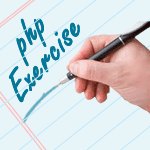
[An editor is available at the bottom of the page to write and execute the scripts. Go to the editor]
1. Write a PHP script to get the PHP version and configuration information.
<?php phpinfo(); ?>
2. Write a PHP script to display the following strings.
Sample String :
'Tomorrow I \'ll learn PHP global variables.'
'This is a bad command : del c:\\*.*'
Expected Output :
Tomorrow I 'll learn PHP global variables.
This is a bad command : del c:\*.*
<?php echo 'Tomorrow I \'ll learn PHP global variables.'; echo 'This is a bad command : del c:\\*.*'; ?>
3. $var = 'PHP Tutorial'. Put this variable into the title section, h3 tag and as an anchor text within a HTML document.
Sample Output :
PHP, an acronym for Hypertext Preprocessor, is a widely-used open source general-purpose scripting language. It is a cross-platform, HTML embedded server-side scripting language and is especially suited for web development.
<?php $var = 'PHP Tutorial'; ?> <!DOCTYPE html> <html> <head> <meta http-equiv="content-type" content="text/html; charset=utf-8"> <title><?php echo $var; ?> - W3resource!</title> </head> <body> <h3><?php echo $var; ?></h3> <p>PHP, an acronym for Hypertext Preprocessor, is a widely-used open source general-purpose scripting language. It is a cross-platform, HTML embedded server-side scripting language and is especially suited for web development.</p> <p><a href="https://www.w3resource.com/php/php-home.php">Go to the <?php echo $var; ?></a>.</p> </body> </html>
4. Create a simple HTML form and accept the user name and display the name through PHP echo statement.
Sample output of the HTML form :
<!DOCTYPE html> <html> <head> <title></title> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> </head> <body> <form method='POST'> <h2>Please input your name:</h2> <input type="text" name="name"> <input type="submit" value="Submit Name"> </form> <?php //Retrieve name from query string and store to a local variable $name = $_POST['name']; echo "<h3> Hello $name </h3>"; ?> </body> </html>
5. Write a PHP script to get the client IP address.
<?php //whether ip is from share internet if (!empty($_SERVER['HTTP_CLIENT_IP'])) { $ip_address = $_SERVER['HTTP_CLIENT_IP']; } //whether ip is from proxy elseif (!empty($_SERVER['HTTP_X_FORWARDED_FOR'])) { $ip_address = $_SERVER['HTTP_X_FORWARDED_FOR']; } //whether ip is from remote address else { $ip_address = $_SERVER['REMOTE_ADDR']; } echo $ip_address; ?>
6. Write a simple PHP browser detection script.
Sample Output : Your User Agent is :Mozilla/5.0 (Windows NT 6.1) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/35.0.1916.114 Safari/537.36
<?php echo "Your User Agent is :" . $_SERVER ['HTTP_USER_AGENT']; ?>
7. Write a PHP script to get the current file name.
<?php $current_file_name = basename($_SERVER['PHP_SELF']); echo $current_file_name; ?>
8. Write a PHP script, which will return the following components of the url 'https://www.w3resource.com/php-exercises/php-basic-exercises.php'.
List of components : Scheme, Host, Path
Expected Output :
Scheme : http
Host : www.w3resource.com
Path : /php-exercises/php-basic-exercises.php
<?php $url = 'https://www.w3resource.com/php-exercises/php-basic-exercises.php'; $url=parse_url($url); echo 'Scheme : '.$url['scheme'].'<br />'; echo 'Host : '.$url['host'].'<br />'; echo 'Path : '.$url['path'].'<br />'; ?>
9. Write a PHP script, which change the color of first character of a word.
Sample string : PHP Tutorial
Expected Output :
PHP Tutorial
<?php $text = 'PHP Tutorial'; $text = preg_replace('/(\b[a-z])/i','<span style="color:red;">\1</span>',$text); echo $text; ?>
10. Write a PHP script, to check whether the page is called from 'https' or 'http'.
<?php if (!empty($_SERVER['HTTPS'])) { echo 'https is enabled'; } else { echo 'http is enabled'; } ?>
11. Write a PHP script to redirect a user to a different page .
Expected output : Redirect the user to https://www.w3resource.com/
<?php header('Location: https://www.w3resource.com/'); ?>
12. Write a simple PHP program to check that emails are valid.
Hints : Use FILTER_VALIDATE_EMAIL filter that validates value as an e-mail address.
Note : The PHP documentation does not saying that FILTER_VALIDATE_EMAIL should pass the RFC5321.
<?php // pass valid/invalid emails $email = "[email protected]"; if (filter_var($email, FILTER_VALIDATE_EMAIL)) { echo '"' . $email . '" = Valid'; } else { echo '"' . $email . '" = Invalid'; } ?>
13. Write a e PHP script to display string, values within a table.
Note : Use HTML table elements into echo.
Expected Output :
<?php $a=1000; $b=1200; $c=1400; echo "<table border=1 cellspacing=0 cellpading=0> <tr> <td><font color=blue>Salary of Mr. A is</td> <td>$a$</font></td></tr> <tr> <td><font color=blue>Salary of Mr. B is</td> <td>$b$</font></td></tr> <tr> <td><font color=blue>Salary of Mr. C is</td> <td>$c$</font></td></tr> </table>"; ?>
14. Write a e PHP script to display source code of a webpage (e.g. "http://www.example.com/").
<?php $all_lines = file('http://www.example.com/'); foreach ($all_lines as $line_num => $line) { echo "Line No.-{$line_num}: " . htmlspecialchars($line) . "<br />\n"; } ?>
15. Write a PHP script to get last modified information of a file.
Sample filename : php-basic-exercises.php
Sample Output : Last modified Monday, 09th June, 2014, 06:45am
<?php $file_last_modified = filemtime("php-basic-exercises.php"); echo "Last modified " . date("l, dS F, Y, h:ia", $file_last_modified); ?>
16. Write a PHP script to count lines in a file.
Note : Store a text file name into a variable and count the number of lines of text it has.
<?php $file = "abc.php"; $no_of_lines = count(file($file)); echo "There are $no_of_lines lines in $file"; ?>
Write your code here :
Next :
More to Come !