C#: Combine two strings. If the given strings have different length remove excess characters from the beginning of the longer string
Combine Strings After Adjusting Lengths
Write a C# Sharp program to combine two given strings. If the given strings have different lengths remove the characters from the longer string.
Visual Presentation:
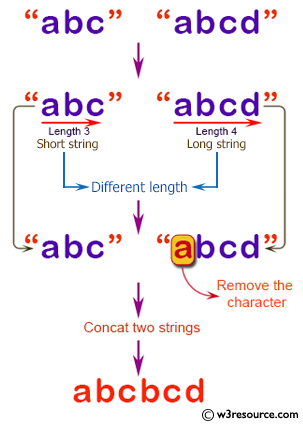
Sample Solution:-
C# Sharp Code:
using System; // Importing the System namespace
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Output the results of the test function with different strings as arguments
Console.WriteLine(test("abc", "abcd")); // Pass "abc" and "abcd" to the test function and display the result
Console.WriteLine(test("Python", "Python")); // Pass "Python" twice to the test function and display the result
Console.WriteLine(test("JS", "Python")); // Pass "JS" and "Python" to the test function and display the result
Console.ReadLine(); // Wait for user input before closing the console window
}
// Function definition of the test function that takes two strings 's1' and 's2' as input and returns a string
public static string test(string s1, string s2)
{
if (s1.Length < s2.Length) // If the length of 's1' is less than the length of 's2'
{
// Concatenate 's1' with the end portion of 's2' to match the length of 's2'
return s1 + s2.Substring(s2.Length - s1.Length);
}
else if (s1.Length > s2.Length) // If the length of 's1' is greater than the length of 's2'
{
// Concatenate the end portion of 's1' with 's2' to match the length of 's1'
return s1.Substring(s1.Length - s2.Length) + s2;
}
else // If the lengths of 's1' and 's2' are equal
{
// Concatenate 's1' and 's2'
return s1 + s2;
}
}
}
}
Sample Output:
abcbcd PythonPython JSon
Flowchart:
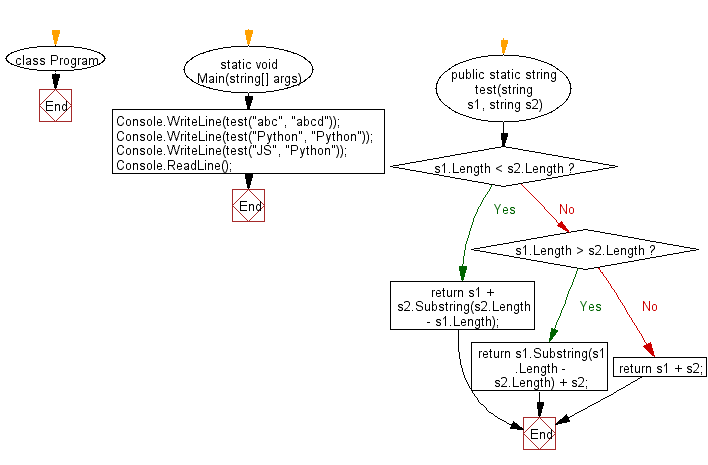
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to check whether the first two characters and last two characters of a given string are same.
Next: Write a C# Sharp program to create a new string using 3 copies of the first 2 characters of a given string. If the length of the given string is less than 2 use the whole string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics