num3)
result = heapq.merge(num1, num2, num3)
print(list(result))
Sample Output:
[4, 5, 11, 15, 19, 20, 24, 24, 25, 25, 26, 29, 48, 54, 56, 110, 154, 233]
Flowchart:
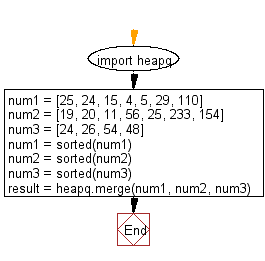
For more Practice: Solve these Related Problems:
- Write a Python program to merge multiple sorted lists into a single sorted iterator using heapq.merge and then output the merged sequence.
- Write a Python script to combine several sorted arrays into one sorted list by leveraging heapq.merge and print the final result.
- Write a Python program to implement a function that merges sorted iterators from different sources using heapq and returns the combined sorted iterator.
- Write a Python function to merge sorted data from multiple files using heapq.merge and then display the merged output.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to get the n expensive and cheap price items from a given dataset using Heap queue algorithm.
Next: Given a n x n matrix where each of the rows and columns are sorted in ascending order, write a Python program to find the kth smallest element in the matrix.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.