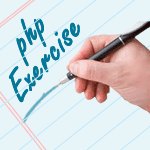
PHP arrays [15 exercises with solution]
1. $color = array('white', 'green', 'red', 'blue', 'black');
Write a script which will display the following string - Go to the editor
"The memory of that scene for me is like a frame of film forever frozen at that moment: the red carpet, the green lawn, the white house, the leaden sky. The new president and his first lady. - Richard M. Nixon"
and the words 'red', 'green' and 'white' will come from $color.
<?php $color = array('white', 'green', 'red', 'blue', 'black'); echo "The memory of that scene for me is like a frame of film forever frozen at that moment: the $color[2] carpet, the $color[1] lawn, the $color[0] house, the leaden sky. The new president and his first lady. - Richard M. Nixon" ?>
2. $color = array('white', 'green', 'red'') Go to the editor
Write a PHP script which will display the colors in the following way :
Output :
white, green, red,
<?php $color = array('white', 'green', 'red'); foreach ($color as $c) { echo "$c, "; } sort($color); echo "<ul>"; foreach ($color as $y) { echo "<li>$y</li>"; } echo "</ul>"; ?>
3. $ceu = array( "Italy"=>"Rome", "Luxembourg"=>"Luxembourg", "Belgium"=> "Brussels", "Denmark"=>"Copenhagen", "Finland"=>"Helsinki", "France" => "Paris", "Slovakia"=>"Bratislava", "Slovenia"=>"Ljubljana", "Germany" => "Berlin", "Greece" => "Athens", "Ireland"=>"Dublin", "Netherlands"=>"Amsterdam", "Portugal"=>"Lisbon", "Spain"=>"Madrid", "Sweden"=>"Stockholm", "United Kingdom"=>"London", "Cyprus"=>"Nicosia", "Lithuania"=>"Vilnius", "Czech Republic"=>"Prague", "Estonia"=>"Tallin", "Hungary"=>"Budapest", "Latvia"=>"Riga", "Malta"=>"Valetta", "Austria" => "Vienna", "Poland"=>"Warsaw") ;
Create a PHP script which display the capital and country name from the above array $ceu. Sort the list by the name of the country. Go to the editor
Sample Output :
The capital of Netherlands is Amsterdam
The capital of Greece is Athens
The capital of Germany is Berlin
- - - - - - - - - - - - - - - - - - - - - - - - -
- - - - - - - - - - - - - - - - - - - - - - - - -
<?php $ceu = array( "Italy"=>"Rome", "Luxembourg"=>"Luxembourg", "Belgium"=> "Brussels", "Denmark"=>"Copenhagen", "Finland"=>"Helsinki", "France" => "Paris", "Slovakia"=>"Bratislava", "Slovenia"=>"Ljubljana", "Germany" => "Berlin", "Greece" => "Athens", "Ireland"=>"Dublin", "Netherlands"=>"Amsterdam", "Portugal"=>"Lisbon", "Spain"=>"Madrid", "Sweden"=>"Stockholm", "United Kingdom"=>"London", "Cyprus"=>"Nicosia", "Lithuania"=>"Vilnius", "Czech Republic"=>"Prague", "Estonia"=>"Tallin", "Hungary"=>"Budapest", "Latvia"=>"Riga", "Malta"=>"Valetta", "Austria" => "Vienna", "Poland"=>"Warsaw") ; asort($ceu) ; foreach($ceu as $country => $capital) { echo "The capital of $country is $capital <br />" ; } ?>
4. $x = array(1, 2, 3, 4, 5);
Delete an element from the above PHP array. After deleting the element, integer keys must be normalized. Go to the editor
Sample Output :
array(5) { [0]=> int(1) [1]=> int(2) [2]=> int(3) [3]=> int(4) [4]=> int(5) }
array(4) { [0]=> int(1) [1]=> int(2) [2]=> int(3) [3]=> int(5) }
<?php $x = array(1, 2, 3, 4, 5); var_dump($x); unset($x[3]); $x = array_values($x); echo '
'; var_dump($x); ?>
5. $color = array(4 => 'white', 6 => 'green', 11=> 'red');
Write a PHP script to to get the first element of the above array. Go to the editor
Expected result : white
<?php $color = array(4 => 'white', 6 => 'green', 11=> 'red'); echo reset($color); ?>
6. Write a PHP script which decode the following JSON string. Go to the editor
Sample JSON code :
{"Title": "The Cuckoos Calling",
"Author": "Robert Galbraith",
"Detail": {
"Publisher": "Little Brown"
}}
Expected Output :
Title : The Cuckoos Calling
Author : Robert Galbraith
Publisher : Little Brown
<?php function w3rfunction($value,$key) { echo "$key : $value<br />"; } $a = '{"Title": "The Cuckoos Calling", "Author": "Robert Galbraith", "Detail": { "Publisher": "Little Brown" } }'; $j1 = json_decode($a,true); array_walk_recursive($j1,"w3rfunction"); ?>
7. Write a PHP script that insert a new item in an array on any position. Go to the editor
Expected Output :
Original array :
1 2 3 4 5
After inserting '$' the array is :
1 2 3 $ 4 5
<?php $original = array( '1','2','3','4','5' ); echo 'Original array : <br />'; foreach ($original as $x) {echo "$x ";} $inserted = '$'; array_splice( $original, 3, 0, $inserted ); echo " <br /> After inserting '$' the array is :<br />"; foreach ($original as $x) {echo "$x ";} ?>
8. Write a PHP script to sort the following associative array : Go to the editor
array("Sophia"=>"31","Jacob"=>"41","William"=>"39","Ramesh"=>"40") in
a) ascending order sort by value
b) ascending order sort by Key
c) descending order sorting by Value
d) descending order sorting by Key Go to the editor
<?php echo "
Associative array : Ascending order sort by value
"; $array2=array("Sophia"=>"31","Jacob"=>"41","William"=>"39","Ramesh"=>"40"); asort($array2); foreach($array2 as $y=>$y_value) { echo "Age of ".$y." is : ".$y_value."
"; } echo "
Associative array : Ascending order sort by Key
"; $array3=array("Sophia"=>"31","Jacob"=>"41","William"=>"39","Ramesh"=>"40"); ksort($array3); foreach($array3 as $y=>$y_value) { echo "Age of ".$y." is : ".$y_value."
"; } echo "
Associative array : Descending order sorting by Value
"; $age=array("Sophia"=>"31","Jacob"=>"41","William"=>"39","Ramesh"=>"40"); arsort($age); foreach($age as $y=>$y_value) { echo "Age of ".$y." is : ".$y_value."
"; } echo "
Associative array : Descending order sorting by Key
"; $array4=array("Sophia"=>"31","Jacob"=>"41","William"=>"39","Ramesh"=>"40"); krsort($array4); foreach($array4 as $y=>$y_value){ echo "Age of ".$y." is : ".$y_value."
"; } ?>
9. Write a PHP script to calculate and display average temperature, seven lowest and highest temperatures. Go to the editor
Recorded temperatures : 78, 60, 62, 68, 71, 68, 73, 85, 66, 64, 76, 63, 75, 76, 73,
68, 62, 73, 72, 65, 74, 62, 62, 65, 64, 68, 73, 75, 79, 73
Expected Output :
Average Temperature is : 70.6
List of seven lowest temperatures : 60, 62, 63, 63, 64,
List of seven highest temperatures : 76, 78, 79, 81, 85,
<?php $month_temp = "78, 60, 62, 68, 71, 68, 73, 85, 66, 64, 76, 63, 81, 76, 73, 68, 72, 73, 75, 65, 74, 63, 67, 65, 64, 68, 73, 75, 79, 73"; $temp_array = explode(',', $month_temp); $tot_temp = 0; $temp_array_length = count($temp_array); foreach($temp_array as $temp) { $tot_temp += $temp; } $avg_high_temp = $tot_temp/$temp_array_length; echo "Average Temperature is : ".$avg_high_temp."
"; sort($temp_array); echo "<br> List of seven lowest temperatures : "; for ($i=0; $i<5; $i++) { echo $temp_array[$i].", "; } echo "<br>List of seven highest temperatures : "; for ($i=($temp_array_length-5); $i<($temp_array_length); $i++) { echo $temp_array[$i].", "; } ?>
10. Write a PHP program to sort an array of positive integers using the Bead Sort Algorithm. Go to the editor
According to Wikipedia "Bead sort is a natural sorting algorithm, developed by Joshua J. Arulanandham, Cristian S. Calude and Michael J. Dinneen in 2002. Both digital and analog hardware implementations of bead sort can achieve a sorting time of O(n); however, the implementation of this algorithm tends to be significantly slower in software and can only be used to sort lists of positive integers".
Input array : Array ( [0] => 5 [1] => 3 [2] => 1 [3] => 3 [4] => 8 [5] => 7 [6] => 4 [7] => 1 [8] => 1 [9] => 3 )
Expected Result : Array ( [0] => 8 [1] => 7 [2] => 5 [3] => 4 [4] => 3 [5] => 3 [6] => 3 [7] => 1 [8] => 1 [9] => 1 )
<?php function columns($uarr) { $n=$uarr; if (count($n) == 0) return array(); else if (count($n) == 1) return array_chunk($n[0], 1); array_unshift($uarr, NULL); $transpose = call_user_func_array('array_map', $uarr); return array_map('array_filter', $transpose); } function bead_sort($uarr) { foreach ($uarr as $e) $poles []= array_fill(0, $e, 1); return array_map('count', columns(columns($poles))); } echo 'Original Array : '.'
'; print_r(array(5,3,1,3,8,7,4,1,1,3)); echo '
'.'After Bead sort : '.'
'; print_r(bead_sort(array(5,3,1,3,8,7,4,1,1,3))); ?>
11. Write a PHP program to merge (by index) the following two arrays. Go to the editor
Sample arrays :
$array1 = array(array(77, 87), array(23, 45));
$array2 = array("w3resource", "com");
Expected Output :
Array ( [0] => Array ( [0] => w3resource [1] => 77 [2] => 87 ) [1] => Array ( [0] => com [1] => 23 [2] => 45 ) )
<?php $array1 = array(array(77, 87), array(23, 45)); $array2 = array("w3resource", "com"); function merge_arrays_by_index($x, $y) { $temp = array(); $temp[] = $x; if(is_scalar($y)) { $temp[] = $y; } else { foreach($y as $k => $v) { $temp[] = $v; } } return $temp; } echo '<pre>'; print_r(array_map('merge_arrays_by_index',$array2, $array1)); ?>
12. Write a PHP function to change the following array's all values to upper or lower case. Go to the editor
Sample arrays :
$Color = array('A' => 'Blue', 'B' => 'Green', 'c' => 'Red');
Expected Output :
Values are in lower case.
Array ( [A] => blue [B] => green [c] => red )
Values are in upper case.
Array ( [A] => BLUE [B] => GREEN [c] => RED )
<?php function array_change_value_case($input, $ucase) { $case = $ucase; $narray = array(); if (!is_array($input)) { return $narray; } foreach ($input as $key => $value) { if (is_array($value)) { $narray[$key] = array_change_value_case($value, $case); continue; } $narray[$key] = ($case == CASE_UPPER ? strtoupper($value) : strtolower($value)); } return $narray; } $Color = array('A' => 'Blue', 'B' => 'Green', 'c' => 'Red'); echo 'Actual array </br>'; print_r($Color); echo '</br>Values are in lower case.</br>'; $myColor = array_change_value_case($Color,CASE_LOWER); print_r($myColor); echo '</br>Values are in upper case.</br>'; $myColor = array_change_value_case($Color,CASE_UPPER); print_r($myColor); ?>
13. Write a PHP script which display all the numbers between 200 and 250 that are divisible by 4. Go to the editor
Note : Do not use any PHP control statement.
Expected Output : 200,204,208,212,216,220,224,228,232,236,240,244,248
<?php echo implode(",",range(200,250,4)); ?>
14. Write a PHP script to get the shortest/longest string length from an array. Go to the editor
Sample arrays : ("abcd","abc","de","hjjj","g","wer")
Expected Output : The shortest array length is 1. The longest array length is 4.
<?php $my_array = array("abcd","abc","de","hjjj","g","wer"); $new_array = array_map('strlen', $my_array); // Show maximum and minimum string length using max() function and min() function echo "The shortest array length is " . min($new_array) . ". The longest array length is " . max($new_array).'.'; ?>
15. Write a PHP script to generate unique random numbers within a range. Go to the editor
Sample Range : (11, 20)
Sample Output : 17 16 13 20 14 19 18 15 11 12
<?php $n=range(11,20); shuffle($n); for ($x=0; $x<10; $x++) { echo $n[$x].' '; } ?>
More to Come !