C#: Check whether the sequence of numbers 1, 2, 3 appears in a given array of integers somewhere
Check Sequence 1, 2, 3 in Array
Write a C# Sharp program to check whether the sequence of numbers 1, 2, 3 appears in a given array of integers somewhere.
Visual Presentation:
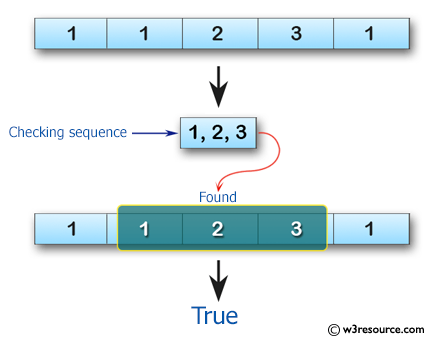
Sample Solution:-
C# Sharp Code:
using System;
using System.Linq;
// Namespace declaration
namespace exercises
{
// Class declaration
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Calling the 'test' method with different integer arrays
Console.WriteLine(test(new[] {1,1,2,3,1})); // Output: True
Console.WriteLine(test(new[] {1,1,2,4,1})); // Output: False
Console.WriteLine(test(new[] {1,1,2,1,2,3})); // Output: True
Console.ReadLine(); // Keeping the console window open
}
// Method to check if an array contains the sequence 1, 2, 3 in consecutive positions
public static bool test(int[] nums)
{
// Loop through the array elements except the last two
for (var i = 0; i < nums.Length-2; i++)
{
// Check if the current element and the next two elements form the sequence 1, 2, 3
if (nums[i] == 1 && nums[i + 1] == 2 && nums[i + 2] == 3)
return true; // Return true if the sequence is found
}
return false; // Return false if the sequence is not found
}
}
}
Sample Output:
True False True
Flowchart:
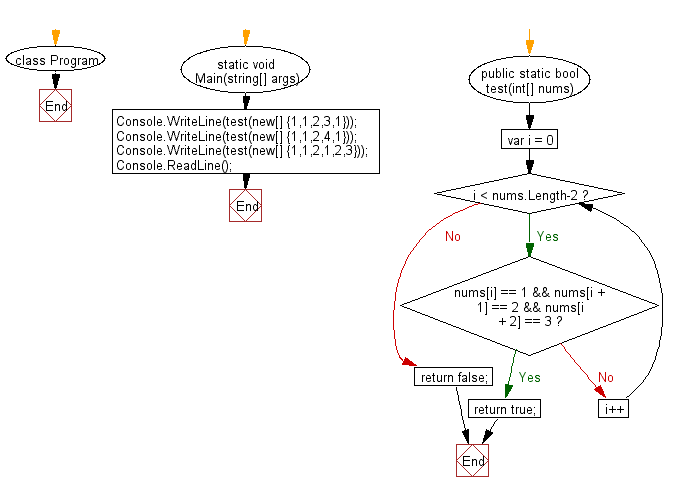
For more Practice: Solve these Related Problems:
- Write a C# program to detect any ascending sequence of 3 consecutive integers in a given array.
- Write a C# program to check if the sequence 1,2,3 exists in reverse order anywhere in the array.
- Write a C# program to determine if multiple non-overlapping sequences of 1,2,3 exist in an array.
- Write a C# program to check if the sequence 1,2,3 appears starting from an even index.
Go to:
PREV : Element in First 4 Positions of Array.
NEXT : Count Matching Substrings in Two Strings.
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.