C#: Test whether a given non-negative number is a multiple of 13 or it is one more than a multiple of 13
Multiple of 13 or 1 More
Write a C# Sharp program to test if a given non-negative number is a multiple of 13 or one more than a multiple of 13.
Sample Solution:-
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
// Class declaration
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Calling the 'test' method with different integers and printing the results
Console.WriteLine(test(13)); // Output: True
Console.WriteLine(test(14)); // Output: True
Console.WriteLine(test(27)); // Output: False
Console.WriteLine(test(41)); // Output: True
Console.ReadLine(); // Keeping the console window open
}
// Method to check if a number is either a multiple of 13 or has a remainder of 1 when divided by 13
public static bool test(int n)
{
// Check if the remainder when 'n' is divided by 13 is either 0 or 1
return n % 13 == 0 || n % 13 == 1;
}
}
}
Sample Output:
True True True False
Flowchart:
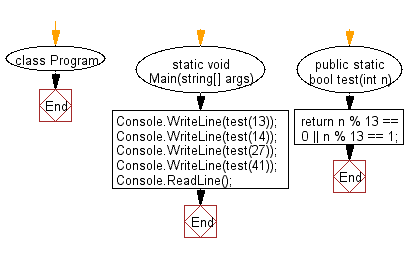
For more Practice: Solve these Related Problems:
- Write a C# program to check if a number is one less than a multiple of 13 or exactly divisible by 13.
- Write a C# program to return true if a number is either a multiple of 13 or a multiple of 7 plus 6.
- Write a C# program to determine whether a number is within 1 of a multiple of 13 or 26.
- Write a C# program to test if a number is divisible by 13 or contains the digit '3'.
Go to:
PREV : Check for 5 or Sum/Difference Equals 5.
NEXT : Multiple of 3 or 7 but Not Both.
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.