PHP: Comparison Operators
Description
In PHP, comparison operators take simple values (numbers or strings) as arguments and evaluate to either TRUE or FALSE.
Here is a list of comparison operators.
Operator | Name | Example | Result |
---|---|---|---|
= = | Equal | $x == $y | TRUE if $x is exactly equal to $y |
= = = | Identical | $x === $y | TRUE if $x is exactly equal to $y, and they are of the same type. |
!= | Not equal | $x != $y | TRUE if $x is exactly not equal to $y. |
<> | Not equal | $x <> $y | TRUE if $x is exactly not equal to $y. |
!== | Not identical | $x !== $y | TRUE if $x is not equal to $y, or they are not of the same type. |
< | Less than | $x < $y | TRUE if $x (left-hand argument) is strictly less than $y (right-hand argument). |
> | Greater than | $x > $y | TRUE if $x (left hand argument) is strictly greater than $y (right hand argument). |
<= | Less than or equal to | $x <= $y | TRUE if $x (left hand argument) is less than or equal to $y (right hand argument). |
>= | Greater than or equal to | $x >= $y | TRUE if $x is greater than or equal to $y. |
Pictorial presentation of Equal (==) operator
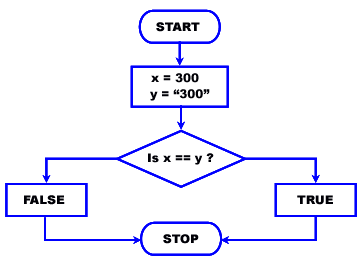
Test Equal (==) operator
The following php codes return true though the type of $x and $y are not equal (the first one is integer type and the second one is character type) but their values are equal.
<?php
$x = 300;
$y = "300";
var_dump($x == $y);
?>
Output :
bool(true)
View the example in the browser
Pictorial presentation of Strict equal (===) operator
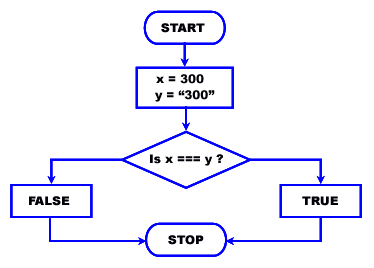
Test Strict equal (===) operator
The following php codes returns false as the strict equal operator will compare both value and type of $x and $y.
<?php
$x = 300;
$y = "300";
var_dump($x === $y);
?>
Output :
bool(false)
View the example in the browser
Pictorial presentation of Not equal(!=)/(<>) operator
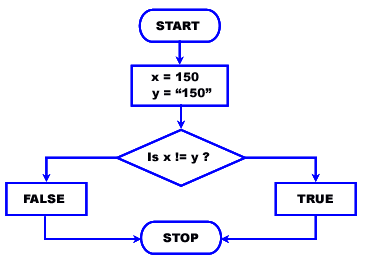
Test Not equal (!=) / (<>) operator
The following php codes return false though the type of $x and $y are not equal (the first one is integer type and the second one is character type) but their values are equal.
<?php
$x = 150;
$y = "150";
var_dump($x != $y);
?>
Output of the example
bool(false)
View the example in the browser
Test Not identical (!==) operator
The following php codes return true though their values are equal but the type of $x and $y are not equal (the first one is integer type and the second one is character type).
<?php
$x = 150;
$y = "150";
var_dump($x !== $y);
?>
Output :
bool(true)
View the example in the browser
Pictorial presentation of Greater than(>) operator
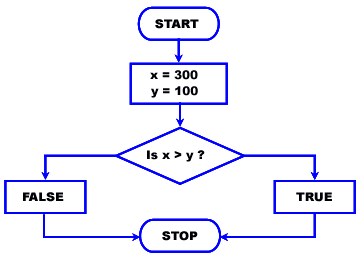
Test Greater than(>) operator
The following php code return true as the value of $x is greater than $y.
<?php
$x = 300;
$y = 100;
var_dump($x>$y);
?>
Output :
bool(true)
View the example in the browser
Pictorial presentation of Greater than or equal (>=)
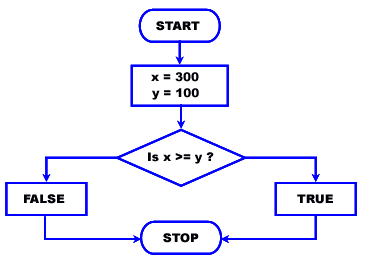
Test Greater than or equal (>=) operator
The following php codes return true as the value of $x is equal to $y.
<?php
$x = 300;
$y = 100;
var_dump($x>=$y);
?>
Output :
bool(true)
View the example in the browser
Pictorial presentation of Less than (<) operator
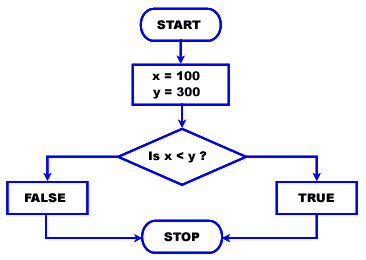
Test Less than (<) operator
The following php codes return true as the value of $x is less than $y.
<?php
$x = 100;
$y = 300;
var_dump($x<$y);
?>
Output :
bool(true)
View the example in the browser
Pictorial presentation of Less than or equal (<=) operator
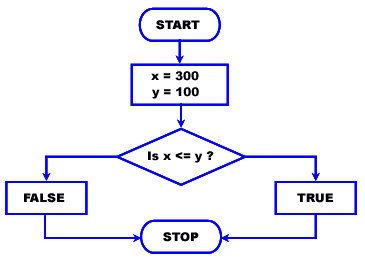
Test Less than or equal (<=) operator
The following PHP codes return false as the value of $x is greater than $y.
<?php
$x = 300;
$y = 100;
var_dump($x<=$y);
?>
Output :
bool(false)
View the example in the browser
See also
Previous: Arithmetic Operators
Next: Logical Operators
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/php/operators/comparison-operators.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics