C#: Check whether two given non-negative integers have the same last digit
Same Last Digit for Two Integers
Write a C# Sharp program to check whether two given non-negative integers have the same last digit.
Visual Presentation:
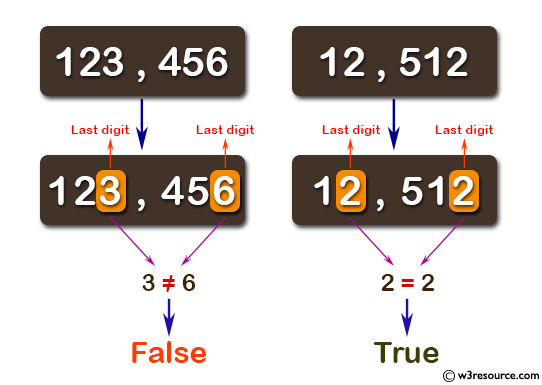
Sample Solution:-
C# Sharp Code:
using System;
// Namespace declaration
namespace exercises
{
// Class declaration
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Displaying the output of the 'test' method with different integer inputs
Console.WriteLine(test(123, 456)); // Output: False
Console.WriteLine(test(12, 512)); // Output: True
Console.WriteLine(test(7, 87)); // Output: True
Console.WriteLine(test(12, 45)); // Output: False
Console.ReadLine(); // Keeping the console window open
}
// Method to check if the last digits of two integers are equal in absolute value
public static bool test(int x, int y)
{
// Using Math.Abs to get the absolute value of the last digit of each integer and comparing them
// Returns true if the absolute value of the last digits of x and y are equal, otherwise returns false
return Math.Abs(x % 10) == Math.Abs(y % 10);
}
}
}
Sample Output:
False True True False
Flowchart:
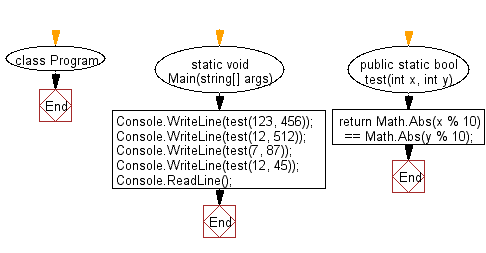
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to check if a given string contains between 2 and 4 'z' character.
Next: Write a C# Sharp program to convert the last 3 characters of a given string in upper case.If the length of the string has less than 3 then uppercase all the characters.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics