C#: Check whether y is greater than x, and z is greater than y from three given integers x,y,z
Strict Increasing Order for Three Numbers
Write a C# Sharp program to check whether y is greater than x, and z is greater than y from three given integers x,y,z.
Visual Presentation:
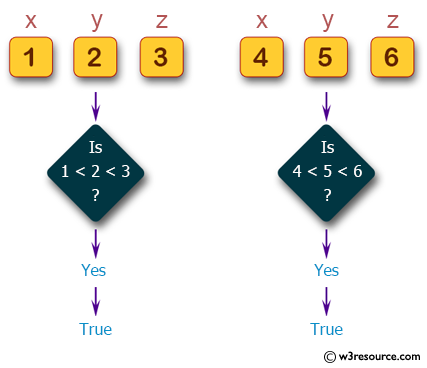
Sample Solution:-
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
// Class declaration
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Calling the 'test' method with different integer values and printing the results
Console.WriteLine(test(1, 2, 3)); // Output: True
Console.WriteLine(test(4, 5, 6)); // Output: True
Console.WriteLine(test(-1, 1, 0)); // Output: False
Console.ReadLine(); // Keeping the console window open
}
// Method to check if the integers x, y, z are in ascending order
public static bool test(int x, int y, int z)
{
// Returns true if x is less than y and y is less than z
return x < y && y < z;
}
}
}
Sample Output:
True True False
Flowchart:
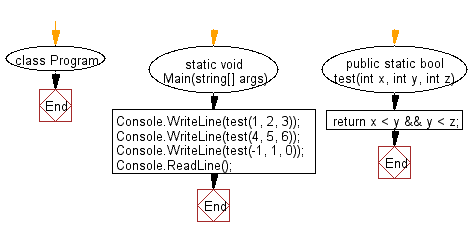
For more Practice: Solve these Related Problems:
- Write a C# program to verify if three integers are in strictly decreasing order.
- Write a C# program to return true if each next number is double the previous number.
- Write a C# program to return true if three given numbers form an arithmetic sequence.
- Write a C# program to check if three integers form a geometric progression.
Go to:
PREV : Sum of Two Equals Third.
NEXT : Strict Increasing Order for Three Numbers.
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.