C#: Check whether a given non-negative given number is a multiple of 3 or 7, but not both
Multiple of 3 or 7 but Not Both
Write a C# Sharp program to check if a given number that is not negative is a multiple of 3 or 7, but not both.
Visual Presentation:
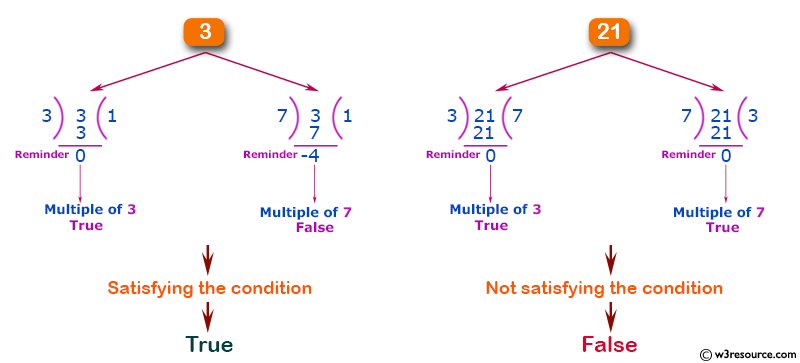
Sample Solution:-
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
// Class declaration
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Calling the 'test' method with different integers and printing the results
Console.WriteLine(test(3)); // Output: True
Console.WriteLine(test(7)); // Output: True
Console.WriteLine(test(21)); // Output: False
Console.ReadLine(); // Keeping the console window open
}
// Method to check if a number is divisible by either 3 or 7 exclusively
public static bool test(int n)
{
// Return the result of the exclusive OR (^) operation between the conditions
// Returns true if either n is divisible by 3 or n is divisible by 7, but not both
return n % 3 == 0 ^ n % 7 == 0;
}
}
}
Sample Output:
True True False
Flowchart:
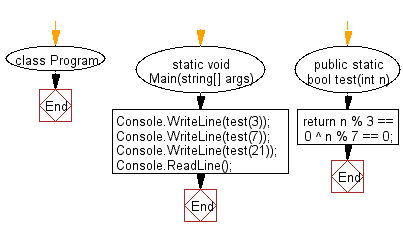
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program test if a given non-negative number is a multiple of 13 or it is one more than a multiple of 13.
Next: Write a C# Sharp program to check if a given number is within 2 of a multiple of 10.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics