C#: Compare two given strings and return the number of the positions where they contain the same substring of length 2
Count Matching Substrings in Two Strings
Write a C# Sharp program to compare two given strings and return the number of positions where they contain the same length of 2 substrings.
Sample Solution:-
C# Sharp Code:
using System;
using System.Linq;
// Namespace declaration
namespace exercises
{
// Class declaration
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Calling the 'test' method with different pairs of strings
Console.WriteLine(test("abcdefgh", "abijsklm")); // Output: 3
Console.WriteLine(test("abcde", "osuefrcd")); // Output: 1
Console.WriteLine(test("pqrstuvwx", "pqkdiewx"));// Output: 2
Console.ReadLine(); // Keeping the console window open
}
// Method to count occurrences of two-character substrings that are common in both input strings
public static int test(string str1, string str2)
{
var ctr = 0; // Counter to track the occurrences of common two-character substrings
// Iterate through the first string's characters except the last one
for (var i = 0; i < str1.Length - 1; i++)
{
var firstString = str1.Substring(i, 2); // Extract a two-character substring from the first string
// Iterate through the second string's characters except the last one
for (var j = 0; j < str2.Length - 1; j++)
{
var secondString = str2.Substring(j, 2); // Extract a two-character substring from the second string
// Check if the extracted substrings from both strings are equal
if (firstString.Equals(secondString))
ctr++; // Increment the counter if the substrings are equal
}
}
return ctr; // Return the total count of common two-character substrings
}
}
}
Sample Output:
1 1 2
Flowchart:
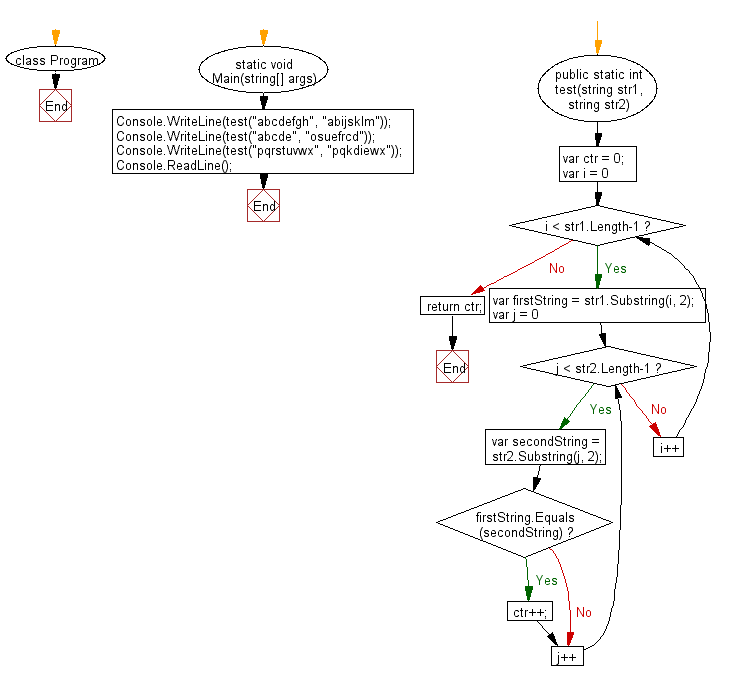
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to check whether the sequence of numbers 1, 2, 3 appears in a given array of integers somewhere.
Next: Write a C# Sharp program to create a new string from a give string where a specified character have been removed except starting and ending position of the given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics