Java compareTo() Method
public int compareTo(Date dt)
The compareTo() method is used to compare two dates for ordering.
Package: java.util
Java Platform: Java SE 8
Syntax:
compareTo(Date dt)
Parameters:
Name | Description |
---|---|
dt | The date to be compared. |
Return Value:
- The return value will be 0 if two dates are equal.
- The return value will be less than 0 if one date is before another date.
- The return value will be greater than 0 if one date is after another date.
Return Value Type: int
Throws:
NullPointerException - if anotherDate is null.
Pictorial Presentation of Jave Date compareto() method:
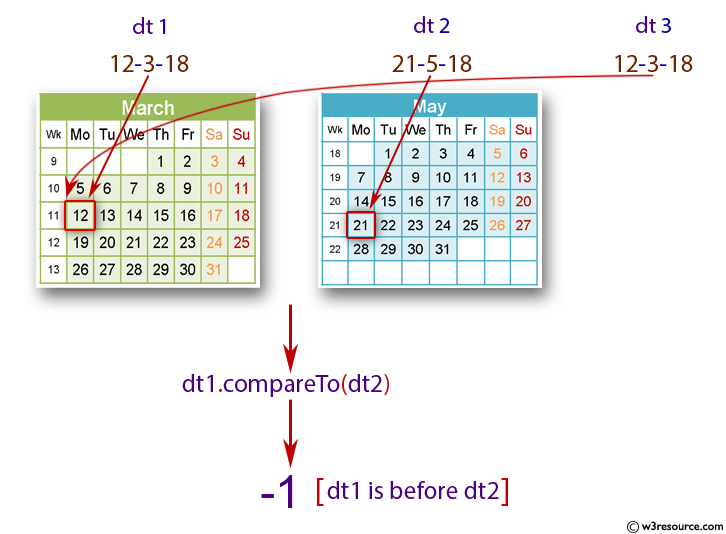
Example: Java Date.compareTo(Date anotherDate) Method
import java.util.Date;
public class Main {
public static void main(String[] args) {
Date dt1 = new Date(2018, 3, 12);
Date dt2 = new Date(2018, 5, 21);
Date dt3 = new Date(2018, 3, 12);
int output = dt1.compareTo(dt2);
System.out.println("If date1 is after date2: " + output);
output = dt2.compareTo(dt3);
System.out.println("If date2 is before date3: " + output);
output = dt1.compareTo(dt3);
System.out.println("If date1 equal to date3: " + output);
}
}
Output:
If date1 is after date2: -1 If date2 is before date3: 1 If date1 equal to date3: 0
Java Code Editor:
Previous:clone Method
Next:equals Method